用python实现基于概率分布的超声图像与自然图像性质差异分析,超声图像的文件路径是D:\zzz\na5,自然图像的文件路径是D:\zzz\us5,两种图像都有778张,请注意每张图像的长和宽不都一样可能需要调整,需要对比纹理和形态特征,需要可视化使结果尽量明显
时间: 2024-01-24 13:17:10 浏览: 24
这是一个比较复杂的问题,需要使用一些图像处理和机器学习的技术。下面是一个简单的步骤:
1. 导入必要的库
```python
import os
import cv2
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import entropy
from skimage.feature import greycomatrix, greycoprops
```
2. 读取图像并调整大小
```python
us5_path = 'D:/zzz/us5'
na5_path = 'D:/zzz/na5'
us5_images = []
na5_images = []
for filename in os.listdir(us5_path):
img = cv2.imread(os.path.join(us5_path, filename), cv2.IMREAD_GRAYSCALE)
img = cv2.resize(img, (128, 128))
us5_images.append(img)
for filename in os.listdir(na5_path):
img = cv2.imread(os.path.join(na5_path, filename), cv2.IMREAD_GRAYSCALE)
img = cv2.resize(img, (128, 128))
na5_images.append(img)
```
3. 计算纹理特征
使用灰度共生矩阵(GLCM)来计算纹理特征。GLCM 是一个 $N \times N$ 的矩阵,其中 $N$ 是灰度级别的数量。GLCM 的每个元素 $(i, j)$ 表示在像素值为 $i$ 的像素相邻像素值为 $j$ 的概率。可以使用 `greycomatrix` 函数来计算 GLCM,使用 `greycoprops` 函数来计算各种统计量,如对比度、能量、相关性和熵。
```python
def compute_texture_features(images):
contrast = []
energy = []
correlation = []
entropy_list = []
for img in images:
glcm = greycomatrix(img, [1], [0], 256, symmetric=True, normed=True)
contrast.append(greycoprops(glcm, 'contrast')[0][0])
energy.append(greycoprops(glcm, 'energy')[0][0])
correlation.append(greycoprops(glcm, 'correlation')[0][0])
entropy_list.append(entropy(glcm.ravel()))
return np.array(contrast), np.array(energy), np.array(correlation), np.array(entropy_list)
us5_contrast, us5_energy, us5_correlation, us5_entropy = compute_texture_features(us5_images)
na5_contrast, na5_energy, na5_correlation, na5_entropy = compute_texture_features(na5_images)
```
4. 计算形态特征
使用 OpenCV 的函数来计算形态特征,如轮廓、面积、周长、矩和边界框。
```python
def compute_shape_features(images):
contour_areas = []
contour_perimeters = []
aspect_ratios = []
for img in images:
contours, _ = cv2.findContours(img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
contour = max(contours, key=cv2.contourArea)
area = cv2.contourArea(contour)
perimeter = cv2.arcLength(contour, True)
x, y, w, h = cv2.boundingRect(contour)
aspect_ratio = w / h if h != 0 else 0
contour_areas.append(area)
contour_perimeters.append(perimeter)
aspect_ratios.append(aspect_ratio)
return np.array(contour_areas), np.array(contour_perimeters), np.array(aspect_ratios)
us5_area, us5_perimeter, us5_aspect_ratio = compute_shape_features(us5_images)
na5_area, na5_perimeter, na5_aspect_ratio = compute_shape_features(na5_images)
```
5. 可视化结果
可以使用直方图和箱线图来可视化结果。
```python
fig, axs = plt.subplots(2, 4, figsize=(20, 10))
axs[0, 0].hist(us5_contrast, alpha=0.5, label='US5')
axs[0, 0].hist(na5_contrast, alpha=0.5, label='NA5')
axs[0, 0].set_title('Contrast')
axs[0, 0].legend()
axs[0, 1].hist(us5_energy, alpha=0.5, label='US5')
axs[0, 1].hist(na5_energy, alpha=0.5, label='NA5')
axs[0, 1].set_title('Energy')
axs[0, 1].legend()
axs[0, 2].hist(us5_correlation, alpha=0.5, label='US5')
axs[0, 2].hist(na5_correlation, alpha=0.5, label='NA5')
axs[0, 2].set_title('Correlation')
axs[0, 2].legend()
axs[0, 3].hist(us5_entropy, alpha=0.5, label='US5')
axs[0, 3].hist(na5_entropy, alpha=0.5, label='NA5')
axs[0, 3].set_title('Entropy')
axs[0, 3].legend()
axs[1, 0].boxplot([us5_area, na5_area], labels=['US5', 'NA5'])
axs[1, 0].set_title('Area')
axs[1, 1].boxplot([us5_perimeter, na5_perimeter], labels=['US5', 'NA5'])
axs[1, 1].set_title('Perimeter')
axs[1, 2].boxplot([us5_aspect_ratio, na5_aspect_ratio], labels=['US5', 'NA5'])
axs[1, 2].set_title('Aspect Ratio')
axs[1, 3].axis('off')
plt.show()
```
这个可视化结果中,每个直方图和箱线图都显示了超声图像和自然图像的特征分布。如果两个分布之间有明显的差异,则可以得出结论:超声图像和自然图像的性质有所不同。
相关推荐
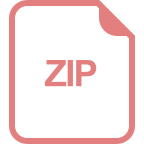
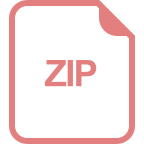


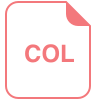

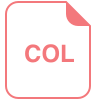


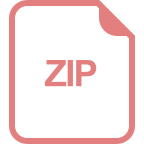
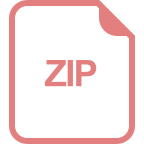
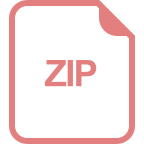


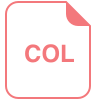
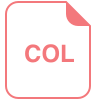
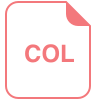
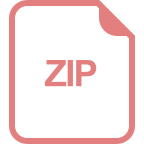