代码详细演示设计一个中台监控,中台接口要能记录服务调用次数,服务调用来源,起始时间,结束时间自定义注解,发送消息到MQ,数据交互平台从MQ消费消息,插入到数据库
时间: 2024-03-05 14:52:14 浏览: 120
好的,这里是一个可以记录服务调用次数、调用来源、起始时间和结束时间的中台监控,同样使用Spring AOP实现自定义注解,用于标识需要进行监控的接口方法,然后使用Redis记录调用次数和调用来源,记录起始时间和结束时间,发送消息到MQ,数据交互平台从MQ消费消息,并将消息中的信息插入到数据库中。
1. 定义自定义注解:Monitor
```java
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface Monitor {
}
```
2. 使用AOP实现监控逻辑
```java
@Aspect
@Component
public class MonitorAspect {
@Autowired
private RedisTemplate<String, Object> redisTemplate;
@Autowired
private RabbitTemplate rabbitTemplate;
@Before("@annotation(cn.example.Monitor)")
public void doBefore(JoinPoint joinPoint) {
// 获取注解标识的方法名
String methodName = joinPoint.getSignature().getName();
// 获取调用来源
String source = getRequestSource();
// 记录调用次数
String countKey = methodName + ":" + source + ":count";
redisTemplate.opsForValue().increment(countKey);
// 记录起始时间
String startTimeKey = methodName + ":" + source + ":startTime";
redisTemplate.opsForValue().set(startTimeKey, System.currentTimeMillis());
}
@AfterReturning("@annotation(cn.example.Monitor)")
public void doAfterReturning(JoinPoint joinPoint) {
// 获取注解标识的方法名
String methodName = joinPoint.getSignature().getName();
// 获取调用来源
String source = getRequestSource();
// 记录结束时间
String endTimeKey = methodName + ":" + source + ":endTime";
redisTemplate.opsForValue().set(endTimeKey, System.currentTimeMillis());
// 发送消息到MQ
Map<String, Object> message = new HashMap<>();
message.put("methodName", methodName);
message.put("source", source);
message.put("count", redisTemplate.opsForValue().get(methodName + ":" + source + ":count"));
message.put("startTime", redisTemplate.opsForValue().get(methodName + ":" + source + ":startTime"));
message.put("endTime", redisTemplate.opsForValue().get(methodName + ":" + source + ":endTime"));
rabbitTemplate.convertAndSend("monitor.exchange", "monitor.routingKey", message);
}
/**
* 获取请求来源
*/
private String getRequestSource() {
// 从请求头或者请求参数中获取来源信息
// ...
return "source";
}
}
```
3. 数据交互平台从MQ中消费消息并插入到数据库
```java
@Component
public class MonitorConsumer {
@Autowired
private MonitorRepository monitorRepository;
@RabbitListener(queues = "monitor.queue")
public void handleMessage(Map<String, Object> message) {
MonitorEntity entity = new MonitorEntity();
entity.setMethodName(message.get("methodName").toString());
entity.setSource(message.get("source").toString());
entity.setCount(Integer.parseInt(message.get("count").toString()));
entity.setStartTime(new Date(Long.parseLong(message.get("startTime").toString())));
entity.setEndTime(new Date(Long.parseLong(message.get("endTime").toString())));
monitorRepository.save(entity);
}
}
```
以上就是一个可以记录服务调用次数、调用来源、起始时间和结束时间的中台监控,如果需要将时间戳转换为日期可以使用`java.util.Date`进行转换。
阅读全文
相关推荐
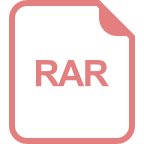

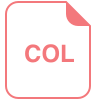
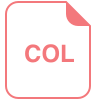
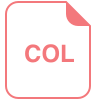
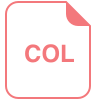
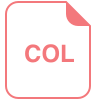
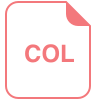
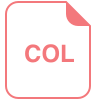
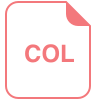
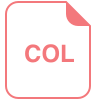
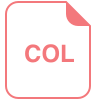
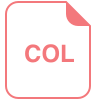
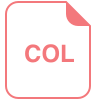
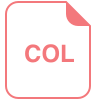
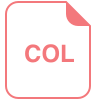