宠物管理系统 商品管理页面 商品评价管理、商品收藏管理、商品订单管理技术实现 代码示例
时间: 2023-12-02 17:03:16 浏览: 37
以下是一个基于Java Spring框架的宠物管理系统商品管理页面商品评价管理、商品收藏管理、商品订单管理的代码示例:
商品评价管理:
```
@Controller
@RequestMapping("/comment")
public class CommentController {
@Autowired
private CommentService commentService;
@GetMapping("/{id}")
public String getComment(@PathVariable("id") Long id, Model model) {
List<Comment> comments = commentService.getCommentsByProductId(id);
model.addAttribute("comments", comments);
return "comment";
}
@PostMapping("/")
public String addComment(@RequestParam("productId") Long productId,
@RequestParam("content") String content,
@RequestParam("userId") Long userId) {
Comment comment = new Comment();
comment.setProductId(productId);
comment.setUserId(userId);
comment.setContent(content);
commentService.addComment(comment);
return "redirect:/comment/" + productId;
}
}
```
商品收藏管理:
```
@Controller
@RequestMapping("/favorite")
public class FavoriteController {
@Autowired
private FavoriteService favoriteService;
@GetMapping("/{userId}")
public String getFavorites(@PathVariable("userId") Long userId, Model model) {
List<Favorite> favorites = favoriteService.getFavoritesByUserId(userId);
model.addAttribute("favorites", favorites);
return "favorite";
}
@PostMapping("/")
public String addFavorite(@RequestParam("productId") Long productId,
@RequestParam("userId") Long userId) {
Favorite favorite = new Favorite();
favorite.setProductId(productId);
favorite.setUserId(userId);
favoriteService.addFavorite(favorite);
return "redirect:/favorite/" + userId;
}
@DeleteMapping("/{id}")
public String deleteFavorite(@PathVariable("id") Long id,
@RequestParam("userId") Long userId) {
favoriteService.deleteFavoriteById(id);
return "redirect:/favorite/" + userId;
}
}
```
商品订单管理:
```
@Controller
@RequestMapping("/order")
public class OrderController {
@Autowired
private OrderService orderService;
@GetMapping("/{userId}")
public String getOrders(@PathVariable("userId") Long userId, Model model) {
List<Order> orders = orderService.getOrdersByUserId(userId);
model.addAttribute("orders", orders);
return "order";
}
@PostMapping("/")
public String addOrder(@RequestParam("productId") Long productId,
@RequestParam("userId") Long userId) {
Order order = new Order();
order.setProductId(productId);
order.setUserId(userId);
orderService.addOrder(order);
return "redirect:/order/" + userId;
}
@DeleteMapping("/{id}")
public String deleteOrder(@PathVariable("id") Long id,
@RequestParam("userId") Long userId) {
orderService.deleteOrderById(id);
return "redirect:/order/" + userId;
}
}
```
相关推荐
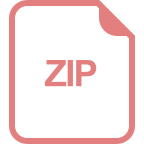
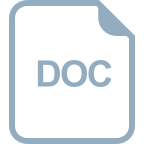
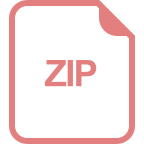














