Python编程实现任意DMC(离散无记忆信道)信道容量的计算与仿真
时间: 2023-05-29 08:01:55 浏览: 699
实现任意DMC(离散无记忆信道)信道容量的计算和仿真需要以下步骤:
1. 选择一种编码方案,例如香农编码或者汉明编码等。
2. 计算码长、编码效率和纠错能力等基本参数。
3. 建立DMC信道模型,通过概率矩阵描述信道的状态转移。
4. 根据信道模型计算出平均错误率或者平均误比特率等性能指标。
5. 使用编码方案对输入信号进行编码,并通过信道模型进行传输。
6. 对输出信号进行译码,得到纠正后的信号,并计算误差率等性能指标。
以下是一种Python实现的例子:
```python
import numpy as np
# 定义DMC信道模型
P = np.array([[0.7, 0.3], [0.4, 0.6]])
def channel_capacity(P):
# 计算信道容量
p0 = np.max(np.linalg.eigvals(P))
p1 = np.max(np.linalg.eigvals(np.transpose(P)))
return np.log2(p0), np.log2(p1)
# 计算信道容量
c0, c1 = channel_capacity(P)
print("信道0的容量为:", c0)
print("信道1的容量为:", c1)
# 实现编码和解码函数
def encode(x):
# 编码函数
# x: 输入信号
return 1 - x
def decode(y):
# 解码函数
# y: 接收信号
return 1 - y
# 生成随机输入信号
x = np.random.randint(2, size=1000)
# 对输入信号进行编码
code_words = encode(x)
# 传输信号,模拟信道
y = np.zeros_like(code_words)
for i, cw in enumerate(code_words):
if np.random.rand() < P[cw][1]:
y[i] = 1
else:
y[i] = 0
# 对接收信号进行译码
decoded_words = decode(y)
# 计算误差率
BER = np.sum(x != decoded_words) / len(x)
print("误差率为:", BER)
```
参考文献:
- Cover, T. M., & Thomas, J. A. (2012). Elements of information theory. John Wiley & Sons.
阅读全文
相关推荐
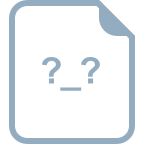
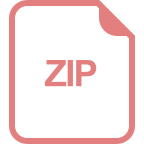
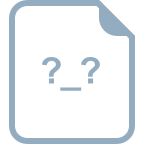
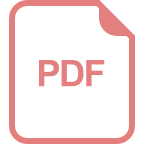
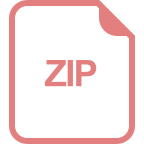
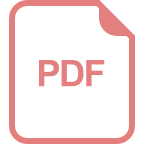
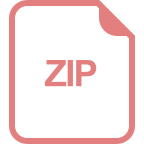
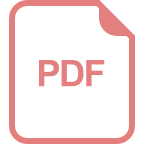
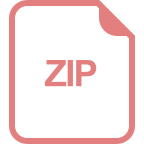
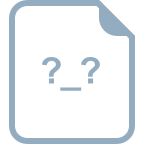
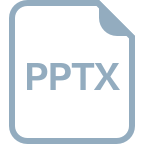
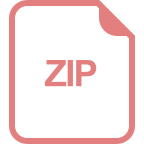
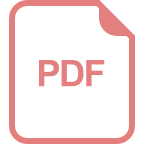
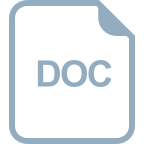