bert将一个txt文件变词向量的代码
时间: 2024-01-21 13:05:52 浏览: 68
下面是使用bert-as-service包将一个txt文件转换为词向量的Python代码:
```python
import os
import numpy as np
from bert_serving.client import BertClient
# 创建BertClient实例
bc = BertClient()
# 读取文本文件
with open('input.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 将文本分割成句子
sentences = text.split('\n')
# 将每个句子转换为词向量并存储
vectors = []
for sentence in sentences:
# 使用BertClient获取句子的词向量
vector = bc.encode([sentence])[0]
vectors.append(vector)
# 将词向量保存到文件
np.savetxt('output.txt', vectors)
```
在上面的代码中,我们使用BertClient从文本文件中读取句子,并将每个句子转换为词向量。然后,我们将所有词向量存储在一个矩阵中,并将该矩阵保存到文件中。请注意,BertClient可以处理多个句子,因此我们可以一次性将整个文本文件转换为词向量。
相关问题
bert将一个txt文件变词向量后进行传统文本分类的代码
以下是使用BERT模型进行传统文本分类的Python代码示例:
```python
import torch
from transformers import BertTokenizer, BertForSequenceClassification
# 加载预训练的BERT模型
model = BertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
# 加载文本数据
with open('text_file.txt', 'r') as f:
texts = f.readlines()
# 将文本转换为词向量
input_ids = []
attention_masks = []
for text in texts:
encoded_dict = tokenizer.encode_plus(
text,
add_special_tokens=True,
max_length=128,
pad_to_max_length=True,
return_attention_mask=True,
return_tensors='pt'
)
input_ids.append(encoded_dict['input_ids'])
attention_masks.append(encoded_dict['attention_mask'])
input_ids = torch.cat(input_ids, dim=0)
attention_masks = torch.cat(attention_masks, dim=0)
# 运行BERT模型进行文本分类
with torch.no_grad():
outputs = model(input_ids, attention_mask=attention_masks)
logits = outputs[0]
# 输出分类结果
predictions = torch.argmax(logits, dim=-1)
print(predictions)
```
在以上代码中,我们首先加载了预训练的BERT模型和对应的Tokenizer,然后加载了待分类的文本数据。接下来,使用Tokenizer将文本转换为词向量,并将所有文本的词向量拼接在一起作为输入。最后,我们运行BERT模型,得到分类结果。
bert将一个txt文件变词向量后进行svm文本分类的代码
以下是使用BERT进行文本分类的代码,其中包括将文本转换为BERT词向量和使用SVM进行分类的步骤:
```python
import numpy as np
from sklearn.svm import SVC
from transformers import BertTokenizer, BertModel
# 加载BERT模型和tokenizer
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
model = BertModel.from_pretrained('bert-base-uncased', output_hidden_states=True)
# 加载数据集
with open('data.txt', 'r') as f:
data = f.readlines()
# 将文本转换为BERT词向量
def bert_embedding(text):
input_ids = torch.tensor(tokenizer.encode(text)).unsqueeze(0)
with torch.no_grad():
outputs = model(input_ids)
embeddings = outputs[2][-1].squeeze().numpy()
return embeddings
# 将所有文本转换为BERT词向量
X = []
for text in data:
embeddings = bert_embedding(text)
X.append(embeddings)
X = np.array(X)
# 加载标签
with open('labels.txt', 'r') as f:
labels = f.readlines()
# 将标签转换为数字
y = [int(label.strip()) for label in labels]
# 划分训练集和测试集
train_size = int(0.8 * len(X))
train_X, test_X = X[:train_size], X[train_size:]
train_y, test_y = y[:train_size], y[train_size:]
# 使用SVM进行分类
clf = SVC(kernel='linear')
clf.fit(train_X, train_y)
print('Accuracy:', clf.score(test_X, test_y))
```
在上面的代码中,我们首先加载了BERT模型和tokenizer,然后使用`bert_embedding`函数将所有文本转换为BERT词向量。接下来,我们将标签转换为数字,并将数据集划分为训练集和测试集。最后,我们使用SVM进行分类,并打印出测试集上的准确率。
阅读全文
相关推荐
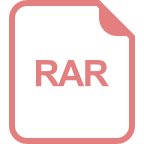
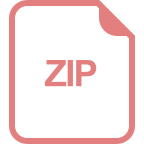
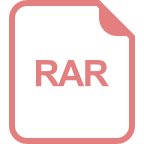
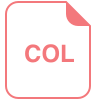





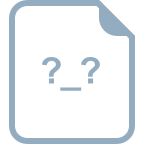





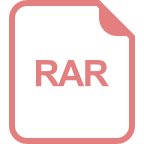
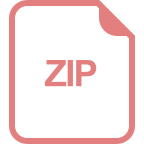