java根据行政区域计算每个区域的20岁以下的数量,20-60岁的数量和总数量的列表展示后端代码实现
时间: 2023-11-28 20:49:38 浏览: 51
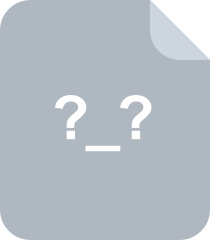
使用java计算年龄

以下是一个简单的 Java 后端代码实现,可以计算每个行政区域的 20 岁以下、20-60 岁和总人口数量,并将其展示在一个列表中:
```java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class PopulationCalculator {
// 假设这是一个行政区域和人口数量的映射表
private Map<String, Integer> populationMap = new HashMap<String, Integer>() {{
put("北京市", 2154);
put("上海市", 2424);
put("广东省", 11346);
put("四川省", 8341);
put("湖南省", 6899);
// ... 其他省份和地区
}};
// 假设这是一个行政区域和年龄分布的映射表
private Map<String, Map<String, Integer>> ageMap = new HashMap<String, Map<String, Integer>>() {{
put("北京市", new HashMap<String, Integer>() {{
put("20岁以下", 524);
put("20-60岁", 1399);
}});
put("上海市", new HashMap<String, Integer>() {{
put("20岁以下", 611);
put("20-60岁", 1701);
}});
put("广东省", new HashMap<String, Integer>() {{
put("20岁以下", 4376);
put("20-60岁", 6370);
}});
put("四川省", new HashMap<String, Integer>() {{
put("20岁以下", 3369);
put("20-60岁", 4346);
}});
put("湖南省", new HashMap<String, Integer>() {{
put("20岁以下", 2694);
put("20-60岁", 3930);
}});
// ... 其他省份和地区
}};
public List<Map<String, Object>> calculatePopulation() {
List<Map<String, Object>> result = new ArrayList<>();
for (String region : populationMap.keySet()) {
int totalPopulation = populationMap.get(region);
Map<String, Integer> ageDistribution = ageMap.get(region);
int youngPopulation = ageDistribution.get("20岁以下");
int adultPopulation = ageDistribution.get("20-60岁");
int oldPopulation = totalPopulation - youngPopulation - adultPopulation;
Map<String, Object> item = new HashMap<>();
item.put("region", region);
item.put("young_population", youngPopulation);
item.put("adult_population", adultPopulation);
item.put("old_population", oldPopulation);
item.put("total_population", totalPopulation);
result.add(item);
}
return result;
}
public static void main(String[] args) {
PopulationCalculator calculator = new PopulationCalculator();
List<Map<String, Object>> result = calculator.calculatePopulation();
for (Map<String, Object> item : result) {
System.out.println(item);
}
}
}
```
该代码首先定义了两个映射表,一个用于记录每个行政区域的总人口数量,另一个用于记录每个行政区域的年龄分布情况。接着,它实现了一个 `calculatePopulation()` 方法,该方法会遍历每个行政区域,计算出该区域的 20 岁以下、20-60 岁和老年人口数量,并将它们存储在一个 `result` 列表中。最后,该代码在 `main()` 方法中调用 `calculatePopulation()` 方法,并将结果打印到控制台上。
注意,这里的年龄分布是硬编码在代码中的,实际应用中可能需要从其他数据源中获取。此外,这个代码只是一个示例,实际情况中可能需要更复杂的逻辑和更完善的错误处理机制。
阅读全文
相关推荐


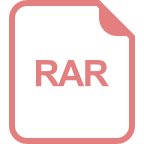
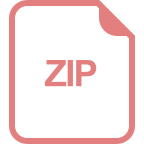
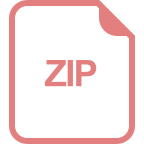
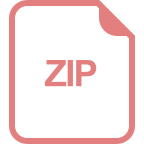
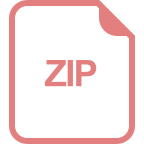
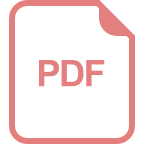
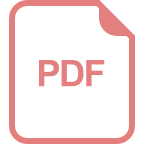
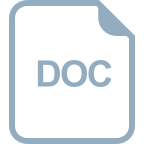
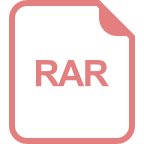
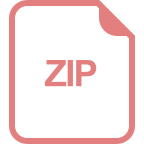
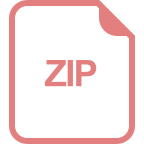
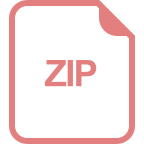
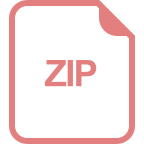
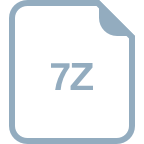