提供爬取微博相关数据的Python程序
时间: 2024-03-28 11:18:29 浏览: 100
以下是使用Python爬取微博相关数据的示例程序,你需要安装selenium、beautifulsoup和pandas库:
```python
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
from bs4 import BeautifulSoup
import pandas as pd
import time
# 设置webdriver的Chrome选项
options = webdriver.ChromeOptions()
options.add_argument('--headless') # 无头模式
options.add_argument('--disable-gpu') # 禁用GPU加速
options.add_argument('lang=zh_CN.UTF-8') # 设置语言为中文
# 打开Chrome浏览器并加载微博页面
driver = webdriver.Chrome(options=options)
driver.get('https://weibo.com/')
# 输入微博账号和密码
username = driver.find_element_by_xpath('//*[@id="loginname"]')
password = driver.find_element_by_xpath('//*[@id="pl_login_form"]/div/div[3]/div[2]/div/input')
username.send_keys('your_username')
password.send_keys('your_password')
password.send_keys(Keys.RETURN)
# 等待页面加载完成
time.sleep(10)
# 输入要搜索的关键字
search_input = driver.find_element_by_xpath('//*[@id="plc_top"]/div/div/div[3]/div/form/input[4]')
search_input.send_keys('关键字')
search_input.send_keys(Keys.RETURN)
# 等待搜索结果页面加载完成
time.sleep(10)
# 定义一个空的DataFrame来存储搜索结果
data = pd.DataFrame(columns=['微博ID', '用户名', '发布时间', '内容', '转发数', '评论数', '点赞数'])
# 循环遍历搜索结果的每一页
for page in range(1, 11):
# 获取页面源代码并使用BeautifulSoup解析
html = driver.page_source
soup = BeautifulSoup(html, 'html.parser')
# 获取搜索结果列表
results = soup.find_all('div', {'class': 'WB_cardwrap WB_feed_type S_bg2 WB_feed_vipcover WB_feed_like'})
# 循环遍历搜索结果列表,提取信息并添加到DataFrame中
for result in results:
weibo_id = result.get('mid')
username = result.find('a', {'class': 'W_f14 W_fb S_txt1'}).text.strip()
time_str = result.find('a', {'class': 'S_txt2'}).text.strip()
content = result.find('div', {'class': 'WB_text W_f14'}).text.strip()
reposts = result.find('a', {'class': 'S_txt2', 'node-type': 'forward_btn_text'}).text.strip()
comments = result.find('a', {'class': 'S_txt2', 'node-type': 'comment_btn_text'}).text.strip()
likes = result.find('a', {'class': 'S_txt2', 'node-type': 'like_status'}).text.strip()
data = data.append({'微博ID': weibo_id, '用户名': username, '发布时间': time_str, '内容': content, '转发数': reposts, '评论数': comments, '点赞数': likes}, ignore_index=True)
# 点击下一页并等待页面加载完成
next_page = driver.find_element_by_xpath('//*[@id="pl_feedlist_index"]/div/div/div[1]/div[2]/a[11]')
next_page.click()
time.sleep(10)
# 将DataFrame保存为CSV文件
data.to_csv('weibo_data.csv', index=False)
# 关闭浏览器
driver.quit()
```
该程序将使用selenium模拟登录微博,并搜索指定的关键字。然后它将循环遍历搜索结果的每一页,提取微博的ID、用户名、发布时间、内容、转发数、评论数和点赞数,并将其保存到一个名为“weibo_data.csv”的CSV文件中。你需要将“your_username”和“your_password”替换为你自己的微博账号和密码,并将“关键字”替换为你想要搜索的关键字。
阅读全文
相关推荐
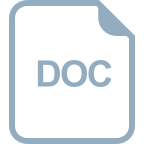
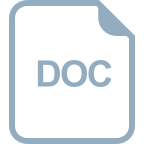
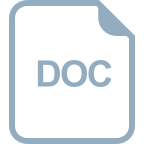
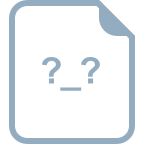



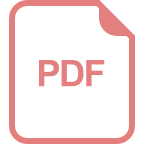
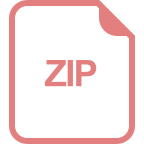
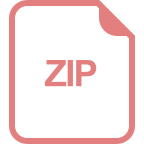






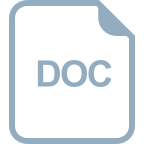
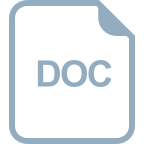
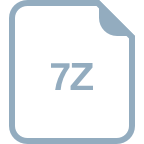