Vue3这一个弹幕从下往上显示数据的页面
时间: 2024-02-06 21:13:05 浏览: 227
以下是一个使用Vue3实现从下往上显示弹幕的页面的示例代码:
```html
<template>
<div class="danmu-container">
<div class="danmu-box" v-for="(danmu, index) in danmuList" :key="index" :style="{ bottom: (index * lineHeight) + 'px' }">{{ danmu }}</div>
</div>
</template>
<script>
import { ref, onMounted, onUnmounted } from 'vue';
export default {
name: 'Danmu',
setup() {
const danmuList = ref([]);
const lineHeight = 60; // 弹幕行高
const danmuHeight = 40; // 弹幕高度
const danmuSpeed = 2; // 弹幕速度,每秒移动的像素数
// 添加弹幕
function addDanmu(text) {
danmuList.value.push(text);
}
// 移除弹幕
function removeDanmu(index) {
danmuList.value.splice(index, 1);
}
// 弹幕移动
function moveDanmu() {
for (let i = 0; i < danmuList.value.length; i++) {
const danmuBox = document.querySelectorAll('.danmu-box')[i];
const bottom = parseFloat(danmuBox.style.bottom);
if (bottom >= (lineHeight * (i + 1))) {
removeDanmu(i);
i--;
} else {
danmuBox.style.bottom = (bottom + danmuSpeed) + 'px';
}
}
}
// 定时器启动弹幕移动
let timer = null;
function startTimer() {
timer = setInterval(() => {
moveDanmu();
}, 16);
}
// 清除定时器
function clearTimer() {
clearInterval(timer);
}
// 组件挂载时启动定时器
onMounted(() => {
startTimer();
});
// 组件卸载时清除定时器
onUnmounted(() => {
clearTimer();
});
return {
danmuList,
lineHeight,
danmuHeight,
addDanmu,
removeDanmu,
moveDanmu,
startTimer,
clearTimer
};
}
};
</script>
<style scoped>
.danmu-container {
position: relative;
width: 100%;
height: 100%;
overflow: hidden;
}
.danmu-box {
position: absolute;
left: 0;
width: 100%;
height: 40px;
line-height: 40px;
font-size: 24px;
color: #FFF;
white-space: nowrap;
text-shadow: 1px 1px 2px rgba(0, 0, 0, 0.5);
}
</style>
```
上面的代码中,我们使用了Vue3的Composition API来实现弹幕从下往上显示的效果。通过`ref`函数来创建响应式变量,使用`onMounted`和`onUnmounted`函数来处理组件挂载和卸载时的操作。
页面中的弹幕内容保存在`danmuList`数组中,通过`v-for`指令渲染到页面上。根据弹幕的数量和弹幕的高度,计算出弹幕的位置,并使用`bottom`样式将弹幕显示在页面上。
弹幕的移动通过定时器来完成,每隔16毫秒移动一次弹幕。在移动弹幕时,判断弹幕是否已经移动到了页面顶部,如果是,则从弹幕列表中删除该弹幕。最后,使用`setInterval`函数启动定时器,在组件卸载时清除定时器,实现弹幕的自动滚动效果。
阅读全文
相关推荐
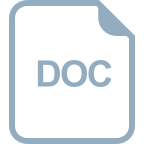
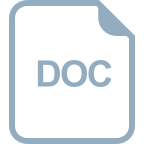
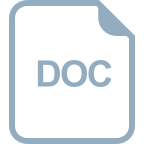



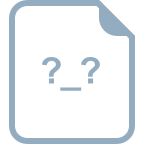
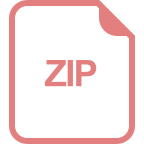
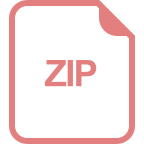
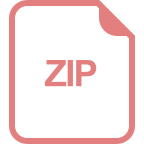
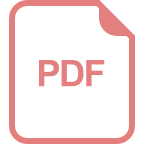
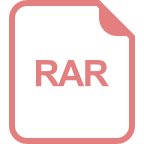
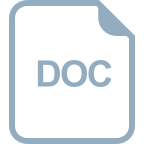
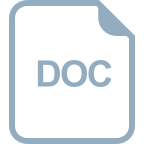
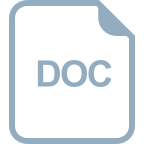
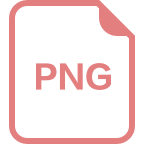