java代码如何实现把一个超大文件分成多个小文件
时间: 2024-02-17 22:04:37 浏览: 135
可以使用Java的IO流和缓冲区来实现把一个超大文件分成多个小文件。以下是一个示例代码:
```java
import java.io.*;
public class LargeFileSplitter {
public static void main(String[] args) {
String filePath = "path/to/large/file";
int bufferSize = 8192; // 缓冲区大小
int fileCount = 10; // 分割后的文件个数
long fileSize = new File(filePath).length(); // 获取文件大小
long blockSize = fileSize / fileCount; // 计算每个文件块的大小
try (InputStream inputStream = new BufferedInputStream(new FileInputStream(filePath), bufferSize)) {
byte[] buffer = new byte[bufferSize];
int bytesRead = 0;
int fileIndex = 1;
long bytesWritten = 0;
OutputStream outputStream = new BufferedOutputStream(new FileOutputStream(filePath + ".part" + fileIndex));
while ((bytesRead = inputStream.read(buffer)) != -1) {
if (bytesWritten + bytesRead > blockSize) {
// 如果当前块大小已经达到限制,则关闭当前块,并创建新的块
outputStream.close();
fileIndex++;
if (fileIndex <= fileCount) {
outputStream = new BufferedOutputStream(new FileOutputStream(filePath + ".part" + fileIndex));
}
bytesWritten = 0;
}
outputStream.write(buffer, 0, bytesRead);
bytesWritten += bytesRead;
}
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上述代码中,我们首先获取待分割文件的大小,然后计算出每个文件块的大小。我们使用while循环来不断读取数据,并将数据写入到当前正在处理的文件块中。如果当前块大小已经达到限制,则关闭当前块,并创建新的块。最终我们得到了多个小文件,文件名为原文件名加上.part和分块编号。可以根据实际需求对分割后的文件进行处理,例如上传到云存储或者其他文件处理操作。
阅读全文
相关推荐






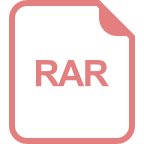











