pthread_mutex_lock函数
时间: 2023-10-13 10:15:45 浏览: 35
pthread_mutex_lock函数是一个用于线程同步的函数,它的作用是获取一个互斥锁。互斥锁是一种常用的线程同步机制,用来保护共享资源,避免多个线程同时访问同一资源而导致的数据竞争。
pthread_mutex_lock函数的原型如下:
```c
int pthread_mutex_lock(pthread_mutex_t *mutex);
```
其中,mutex是一个指向互斥锁的指针。
pthread_mutex_lock函数的作用是获取互斥锁,如果当前互斥锁已经被其他线程占用,则调用线程会被阻塞,直到互斥锁被释放为止。当函数成功获取互斥锁时,返回值为0;否则,返回一个非零错误码。
需要注意的是,pthread_mutex_lock函数获取互斥锁后,需要在适当的时候释放互斥锁,以允许其他线程获取锁并访问共享资源。可以使用pthread_mutex_unlock函数来释放互斥锁。
相关问题
pthread_mutex_lock 函数怎么用
`pthread_mutex_lock` 是一个线程同步的函数,用于锁定互斥量(mutex),保证同一时刻只有一个线程能够访问共享资源。
函数原型如下:
```c
#include <pthread.h>
int pthread_mutex_lock(pthread_mutex_t *mutex);
```
参数 `mutex` 是一个指向需要加锁的互斥量的指针。
函数返回值为 0 表示成功加锁,否则返回错误码。
下面是一个简单的例子,使用 `pthread_mutex_lock` 来保证线程同步:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
void *thread_func(void *arg) {
int *p = (int *)arg;
pthread_mutex_lock(&mutex);
printf("Thread %d is running...\n", *p);
pthread_mutex_unlock(&mutex);
return NULL;
}
int main() {
int i;
pthread_t tid[5];
for (i = 0; i < 5; ++i) {
pthread_create(&tid[i], NULL, thread_func, &i);
}
for (i = 0; i < 5; ++i) {
pthread_join(tid[i], NULL);
}
return 0;
}
```
在上面的例子中,我们创建了 5 个线程,它们都会执行 `thread_func` 函数。由于我们使用了互斥量来保证同一时刻只有一个线程能够访问共享资源,因此每个线程输出的时候都会先加锁,然后再输出,最后再解锁。这样就能够保证线程同步,避免了竞争条件的问题。
举例说明pthread_mutex_lock函数的使用方法
pthread_mutex_lock函数是Linux线程库中的一个函数,用于对互斥锁进行加锁操作。下面是一个简单的使用示例:
```c
#include <stdio.h>
#include <pthread.h>
pthread_mutex_t mutex;
void *thread_func(void *arg) {
pthread_mutex_lock(&mutex);
printf("Thread locked\n");
// Do some work...
pthread_mutex_unlock(&mutex);
printf("Thread unlocked\n");
return NULL;
}
int main() {
pthread_t thread;
pthread_mutex_init(&mutex, NULL);
pthread_create(&thread, NULL, thread_func, NULL);
// Do some other work...
pthread_join(thread, NULL);
pthread_mutex_destroy(&mutex);
return 0;
}
```
在上面的代码中,我们首先定义了一个互斥锁`mutex`,然后创建了一个线程`thread`,并在该线程中对互斥锁进行加锁操作。主线程在创建子线程后也做了一些其他的工作,最后使用`pthread_join`函数等待子线程结束。在主线程结束前,我们还需要使用`pthread_mutex_destroy`函数销毁互斥锁。
需要注意的是,`pthread_mutex_lock`函数可能会阻塞当前线程,直到该互斥锁被解锁。因此,在使用互斥锁时,我们需要注意避免死锁的情况。为了避免死锁,我们需要在对多个互斥锁进行加锁操作时,按照相同的顺序进行加锁,以避免出现互相等待的情况。
相关推荐
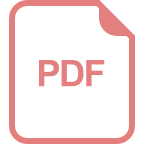
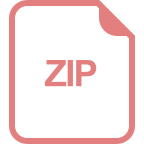
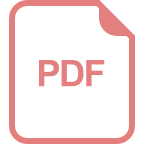












