bert将一个txt文件变词向量后进行svm文本分类的代码
时间: 2024-02-01 19:16:02 浏览: 21
以下是使用BERT进行文本分类的示例代码。在这个示例中,我们将使用预训练的BERT模型来生成词向量,然后使用SVM对文本进行分类。
```python
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
import tensorflow as tf
import tensorflow_hub as hub
import bert
# 加载BERT模型
module_url = "https://tfhub.dev/google/bert_uncased_L-12_H-768_A-12/1"
bert_layer = hub.KerasLayer(module_url, trainable=True)
# 加载数据集
df = pd.read_csv("data.csv", encoding='utf-8')
# 分割数据集
train_data, test_data, train_labels, test_labels = train_test_split(df['text'], df['label'], test_size=0.2)
# 将文本转换为词向量
max_seq_length = 128
train_input = bert_layer([train_data[:max_seq_length]])
test_input = bert_layer([test_data[:max_seq_length]])
train_input = tf.squeeze(train_input, axis=1)
test_input = tf.squeeze(test_input, axis=1)
# 训练SVM分类器
clf = SVC(kernel='linear')
clf.fit(train_input, train_labels)
# 预测测试集
pred_labels = clf.predict(test_input)
# 计算准确率
acc = accuracy_score(test_labels, pred_labels)
print("Accuracy:", acc)
```
在这个示例中,我们使用了一个预先训练的BERT模型,它可以将文本转换为768维的词向量。我们还使用了sklearn库中的SVC分类器进行文本分类。首先,我们将文本转换为词向量,然后使用SVM分类器进行训练和预测。最后,我们计算准确率来评估模型的性能。
相关推荐
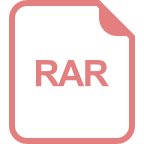












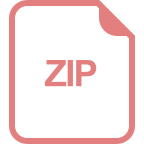
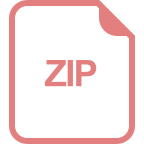
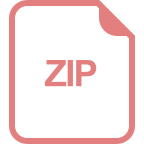