如何判断基类指针指向的派生类是否相同
时间: 2023-09-11 13:10:56 浏览: 94
可以使用dynamic_cast运算符将基类指针转换为派生类指针,如果转换成功,则说明基类指针指向的派生类与我们要比较的派生类是同一个类,否则指向的是不同的派生类。例如:
```
class Base { ... };
class Derived1 : public Base { ... };
class Derived2 : public Base { ... };
void func(Base* ptr) {
if (Derived1* d1 = dynamic_cast<Derived1*>(ptr)) {
// ptr指向Derived1
} else if (Derived2* d2 = dynamic_cast<Derived2*>(ptr)) {
// ptr指向Derived2
} else {
// ptr指向其他类型
}
}
```
在这个例子中,如果ptr指向Derived1,则dynamic_cast返回一个Derived1指针,判断为真;如果ptr指向Derived2,则dynamic_cast返回一个空指针,判断为假;如果ptr指向其他类型,则dynamic_cast返回一个空指针,同样判断为假。
相关问题
在C++中能直接将二义性基类的指针指向派生类对象吗?
在C++中,如果一个派生类从多个基类派生而来,且这些基类中有相同的成员函数或数据成员,那么在派生类中就会出现二义性。此时,如果直接将二义性基类的指针指向派生类对象,就会导致编译器无法确定要访问哪个基类的成员,从而产生编译错误。
为了解决这个问题,可以使用作用域解析运算符来明确指定要访问的基类成员。例如,假设派生类D继承了基类B1和B2,且这两个基类都有一个成员函数f(),那么在D中访问B1的f()可以使用B1::f()的方式,访问B2的f()可以使用B2::f()的方式。
总之,不能直接将二义性基类的指针指向派生类对象。如果要使用指针指向派生类对象中的成员,需要使用作用域解析运算符来指定要访问的基类成员。
c++中,什么具体的应用场景下会用基类的指针指向派生类的对象,给出具体的例子
在C++中,基类的指针指向派生类的对象的应用场景很多,这里给出几个例子:
1. 多态性:当需要对不同的派生类对象进行相同的操作时,可以使用基类的指针指向派生类的对象,从而实现多态性。比如,定义一个动物Animal基类,派生出狗Dog和猫Cat两个类,它们都有一个makeSound()函数。我们可以定义一个指向Animal类型的指针,然后通过指针调用makeSound()函数,这样就可以实现对不同的派生类对象进行相同的操作了。
```c++
class Animal {
public:
virtual void makeSound() { cout << "Animal makes sound." << endl; }
};
class Dog : public Animal {
public:
void makeSound() { cout << "Dog barks." << endl; }
};
class Cat : public Animal {
public:
void makeSound() { cout << "Cat meows." << endl; }
};
int main() {
Animal* animal1 = new Dog();
Animal* animal2 = new Cat();
animal1->makeSound(); // output: Dog barks.
animal2->makeSound(); // output: Cat meows.
return 0;
}
```
2. 多态性和动态绑定:在使用指向派生类的基类指针时,如果在基类中将函数声明为虚函数,那么编译器会在运行时动态绑定函数的调用地址,从而实现多态性。比如,如果在上面的例子中,将makeSound()函数声明为虚函数,那么在运行时会根据指针所指向的对象类型来调用相应的makeSound()函数。
```c++
class Animal {
public:
virtual void makeSound() { cout << "Animal makes sound." << endl; }
};
class Dog : public Animal {
public:
void makeSound() { cout << "Dog barks." << endl; }
};
class Cat : public Animal {
public:
void makeSound() { cout << "Cat meows." << endl; }
};
int main() {
Animal* animal1 = new Dog();
Animal* animal2 = new Cat();
animal1->makeSound(); // output: Dog barks.
animal2->makeSound(); // output: Cat meows.
delete animal1;
delete animal2;
return 0;
}
```
3. 多态性和抽象类:抽象类是指包含至少一个纯虚函数的类,不能实例化对象,只能作为其他类的基类。如果我们要实现一个接口类,可以将其定义为一个抽象类,然后派生出不同的类来实现其纯虚函数。在使用指向派生类的基类指针时,如果将基类定义为抽象类,那么派生类必须实现其纯虚函数,否则编译器将会报错。
```c++
class Shape {
public:
virtual double getArea() = 0; // 纯虚函数,必须在派生类中实现
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double getArea() { return 3.14 * radius * radius; }
};
class Rectangle : public Shape {
private:
double width, height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double getArea() { return width * height; }
};
int main() {
Shape* shape1 = new Circle(5);
Shape* shape2 = new Rectangle(3, 4);
cout << "Circle area: " << shape1->getArea() << endl; // output: Circle area: 78.5
cout << "Rectangle area: " << shape2->getArea() << endl; // output: Rectangle area: 12
delete shape1;
delete shape2;
return 0;
}
```
阅读全文
相关推荐
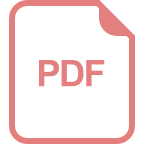
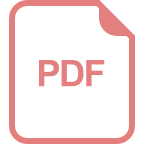
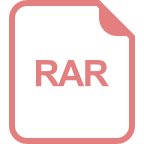
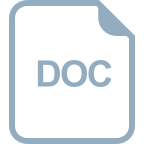





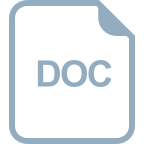
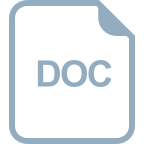
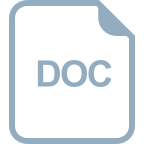


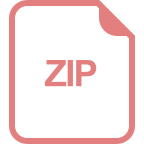
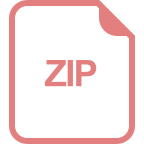