好的,我可以为您解答这个问题。处理分页通常需要以下步骤: 定义每一页的大小 在React中,您可以使用state来定义每一页的大小。例如: 复制 this.state = { pageSize: 10 // 每页显示10个数据 } 计算总页数 根据数据的总数和每页大小,可以计算出总页数。例如: 复制 const { data } = this.props; const { pageSize } = this.state; const totalPages = Math.ceil(data.length / pageSize); 定义当前页码 使用state来定义当前页码。例如: 复制 this.state = { currentPage: 1 // 默认显示第一页 } 根据当前页码和每页大小,计算出要显示的数据 使用slice方法根据当前页码和每页大小来计算要显示的数据。例如: 复制 const { data } = this.props; const { currentPage, pageSize } = this.state; const startIndex = (currentPage - 1) * pageSize; const endIndex = startIndex + pageSize; const currentData = data.slice(startIndex, endIndex); 显示分页组件 使用React组件来显示分页组件,并且在用户点击页码时更新当前页码。例如: 复制 render() { const { data } = this.props; const { currentPage, pageSize } = this.state; const startIndex = (currentPage - 1) * pageSize; const endIndex = startIndex + pageSize; const currentData = data.slice(startIndex, endIndex); const totalPages = Math.ceil(data.length / pageSize); return ( <div> {/* 显示当前页的数据 /} {currentData.map(item => ( <div key={item.id}>{item.name}</div> ))} {/ 显示分页按钮 */} {Array.from({ length: totalPages }).map((_, index) => ( <button key={index} onClick={() => this.setState({ currentPage: index + 1 })} disabled={index + 1 === currentPage} > {index + 1} </button> ))} </div> ); } 合成完整代码
时间: 2024-03-01 17:55:09 浏览: 75
好的,以下是完整的代码示例:
```
import React, { Component } from "react";
class Pagination extends Component {
constructor(props) {
super(props);
this.state = {
currentPage: 1,
pageSize: 10,
};
}
render() {
const { data } = this.props;
const { currentPage, pageSize } = this.state;
const startIndex = (currentPage - 1) * pageSize;
const endIndex = startIndex + pageSize;
const currentData = data.slice(startIndex, endIndex);
const totalPages = Math.ceil(data.length / pageSize);
return (
<div>
{/* 显示当前页的数据 */}
{currentData.map((item) => (
<div key={item.id}>{item.name}</div>
))}
{/* 显示分页按钮 */}
{Array.from({ length: totalPages }).map((_, index) => (
<button
key={index}
onClick={() => this.setState({ currentPage: index + 1 })}
disabled={index + 1 === currentPage}
>
{index + 1}
</button>
))}
</div>
);
}
}
export default Pagination;
```
这个组件可以接受`data`属性,表示要分页的数据。您可以在需要使用分页的地方引入这个组件,例如:
```
import React from "react";
import Pagination from "./Pagination";
const data = [
{ id: 1, name: "A" },
{ id: 2, name: "B" },
{ id: 3, name: "C" },
{ id: 4, name: "D" },
{ id: 5, name: "E" },
{ id: 6, name: "F" },
{ id: 7, name: "G" },
{ id: 8, name: "H" },
{ id: 9, name: "I" },
{ id: 10, name: "J" },
{ id: 11, name: "K" },
{ id: 12, name: "L" },
{ id: 13, name: "M" },
{ id: 14, name: "N" },
{ id: 15, name: "O" },
];
function App() {
return <Pagination data={data} />;
}
export default App;
```
这样就可以在页面上显示分页组件了。希望这个回答能够帮到您。
阅读全文
相关推荐



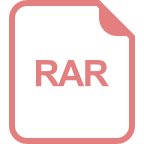















