java代码实现分卷压缩成多个文件
时间: 2023-03-02 07:50:09 浏览: 469
您可以使用 Java 中的 ZipOutputStream 类来实现分卷压缩成多个文件的功能。以下是一个示例代码,用于将一个大的文件分成多个大小相等的文件进行压缩:
```java
import java.io.*;
import java.util.zip.*;
public class ZipSplitter {
public static void splitAndZip(String fileName, int chunkSize) throws IOException {
byte[] buffer = new byte[1024];
// Create a ZipOutputStream object for the output file
ZipOutputStream out = new ZipOutputStream(new FileOutputStream(fileName + ".zip"));
// Read the input file and split it into chunks
FileInputStream in = new FileInputStream(fileName);
int partCount = 0;
while (true) {
// Create a new ZipEntry for the chunk
ZipEntry entry = new ZipEntry(fileName + ".z" + partCount);
out.putNextEntry(entry);
// Write the chunk to the output file
int count = in.read(buffer);
if (count == -1) {
break;
}
out.write(buffer, 0, count);
// Close the entry
out.closeEntry();
// If the chunk is larger than the specified chunk size, split it into smaller chunks
if (out.getBytesWritten() > chunkSize) {
out.finish();
out.close();
out = new ZipOutputStream(new FileOutputStream(fileName + ".z" + ++partCount + ".zip"));
}
}
// Close the input and output streams
in.close();
out.finish();
out.close();
}
public static void main(String[] args) throws IOException {
// Split and zip the file "largefile.txt" into chunks of size 1 MB
splitAndZip("largefile.txt", 1024 * 1024);
}
}
```
这段代码将输入文件 "largefile.txt" 分成大小为 1 MB 的多个文件,并将它们压缩成多个 zip 文件(例如 "largefile.txt.z0.zip"、"largefile.txt.z1.zip"、"largefile.txt.z2.zip" 等)。您可以根据需要调整文件大小和文件名的格式。
阅读全文
相关推荐
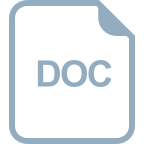
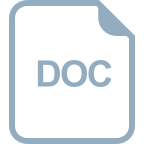
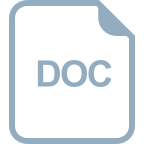






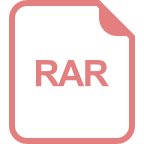
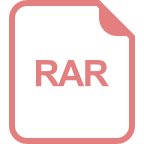
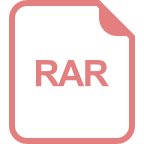
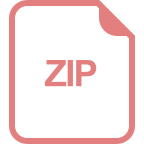
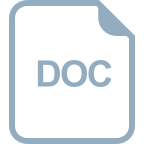
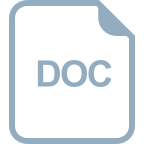


