任务: 采用面向对象的思想构建“超市购物”,任务描述如下: 超市的货物包括生活用品和学习用品等,购物者根据货物名能够查找是否有该货物,根据货号能查找该货物的价格,打印购物清单。 ,统计货品销售的数量。
时间: 2023-09-17 07:06:01 浏览: 176
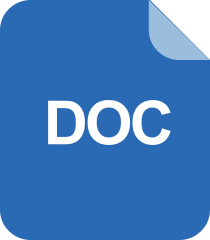
面向对象技术与UML技术课程设计-网上购物系统

### 回答1:
我可以为您提供使用面向对象的思想构建“超市购物”的程序代码。请看以下代码:
class Commodity:
def __init__(self, id, name, price, type):
self.id = id
self.name = name
self.price = price
self.type = type
self.quantity_sold = 0
def __str__(self):
return self.name + ": " + str(self.price) + "元" + "(ID:" + str(self.id) + ")"
def sold(self, quantity=1):
self.quantity_sold += quantity
class Supermarket:
def __init__(self, name):
self.name = name
self.commodities = []
def add_commodity(self, commodity):
self.commodities.append(commodity)
def find_commodity_by_name(self, name):
for commodity in self.commodities:
if commodity.name == name:
return commodity
return None
def find_commodity_by_id(self, id):
for commodity in self.commodities:
if commodity.id == id:
return commodity
return None
def generate_shopping_list(self, purchases):
print("-------------购物清单-------------")
total_price = 0
for purchase in purchases:
commodity = self.find_commodity_by_name(purchase["name"])
if commodity is None:
print("很抱歉,没有货品" + purchase["name"])
continue
total_price += commodity.price * purchase["quantity"]
commodity.sold(purchase["quantity"])
print(commodity.name + " x" + str(purchase["quantity"]) + " = " + str(commodity.price*purchase["quantity"]) + "元")
print("----------------------------------")
return total_price
# 测试代码
if __name__ == "__main__":
# 创建超市
supermarket = Supermarket("大卖场")
# 添加货品
commodity1 = Commodity(1, "衣服", 100, "生活用品")
commodity2 = Commodity(2, "书", 50, "学习用品")
supermarket.add_commodity(commodity1)
supermarket.add_commodity(commodity2)
# 查找货品
print(supermarket.find_commodity_by_name("衣服"))
print(supermarket.find_commodity_by_id(2))
# 生成购物清单
purchases = [
{"name":"衣服", "quantity":2},
{"name":"书", "quantity":3},
{"name":"电视", "quantity":1},
]
total_price = supermarket.generate_shopping_list(purchases)
print("总价:" + str(total_price) + "元")
# 查看销售数量
print(commodity1.quantity_sold)
print(commodity2.quantity_sold)
以上是使用面向对象的思想构建“超市购物”的完整代码,您可以在此基础上进行修改或调整,以满足您的具体需求。
### 回答2:
超市购物的面向对象构建可以分为三个类:商品类、购物者类和超市类。
1. 商品类:包括生活用品和学习用品,属性包括货物名、货号和价格。
- 货物名用来查找是否有该货物。
- 货号用来查找该货物的价格。
2. 购物者类:负责购物行为,包括查找商品、添加购物清单和统计货品销售数量等功能。
- 查找商品功能:通过货物名查找是否有该货物。
- 添加购物清单功能:将购买的商品添加到购物清单中。
- 统计货品销售数量功能:记录购物者购买的商品数量。
3. 超市类:负责管理商品信息和购物者行为。
- 商品管理功能:维护商品信息,包括货物名、货号和价格等。
- 购物者管理功能:管理购物者的行为,包括查找商品和统计销售数量等。
购物流程如下:
1. 超市管理员维护商品信息,包括添加新货物的属性:货物名、货号和价格。
2. 购物者根据商品的货物名查找是否有该货物。
3. 如果有该货物,则购物者可以选择购买该商品,并将商品添加到购物清单中。
4. 购物者可以继续查找其他商品并进行购买操作。
5. 当购物结束时,购物者可以打印购物清单,并统计购买商品的数量。
