uniapp刷新子组件
时间: 2023-09-27 18:04:28 浏览: 79
在uniapp中,可以使用$refs来获取子组件的实例,然后调用子组件的方法来刷新子组件。
下面是一个示例代码:
// 父组件
<template>
<div>
<child ref="child"></child>
<button @click="refreshChild">刷新子组件</button>
</div>
</template>
<script>
import child from './components/child.vue'
export default {
components: {
child
},
methods: {
refreshChild() {
this.$refs.child.refresh()
}
}
}
</script>
// 子组件
<template>
<div>{{ message }}</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello World'
}
},
methods: {
refresh() {
// 刷新操作
}
}
}
</script>
在父组件中,我们使用$refs来获取子组件的实例,并调用子组件的refresh方法来刷新子组件。在子组件中,我们定义了一个refresh方法,用于执行刷新操作。
相关问题
uniapp 刷新子组件的list
如果您想在 uni-app 中刷新子组件的 list,可以使用以下步骤:
1. 在父组件中使用 props 将 list 数据传递给子组件。
2. 在子组件中使用 props 接收 list 数据,并将其存储在子组件的 data 中。
3. 在子组件中使用 watch 监听 list 数据的变化,并在数据变化时更新子组件的 list。
示例代码如下:
父组件:
```html
<template>
<div>
<child :list="list"></child>
<button @click="refreshList">刷新列表</button>
</div>
</template>
<script>
import child from './child.vue'
export default {
components: {
child
},
data () {
return {
list: [/* 初始数据 */]
}
},
methods: {
refreshList () {
// 模拟刷新数据
this.list = [/* 新的数据 */]
}
}
}
</script>
```
子组件:
```html
<template>
<div>
<ul>
<li v-for="item in itemList" :key="item.id">{{ item.name }}</li>
</ul>
</div>
</template>
<script>
export default {
props: ['list'],
data () {
return {
itemList: []
}
},
watch: {
list () {
this.itemList = this.list // 更新子组件的 list
}
}
}
</script>
```
当父组件中的 list 数据发生变化时,子组件的 watch 监听函数会自动触发,更新子组件的 itemList,从而实现了刷新子组件的 list 的效果。
uniapp 强制刷新子组件
在 uniapp 中,可以使用 $emit 在父组件和子组件之间进行通信。
子组件可以通过调用 $emit 方法来向父组件发送消息,父组件可以监听子组件的 $emit 触发的事件并执行相应的操作。
在父组件中,可以在子组件的标签上绑定事件监听器来监听子组件的 $emit 触发的事件,例如:
```
<template>
<child-component @refresh="refreshChild"></child-component>
</template>
<script>
export default {
methods: {
refreshChild() {
// 在这里执行刷新子组件的操作
}
}
}
</script>
```
在子组件中,可以通过调用 $emit 方法来触发事件,例如:
```
export default {
methods: {
refresh() {
// 在这里执行刷新操作
this.$emit('refresh')
}
}
}
```
当调用子组件的 refresh 方法时,就会触发父组件的 refreshChild 方法,从而实现了子组件的刷新。
相关推荐
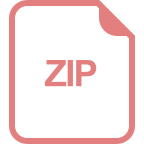












