watch( () => router.currentRoute, (to, from) => { // to: 当前路由对象 // from: 上一个路由对象 console.log("路由变化", to, from); } )没有效果
时间: 2024-03-02 12:49:50 浏览: 86
如果`watch`函数无法监听路由变化,可能是因为`currentRoute`对象是一个响应式对象,需要使用`.value`属性才能获取到其值。因此,可以将`watch`函数修改为如下形式:
```javascript
import { watch, onMounted } from 'vue'
import { useRouter } from 'vue-router'
export default {
setup() {
let router = null
onMounted(() => {
router = useRouter()
watch(() => router.currentRoute.value, (to, from) => {
// to: 当前路由对象
// from: 上一个路由对象
console.log('路由变化', to, from)
})
})
return {}
}
}
```
在上述代码中,我们使用`watch`函数监听`currentRoute.value`属性的变化。这样,当路由发生变化时,`watch`函数就会执行回调函数了。
相关问题
<div class="goods-icon"> <van-button type="info" color="#b8860b" @click="payFn" >立即购买</van-button > <van-button type="primary" color="#d81e06" @click="joinShopCar" >加入购物车</van-button > </div> </div> </template> <script> import { getGoodsDetailApi } from "@/api/details"; export default { data() { return { goodsId: " ", goodsData: [], current: 0, oldScrollTop: 0, scrollTop: 0, searchBgc: " rgba(0, 0, 0, 0.1)", show: false, opacity: 0, flag: true, timer: null, }; }, mounted() { this.handleScroll(); }, beforeDestroy() { window.removeEventListener("scroll", this.handleScrollFn); //离开当前组件别忘记移除事件监听哦 }, watch: { scrollTop: { handler: function (newValue, oldValue) { clearTimeout(this.timer); this.timer = setTimeout(() => { if (newValue == window.scrollY) { this.oldScrollTop = newValue; if (this.oldScrollTop > 100) { this.show = true; } else { this.show = false; } } else { if (this.oldScrollTop == oldValue) { // console.log("滚动开始"); } } }, 20); }, immediate: true, }, }, methods: { handleScroll() { this.handleScrollFn = () => { this.scrollTop = window.scrollY; }; window.addEventListener("scroll", this.handleScrollFn); }, // 返回上一页 onClickLeft() { this.$router.go(-1); }, // 切换商品详情页 onChange(index) { this.current = index; }, // 加入购物车 joinShopCar() { alert("加入购物车成功"); let shopcarList = JSON.parse(localStorage.getItem("shopcar")) || []; console.log(shopcarList); this.goodsData.num = 1; this.goodsData.isChecked = false; let ind = shopcarList.findIndex((ele) => { return ele.id == this.goodsId; }); console.log(ind); // 如果有相同的返回该数据的下标,没有则返回-1 if (ind != -1) { shopcarList[ind].num++; } else { shopcarList.push(this.goodsData); } localStorage.setItem("shopcar", JSON.stringify(shopcarList)); this.$router.push("/shopcar"); }, // 立即结算 payFn() { localStorage.setItem("goodspay", JSON.stringify(this.goodsData)); this.$router.push("/pay"); }, },跳转到购物车页面为什么不会高亮显示
可能是因为在跳转到购物车页面的代码中没有设置高亮显示的相关逻辑。要实现高亮显示,可以在购物车页面组件的 mounted 钩子函数中获取当前路由信息,然后根据路由信息设置高亮样式。例如可以通过 $route 对象中的 path 属性获取当前路由路径,然后通过 DOM 操作设置高亮样式。具体实现方式可以参考 Vue 官方文档中的路由导航守卫相关内容。
watch: { $route: { immediate: true, handler (route) { this.updateCurrentPath(route.path) this.list = route.matched.map(item => { return { name: item.name, label: this.routesMap.get(item.name) } }) } } }改用setup和ts编写这个函数
好的,以下是使用 `setup` 和 TypeScript 重写这个函数的示例代码:
```typescript
import { defineComponent, onMounted, onBeforeUnmount, ref } from 'vue'
import { RouteLocationNormalized } from 'vue-router'
export default defineComponent({
setup() {
const currentPath = ref('')
const routesMap = new Map([
['home', '首页'],
['about', '关于'],
['contact', '联系我们'],
])
const list = ref<Array<{ name: string; label: string }>>([])
const updateCurrentPath = (path: string) => {
currentPath.value = path
}
const updateList = (route: RouteLocationNormalized) => {
list.value = route.matched.map(item => ({
name: item.name as string,
label: routesMap.get(item.name as string) as string,
}))
}
onMounted(() => {
updateCurrentPath(window.location.pathname)
updateList({ ...$route })
})
onBeforeUnmount(() => {
// do cleanup work here
})
return {
currentPath,
list,
}
},
})
```
需要注意的是,在 `options API` 中,我们可以直接使用 `$route` 对象来监听路由变化,而在 `setup` 函数中,我们需要通过 `useRoute` 函数来获取 `$route` 对象。此外,在 `setup` 函数中,我们还需要手动处理组件的生命周期钩子函数。
阅读全文
相关推荐
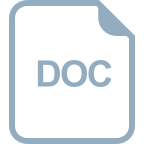
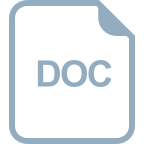
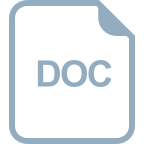

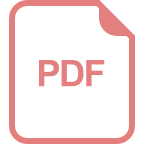
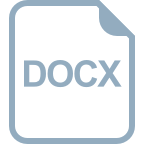
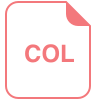