这样,通过面向对象的思想,构建了一个超市购物的模型,包括商品类、购物者类和超市类,并实现了根据货物名查找商品、根据货号查找价格、添加购物清单和统计销售数量等功能。这样可以更好地管理超市的商品信息和购物行为。
### 回答3:
为实现超市购物系统,可以创建两个类:商品类和购物车类。
商品类(Product)具有以下属性和方法:货物名(name),货号(id),价格(price),数量(quantity)。
- 属性可以通过构造函数传递给对象。
- 提供获取货物名和货号的方法。
- 提供获取价格和数量的方法。
- 提供设置价格和数量的方法,用于更新货物的相关信息。
购物车类(ShoppingCart)具有以下属性和方法:购物车中的商品列表(products),购物清单(bill)。
- 属性可以通过构造函数传递给对象。
- 提供向购物车添加商品的方法,将商品对象添加到商品列表中。
- 提供根据货物名查找是否有该货物的方法,遍历商品列表并比较货物名。
- 提供根据货号查找商品价格的方法,遍历商品列表并比较货号。
- 提供打印购物清单的方法,遍历商品列表并输出相关信息。
- 提供统计货品销售数量的方法,遍历商品列表并累加数量。
示例代码如下:
```python
class Product:
def __init__(self, name, id, price, quantity):
self.name = name
self.id = id
self.price = price
self.quantity = quantity
def get_name(self):
return self.name
def get_id(self):
return self.id
def get_price(self):
return self.price
def get_quantity(self):
return self.quantity
def set_price(self, price):
self.price = price
def set_quantity(self, quantity):
self.quantity = quantity
class ShoppingCart:
def __init__(self):
self.products = []
def add_product(self, product):
self.products.append(product)
def find_product_by_name(self, name):
for product in self.products:
if product.get_name() == name:
return product
return None
def find_price_by_id(self, id):
for product in self.products:
if product.get_id() == id:
return product.get_price()
return None
def print_bill(self):
for product in self.products:
print(f"商品名:{product.get_name()},货号:{product.get_id()},价格:{product.get_price()},数量:{product.get_quantity()}")
def count_sales_quantity(self):
total_quantity = 0
for product in self.products:
total_quantity += product.get_quantity()
return total_quantity
# 示例运行
cart = ShoppingCart()
product1 = Product("洗发水", "A001", 10.0, 2)
product2 = Product("笔记本", "B002", 5.0, 5)
cart.add_product(product1)
cart.add_product(product2)
print(cart.find_product_by_name("洗发水"))
print(cart.find_price_by_id("B002"))
cart.print_bill()
print(cart.count_sales_quantity())
```
这样,我们就可以通过创建商品对象,添加到购物车对象中,并通过购物车对象进行购物、查询价格、打印购物清单和统计销售数量。
阅读全文
相关推荐
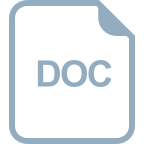
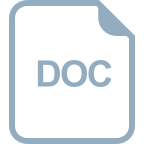


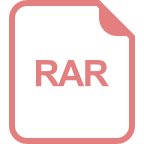
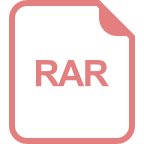
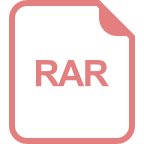
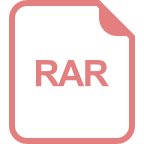
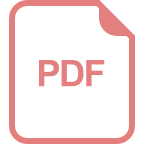
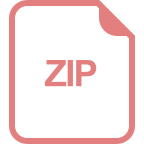
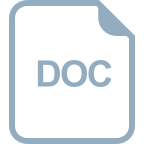
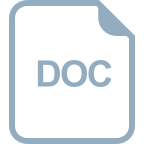
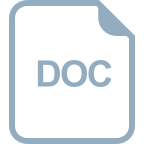
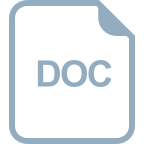
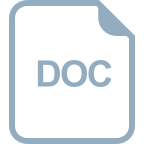
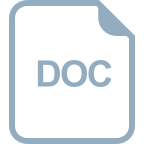
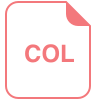
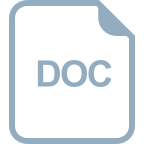