用bert将一个csv文件分为词向量后进行svm分类
时间: 2024-01-31 20:04:03 浏览: 26
可以用Python中的scikit-learn库来实现这个任务。以下是一些大致的步骤:
1. 读取csv文件,并将文本数据和标签分别存储在两个列表中。
2. 使用BERT模型对文本数据进行编码,得到词向量矩阵。
3. 将词向量矩阵和标签列表传入SVM分类器进行训练。
4. 对测试集进行预测,并计算模型的准确率、精确率、召回率等性能指标。
以下是一份示例代码:
```python
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from transformers import BertTokenizer, BertModel
# 读取csv文件
data = pd.read_csv('data.csv')
# 将文本数据和标签分别存储在两个列表中
sentences = data['text'].tolist()
labels = data['label'].tolist()
# 加载BERT模型和分词器
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
model = BertModel.from_pretrained('bert-base-uncased')
# 对文本数据进行编码,得到词向量矩阵
input_ids = []
attention_masks = []
for sent in sentences:
encoded_dict = tokenizer.encode_plus(sent, add_special_tokens=True,
max_length=64, pad_to_max_length=True,
return_attention_mask=True, return_tensors='pt')
input_ids.append(encoded_dict['input_ids'])
attention_masks.append(encoded_dict['attention_mask'])
input_ids = torch.cat(input_ids, dim=0)
attention_masks = torch.cat(attention_masks, dim=0)
with torch.no_grad():
last_hidden_states = model(input_ids, attention_mask=attention_masks)
features = last_hidden_states[0][:,0,:].numpy()
# 将数据分为训练集和测试集
train_features, test_features, train_labels, test_labels = train_test_split(features, labels)
# 训练SVM分类器
clf = SVC(kernel='linear')
clf.fit(train_features, train_labels)
# 在测试集上评估模型性能
pred_labels = clf.predict(test_features)
accuracy = np.mean(pred_labels == test_labels)
precision = precision_score(test_labels, pred_labels)
recall = recall_score(test_labels, pred_labels)
f1 = f1_score(test_labels, pred_labels)
```
需要注意的是,这份代码中使用的是BERT的CLS向量作为句子的表示,因此在对数据进行编码时,需要对每个句子添加特殊的`[CLS]`和`[SEP]`标记。如果需要使用BERT的其他表示方式,可以参考Hugging Face的官方文档进行修改。
相关推荐
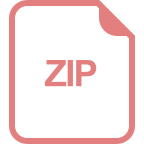














