java增量备份mysql数据库到指定位置
时间: 2023-08-05 15:11:43 浏览: 52
要实现Java增量备份MySQL数据库到指定位置,可以使用以下步骤:
1. 首先需要连接MySQL数据库,可以使用JDBC来实现。需要在代码中导入mysql-connector-java依赖包,并使用以下代码获取数据库连接:
```
String url = "jdbc:mysql://localhost:3306/db_name";
String user = "username";
String password = "password";
Connection conn = DriverManager.getConnection(url, user, password);
```
2. 接下来,需要获取需要备份的表的最后备份时间。可以在备份时将备份时间保存在一个配置文件中,然后在备份时读取该配置文件获取最后备份时间。如果是第一次备份,则将最后备份时间设为初始时间。
3. 查询需要备份的表数据时,只查询最后备份时间之后更新的数据,可以使用以下SQL语句:
```
SELECT * FROM table_name WHERE update_time > last_backup_time;
```
其中,table_name为需要备份的表名,update_time为表中记录的更新时间,last_backup_time为上一次备份的时间。
4. 将查询到的数据写入到备份文件中。可以使用Java的IO流来实现,写入到指定位置即可。
完整代码如下:
```
import java.io.*;
import java.sql.*;
public class MySQLBackup {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/db_name";
String user = "username";
String password = "password";
String backupPath = "path/to/backup/file";
String tableName = "table_name";
String backupTime = "2021-01-01 00:00:00"; // 初始备份时间
try {
Connection conn = DriverManager.getConnection(url, user, password);
// 获取最后备份时间
File configFile = new File("config.properties");
if (configFile.exists()) {
Properties prop = new Properties();
prop.load(new FileInputStream(configFile));
backupTime = prop.getProperty("last_backup_time");
}
// 查询需要备份的数据
String sql = "SELECT * FROM " + tableName + " WHERE update_time > ?";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, backupTime);
ResultSet rs = pstmt.executeQuery();
// 写入备份文件
File backupFile = new File(backupPath);
if (!backupFile.exists()) {
backupFile.createNewFile();
}
BufferedWriter writer = new BufferedWriter(new FileWriter(backupFile));
while (rs.next()) {
// 将数据写入到文件中
}
writer.close();
// 更新最后备份时间
Properties prop = new Properties();
prop.setProperty("last_backup_time", getCurrentTime());
prop.store(new FileOutputStream(configFile), null);
conn.close();
} catch (Exception e) {
e.printStackTrace();
}
}
private static String getCurrentTime() {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
return sdf.format(new Date());
}
}
```
在代码中,需要将db_name、username、password、path/to/backup/file和table_name替换为实际的值。可以将上述代码保存为Java文件,然后使用编译器或命令行工具进行编译和执行。
相关推荐
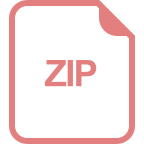














