给出使用DFS解决七桥问题的python代码
时间: 2023-06-04 12:04:33 浏览: 125
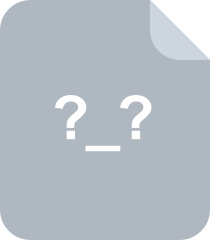
基于python的DFS算法设计与实现
以下是使用DFS解决七桥问题的Python代码示例:
```python
def dfs(graph, v, visited):
visited.add(v)
for neighbor in graph[v]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
def has_eulerian_path(graph):
odd_vertices = [v for v in graph if len(graph[v]) % 2 == 1]
if len(odd_vertices) != 2:
return False
visited = set()
dfs(graph, odd_vertices[0], visited)
return len(visited) == len(graph)
graph = {
'A': ['B', 'C', 'D'],
'B': ['A', 'D'],
'C': ['A', 'D'],
'D': ['A', 'B', 'C']
}
print(has_eulerian_path(graph)) # True
```
注:七桥问题也称为Königsberg问题,是欧拉于1735年解决的一个古老的数学难题。该问题描述了一个四岛之城的地图,其中有七座桥,是否可能从任意一个岛屿开始,穿过每座桥一次且只穿过一次,最终回到起点。
阅读全文
相关推荐
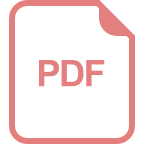
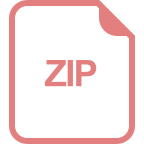

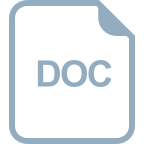
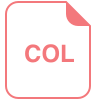
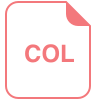
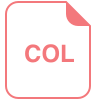
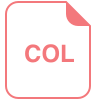
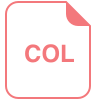
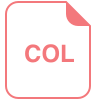
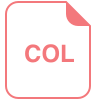
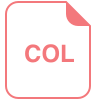
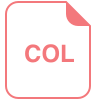
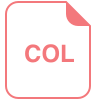
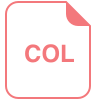
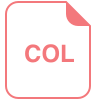
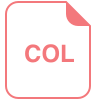
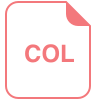