c语言循环队列
时间: 2023-07-24 14:06:33 浏览: 80
C语言中实现循环队列需要使用数组和指针,下面是一个简单的循环队列代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_QUEUE_SIZE 5
typedef struct {
int data[MAX_QUEUE_SIZE];
int front; // 队头指针
int rear; // 队尾指针
} Queue;
void init(Queue *queue) {
queue->front = queue->rear = 0;
}
int is_empty(Queue *queue) {
return queue->front == queue->rear;
}
int is_full(Queue *queue) {
return (queue->rear + 1) % MAX_QUEUE_SIZE == queue->front;
}
int enqueue(Queue *queue, int value) {
if (is_full(queue)) {
printf("Queue is full.\n");
return -1;
}
queue->data[queue->rear] = value;
queue->rear = (queue->rear + 1) % MAX_QUEUE_SIZE;
return 0;
}
int dequeue(Queue *queue) {
if (is_empty(queue)) {
printf("Queue is empty.\n");
return -1;
}
int value = queue->data[queue->front];
queue->front = (queue->front + 1) % MAX_QUEUE_SIZE;
return value;
}
int main() {
Queue queue;
init(&queue);
enqueue(&queue, 1);
enqueue(&queue, 2);
enqueue(&queue, 3);
enqueue(&queue, 4);
enqueue(&queue, 5);
enqueue(&queue, 6); // 队列已满,插入失败
printf("%d\n", dequeue(&queue));
printf("%d\n", dequeue(&queue));
printf("%d\n", dequeue(&queue));
printf("%d\n", dequeue(&queue));
printf("%d\n", dequeue(&queue));
printf("%d\n", dequeue(&queue)); // 队列已空,删除失败
return 0;
}
```
该代码中,`init` 函数用于初始化队列,`is_empty` 函数用于判断队列是否为空,`is_full` 函数用于判断队列是否已满,`enqueue` 函数用于插入元素到队列尾部,`dequeue` 函数用于从队列头部删除元素并返回其值。注意,在循环队列中,队尾指针和队头指针的更新需要使用取模运算。
阅读全文
相关推荐
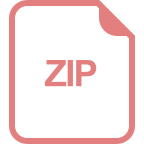
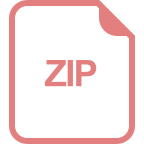
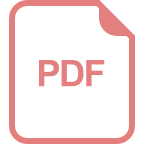


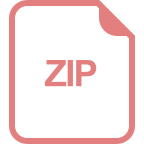
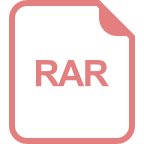
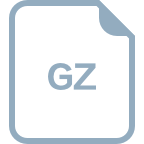
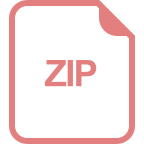
