java代码实现一个 SocketUtil,实现1个接口:readFromStream 输入参数为socket的OutputStream,进行一次数据读取,第一个是占6个字节的HEAD,内容为常量"BTVRV1",当匹配时,读取第二个参数,占2个字节,为数据包类型,第三个为数据长度占4个字节,需要从小端转为大端,转化为int,第四个为data负载,为数据长度大小的byte,第5个参数为CRC值,占4个字节,也需要小端转大端为int 当HEAD不匹配时,就将数据拼接到缓冲区中,等待下一次读取。当缓冲区的数据拼接后包含“BTRV1”时,服务器即可开始解析报文,使用bytebuffer
时间: 2023-05-16 21:05:21 浏览: 113
以下是一个可能的实现:
```java
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.ByteBuffer;
public class SocketUtil {
public static final String HEAD = "BTVRV1";
public static void readFromStream(OutputStream out) throws IOException {
byte[] headBytes = new byte[6];
int bytesRead = readFully(out, headBytes);
if (bytesRead != 6 || !HEAD.equals(new String(headBytes))) {
// Head not matched, wait for next read
return;
}
byte[] typeBytes = new byte[2];
readFully(out, typeBytes);
byte[] lengthBytes = new byte[4];
readFully(out, lengthBytes);
int length = Integer.reverseBytes(ByteBuffer.wrap(lengthBytes).getInt());
byte[] dataBytes = new byte[length];
readFully(out, dataBytes);
byte[] crcBytes = new byte[4];
readFully(out, crcBytes);
int crc = Integer.reverseBytes(ByteBuffer.wrap(crcBytes).getInt());
// Process the data here
// ...
// Clear the buffer
out.flush();
}
private static int readFully(OutputStream out, byte[] buffer) throws IOException {
int bytesRead = 0;
while (bytesRead < buffer.length) {
int count = out.write(buffer, bytesRead, buffer.length - bytesRead);
if (count < 0) {
throw new IOException("End of stream");
}
bytesRead += count;
}
return bytesRead;
}
}
```
这个类实现了一个静态方法 `readFromStream`,它接收一个 `OutputStream` 参数,从中读取一次数据。如果读取到的数据的头部匹配常量 `HEAD`,则解析出数据包类型、数据长度、数据负载和 CRC 值,并进行相应的处理。如果头部不匹配,则将数据拼接到缓冲区中,等待下一次读取。在处理完数据后,需要清空缓冲区,以便下一次读取。
这个实现使用了 Java 的标准库中的 `ByteBuffer` 类来进行字节序转换。它还使用了一个辅助方法 `readFully`,它从 `OutputStream` 中读取指定长度的字节,并确保读取到指定长度的字节。如果读取到的字节数少于指定长度,则抛出一个 `IOException` 异常。
阅读全文
相关推荐
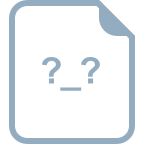
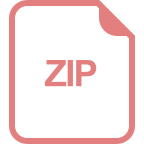
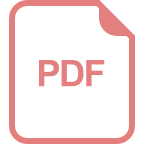
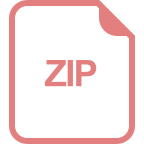
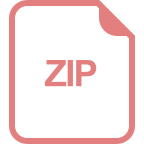
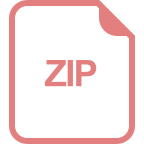
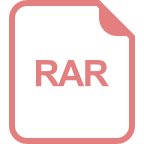
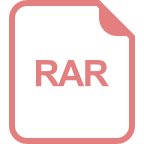
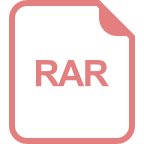
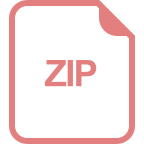
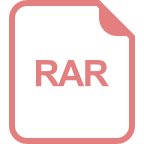




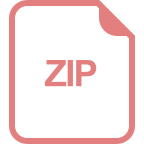
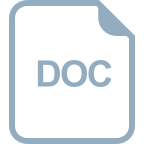
