用matlab编写一段射线追踪的代码
时间: 2023-11-03 22:05:17 浏览: 342
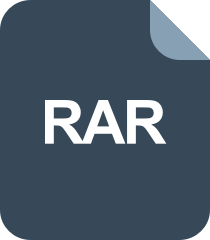
最短路径法弯曲射线追踪的MATLAB代码
以下是一段简单的射线追踪代码,实现了从视点向场景中投射射线,并计算射线与场景中物体的相交点:
```matlab
% 定义场景中的物体
sphere1 = struct('center', [0,0,5], 'radius', 1);
sphere2 = struct('center', [2,2,8], 'radius', 2);
objects = {sphere1, sphere2};
% 定义相机参数
width = 640;
height = 480;
fov = 60;
aspect_ratio = width/height;
camera_pos = [0,0,0];
camera_dir = [0,0,1];
camera_up = [0,1,0];
% 计算相机坐标系
w = -camera_dir/norm(camera_dir);
u = cross(camera_up, w)/norm(cross(camera_up, w));
v = cross(w, u);
% 进行射线追踪
for i = 1:height
for j = 1:width
% 计算当前像素对应的射线方向
x = (2*(j-0.5)/width-1)*aspect_ratio*tan(fov/2);
y = (1-2*(i-0.5)/height)*tan(fov/2);
ray_dir = normalize(x*u + y*v + w);
% 计算射线与场景中物体的相交点
t_min = Inf;
for k = 1:length(objects)
obj = objects{k};
oc = camera_pos - obj.center;
a = dot(ray_dir, ray_dir);
b = 2*dot(oc, ray_dir);
c = dot(oc, oc) - obj.radius^2;
discriminant = b^2 - 4*a*c;
if discriminant >= 0
t1 = (-b - sqrt(discriminant))/(2*a);
t2 = (-b + sqrt(discriminant))/(2*a);
if t1 >= 0 && t1 < t_min
t_min = t1;
end
if t2 >= 0 && t2 < t_min
t_min = t2;
end
end
end
% 在像素位置上显示相交点
if t_min < Inf
plot(j, i, 'r.');
else
plot(j, i, 'k.');
end
end
end
```
其中,我们使用了结构体来表示场景中的物体(这里只用了两个球体),并通过定义相机参数和计算相机坐标系来确定每个像素对应的射线方向。在计算每个射线与物体的相交点时,我们使用了二次方程求解,并记录下最近的相交点。最后,我们在对应的像素位置上显示相交点(用红色点表示相交,黑色点表示未相交)。
阅读全文
相关推荐
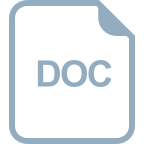
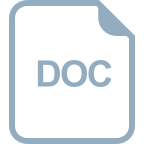


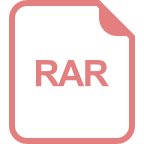
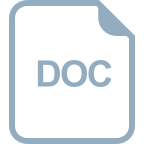
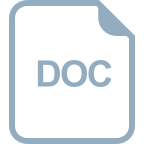
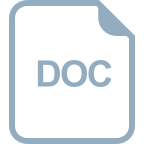
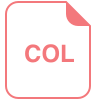
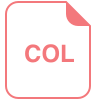
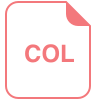
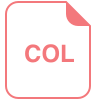
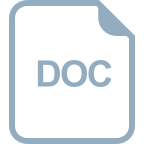
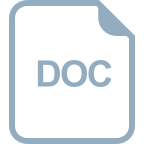