strstr函数的用法
时间: 2023-08-30 15:06:59 浏览: 53
strstr函数是C语言中的一个字符串函数,用于在一个字符串中查找另一个字符串的出现位置。它的函数原型如下:
```
char *strstr(const char *str1, const char *str2);
```
其中,str1是要查找的字符串,str2是要查找的子字符串。函数返回值是一个指向字符串str2第一次出现的位置的指针,如果没有找到则返回NULL。
例如,如果我们要在字符串str1中查找子字符串str2,可以这样使用strstr函数:
```
char *str1 = "Hello, world!";
char *str2 = "world";
char *result = strstr(str1, str2);
if (result == NULL) {
printf("Not found\n");
} else {
printf("Found at position: %ld\n", result - str1);
}
```
上述代码将在字符串str1中查找子字符串str2,并输出它的位置。如果找不到则输出"Not found"。需要注意的是,在使用strstr函数时应该确保str1和str2都是以'\0'结尾的字符串。
相关问题
strstr函数使用方法
strstr函数是C语言中的一个字符串处理函数,其作用是在一个字符串中查找另一个字符串第一次出现的位置。其函数原型为:
```
char *strstr(const char *str1, const char *str2);
```
其中,str1表示要被查找的字符串,str2表示要查找的子字符串。函数返回值为一个指向str2在str1中第一次出现位置的指针,如果str2未在str1中出现,则返回NULL。
使用该函数时,需要注意以下几点:
1. 在使用该函数前,需要确保str1和str2指向的字符串以'\0'结尾,否则可能会导致结果不正确。
2. 在使用该函数时,应该判断函数的返回值是否为NULL,以确保查找到了需要的子字符串。
下面是一个使用strstr函数的例子:
```
#include <stdio.h>
#include <string.h>
int main()
{
char str1[] = "hello world";
char str2[] = "world";
char *p = NULL;
p = strstr(str1, str2);
if (p != NULL)
{
printf("'%s' is found in '%s' at position %ld.\n", str2, str1, p - str1);
}
else
{
printf("'%s' is not found in '%s'.\n", str2, str1);
}
return 0;
}
```
运行结果为:"'world' is found in 'hello world' at position 6."
strstr函数用法举例
strstr函数是C语言中的字符串处理函数,用于在一个字符串中查找指定子串的位置。它的函数原型如下:
```c
char *strstr(const char *haystack, const char *needle);
```
其中,`haystack`是要搜索的字符串,`needle`是要查找的子串。函数返回子串在字符串中第一次出现的位置,如果找不到则返回NULL。
下面是一个使用strstr函数的例子:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, world!";
char *result = strstr(str, "world");
if (result != NULL) {
printf("子串在字符串中的位置:%ld\n", result - str);
} else {
printf("未找到子串\n");
}
return 0;
}
```
输出结果为:
```
子串在字符串中的位置:7
```
这个例子中,我们在字符串"Hello, world!"中查找子串"world",并输出子串在字符串中的位置。由于子串在字符串中的起始位置是从0开始计数的,所以输出结果为7。
相关推荐
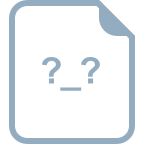
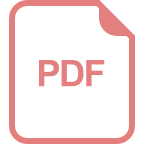



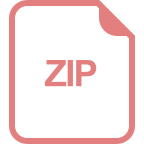
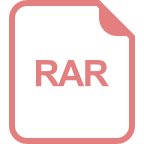
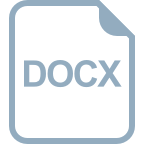
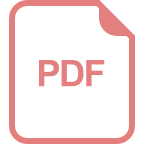
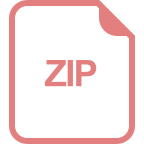