用Python做一个黄金矿工
时间: 2023-11-19 14:35:09 浏览: 142
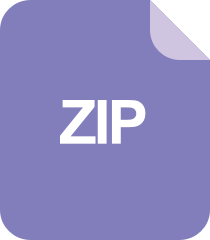
python,pygame自制黄金矿工小游戏
好的,我可以为您提供一些思路和建议。
首先,您需要安装pygame这个Python库,它是用于创建游戏的工具包。然后,您可以开始编写您的黄金矿工游戏。
游戏的主要流程是这样的:玩家控制一个矿工,通过挖掘地下的矿石和宝石,来获取分数。在游戏过程中,玩家需要避开一些障碍物,比如炸药和毒药,否则就会损失生命值。
为了实现这个游戏,您需要创建几个元素,包括矿工、矿石和宝石、障碍物等。然后,您需要编写一些逻辑代码,比如:玩家使用方向键控制矿工的移动,矿工挖矿和收集宝石的动作,障碍物的生成和移动等。
下面是一个简单的代码示例,可以帮助您开始编写您的黄金矿工游戏:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口
width = 800
height = 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("Gold Miner")
# 加载游戏图片
miner_image = pygame.image.load("miner.png")
stone_image = pygame.image.load("stone.png")
gem_image = pygame.image.load("gem.png")
bomb_image = pygame.image.load("bomb.png")
poison_image = pygame.image.load("poison.png")
# 设置游戏元素的大小和位置
miner_rect = miner_image.get_rect()
miner_rect.centerx = width // 2
miner_rect.bottom = height - 10
stone_rect = stone_image.get_rect()
stone_rect.centerx = random.randint(50, width - 50)
stone_rect.centery = random.randint(50, height - 200)
gem_rect = gem_image.get_rect()
gem_rect.centerx = random.randint(50, width - 50)
gem_rect.centery = random.randint(50, height - 200)
bomb_rect = bomb_image.get_rect()
bomb_rect.centerx = random.randint(50, width - 50)
bomb_rect.centery = random.randint(50, height - 200)
poison_rect = poison_image.get_rect()
poison_rect.centerx = random.randint(50, width - 50)
poison_rect.centery = random.randint(50, height - 200)
# 设置游戏元素的移动速度
stone_speed = 5
gem_speed = 3
bomb_speed = 4
poison_speed = 3
# 设置游戏分数和生命值
score = 0
life = 3
# 设置游戏字体
font = pygame.font.Font(None, 36)
# 游戏主循环
while True:
# 事件处理
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
miner_rect.move_ip(-10, 0)
elif event.key == pygame.K_RIGHT:
miner_rect.move_ip(10, 0)
# 更新游戏元素的位置
stone_rect.move_ip(0, stone_speed)
gem_rect.move_ip(0, gem_speed)
bomb_rect.move_ip(0, bomb_speed)
poison_rect.move_ip(0, poison_speed)
# 判断矿工是否碰到了矿石或宝石
if miner_rect.colliderect(stone_rect):
score += 10
stone_rect.centerx = random.randint(50, width - 50)
stone_rect.centery = random.randint(50, height - 200)
elif miner_rect.colliderect(gem_rect):
score += 50
gem_rect.centerx = random.randint(50, width - 50)
gem_rect.centery = random.randint(50, height - 200)
# 判断矿工是否碰到了障碍物
if miner_rect.colliderect(bomb_rect):
life -= 1
bomb_rect.centerx = random.randint(50, width - 50)
bomb_rect.centery = random.randint(50, height - 200)
elif miner_rect.colliderect(poison_rect):
life -= 1
poison_rect.centerx = random.randint(50, width - 50)
poison_rect.centery = random.randint(50, height - 200)
# 绘制游戏元素和分数和生命值
screen.fill((255, 255, 255))
screen.blit(miner_image, miner_rect)
screen.blit(stone_image, stone_rect)
screen.blit(gem_image, gem_rect)
screen.blit(bomb_image, bomb_rect)
screen.blit(poison_image, poison_rect)
score_text = font.render("Score: {}".format(score), True, (0, 0, 0))
screen.blit(score_text, (10, 10))
life_text = font.render("Life: {}".format(life), True, (0, 0, 0))
screen.blit(life_text, (width - 80, 10))
# 判断游戏是否结束
if life <= 0:
gameover_text = font.render("Game Over", True, (255, 0, 0))
screen.blit(gameover_text, (width // 2 - 60, height // 2 - 20))
pygame.display.update()
pygame.time.delay(3000)
pygame.quit()
exit()
# 更新游戏画面
pygame.display.update()
```
代码中的图片文件需要您自己准备,并且需要放在与代码文件相同的目录下。您可以根据您自己的需要,对代码进行修改和扩展,来创建您自己的黄金矿工游戏。
阅读全文
相关推荐
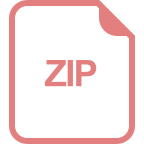















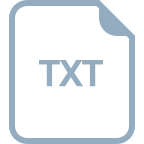