c++实现:作为Web服务器,接收浏览器的TCP连接请求,接收HTTP命令和邮件数据,将邮件保存在文件中;
时间: 2023-11-09 11:05:29 浏览: 128
要实现这个功能,我们可以使用C++中的网络编程库,如Boost.Asio或Poco.Net等。以下是基本的步骤:
1. 创建一个TCP服务器,并绑定一个端口,等待客户端连接。
2. 接受客户端的连接请求,并读取客户端发送的HTTP命令和邮件数据。
3. 将邮件数据保存在文件中。
下面是一个简单的实现:
```cpp
#include <iostream>
#include <fstream>
#include <boost/asio.hpp>
using boost::asio::ip::tcp;
int main() {
try {
// 创建io_context对象
boost::asio::io_context io_context;
// 创建一个TCP服务器对象并绑定端口
tcp::acceptor acceptor(io_context, tcp::endpoint(tcp::v4(), 8080));
// 循环等待客户端连接
while (true) {
// 接受客户端连接请求
tcp::socket socket(io_context);
acceptor.accept(socket);
// 读取客户端发送的HTTP命令和邮件数据
boost::asio::streambuf request_buf;
boost::asio::read_until(socket, request_buf, "\r\n\r\n");
// 解析HTTP命令
std::istream request_stream(&request_buf);
std::string http_method, path, http_version;
request_stream >> http_method >> path >> http_version;
// 读取邮件数据并保存到文件中
std::ofstream outfile("mail.txt");
std::string line;
while (std::getline(request_stream, line)) {
outfile << line << std::endl;
}
// 关闭socket
boost::system::error_code ec;
socket.shutdown(tcp::socket::shutdown_both, ec);
if (ec == boost::asio::error::eof)
ec.clear();
if (ec)
throw boost::system::system_error(ec);
}
}
catch (std::exception& e) {
std::cerr << "Exception: " << e.what() << std::endl;
}
return 0;
}
```
这是一个简单的实现,你可以根据实际需求进行修改和扩展。同时,注意安全性问题,例如输入验证和文件路径处理等。
阅读全文
相关推荐
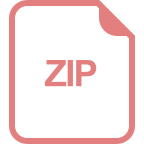
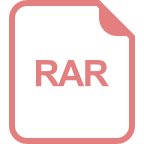
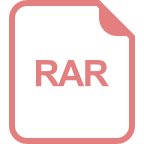
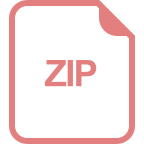
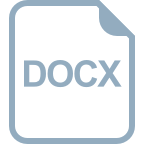
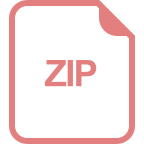
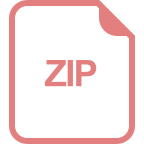
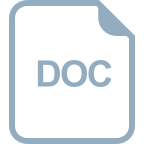
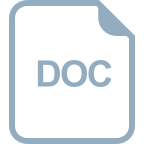
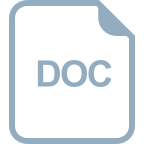
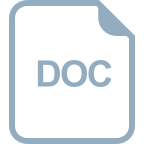
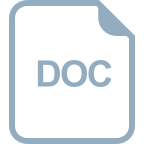
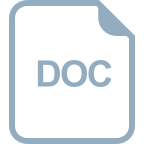
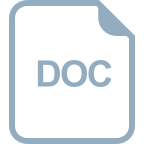
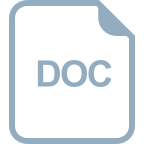