C语言统计字符串S2的每个字符在S1中出现的次数
时间: 2023-06-07 08:08:24 浏览: 171
好的,这是一个统计字符出现次数的问题,可以回答。以下是代码示例:
```c
#include <stdio.h>
#include <string.h>
int main() {
char s1[100], s2[100];
int freq[128] = {0};
printf("请输入字符串S1:\n");
gets(s1);
printf("请输入字符串S2:\n");
gets(s2);
// 统计S1中每个字符的出现频率
for (int i = 0; i < strlen(s1); i++) {
freq[(int)s1[i]]++;
}
// 输出S2中每个字符在S1中出现的次数
printf("S2中每个字符在S1中出现的次数:\n");
for (int i = 0; i < strlen(s2); i++) {
printf("%c:%d\n", s2[i], freq[(int)s2[i]]);
}
return 0;
}
```
注意:此代码示例仅为参考,实际应用时需要加入对输入的合法性检查。
相关问题
C语言编程统计字符串s2中的每个字符在字符串s1中出现的次数
好的,这是一个编程问题。您可以通过遍历字符串s1和s2,然后使用一个计数器来统计每个字符在字符串s1中出现的次数。以下是一个示例C程序:
```
#include <stdio.h>
#include <string.h>
void count_chars(char *s1, char *s2)
{
int counts[128] = {0}; // assume ASCII characters
// count characters in s1
for (int i = 0; i < strlen(s1); i++) {
counts[(int)s1[i]]++;
}
// print character counts for s2
printf("Character counts for s2:\n");
for (int i = 0; i < strlen(s2); i++) {
int count = counts[(int)s2[i]];
printf("%c: %d\n", s2[i], count);
}
}
int main()
{
char s1[] = "Hello, world!";
char s2[] = "lo";
count_chars(s1, s2);
return 0;
}
```
这个程序的输出应该是:
```
Character counts for s2:
l: 3
o: 2
```
判断字符串s1中是否包含字符串s2,用C语言
可以使用C语言中的字符串函数来判断一个字符串是否包含另一个字符串。
其中一个比较常用的函数是`strstr()`,该函数可以在一个字符串中搜索另一个字符串,并返回第一次出现该字符串的地址。如果没找到,返回NULL。
以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char s1[100], s2[100];
printf("Enter string s1: ");
gets(s1);
printf("Enter string s2: ");
gets(s2);
if (strstr(s1, s2) != NULL)
printf("%s is found in %s\n", s2, s1);
else
printf("%s is not found in %s\n", s2, s1);
return 0;
}
```
在上面的代码中,我们使用`gets()`函数从标准输入中读取字符串s1和s2。然后,我们使用`strstr()`函数来查找s2是否在s1中出现。如果找到了,就输出“s2 is found in s1”,否则输出“s2 is not found in s1”。
阅读全文
相关推荐
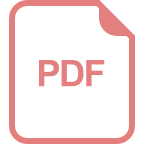
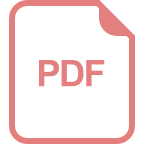
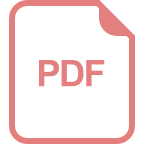













