ZipSplitReadOnlySeekableByteChannel创建分卷压缩代码示例
时间: 2023-09-11 13:05:10 浏览: 231
### 回答1:
ZipSplitReadOnlySeekableByteChannel是Java NIO库中的一个接口,可以用于读取已分卷压缩的ZIP文件。下面是一个示例代码,演示如何使用ZipSplitReadOnlySeekableByteChannel创建分卷压缩的ZIP文件:
```java
import java.io.*;
import java.nio.channels.*;
import java.nio.file.*;
import java.util.zip.*;
public class ZipSplitExample {
public static void main(String[] args) throws IOException {
// 设置要创建的 ZIP 文件的路径和名称
Path zipFilePath = Paths.get("example.zip");
// 打开一个 ZipSplitReadOnlySeekableByteChannel 以写入文件
ZipSplitReadOnlySeekableByteChannel zipChannel = ZipSplitReadOnlySeekableByteChannel
.open(zipFilePath, StandardOpenOption.CREATE, StandardOpenOption.WRITE);
// 创建一个新的 ZIP 文件系统,并获取其根目录
FileSystem zipFileSystem = FileSystems.newFileSystem(zipFilePath, null);
Path root = zipFileSystem.getPath("/");
// 创建一个新的 ZIP 文件,并将其压缩为两个分卷
int segmentSize = 1024 * 1024 * 10; // 10 MB per segment
ZipOutputStream zipOutputStream = new ZipOutputStream(
Channels.newOutputStream(zipChannel.newOutputStream(0, segmentSize)));
zipOutputStream.setLevel(Deflater.DEFAULT_COMPRESSION);
// 添加要压缩的文件或目录
Path fileToZip = Paths.get("example.txt");
Path zipEntryPath = zipFileSystem.getPath("/example.txt");
zipOutputStream.putNextEntry(new ZipEntry(zipEntryPath.toString()));
Files.copy(fileToZip, zipOutputStream);
zipOutputStream.closeEntry();
// 关闭输出流和 ZIP 文件系统
zipOutputStream.close();
zipFileSystem.close();
// 打印已创建的 ZIP 文件的路径
System.out.println("ZIP file created: " + zipFilePath);
}
}
```
在这个示例代码中,我们首先设置要创建的 ZIP 文件的路径和名称,然后使用ZipSplitReadOnlySeekableByteChannel打开一个通道以写入文件。接着,我们创建一个新的ZIP文件系统,并获取其根目录。我们将创建一个新的ZIP文件,并将其压缩为两个分卷,每个分卷的大小为10MB。然后我们将要压缩的文件或目录添加到ZIP文件中,并关闭输出流和ZIP文件系统。最后,我们打印已创建的ZIP文件的路径。
请注意,如果您想要读取已分卷压缩的ZIP文件,可以使用ZipSplitReadOnlySeekableByteChannel打开一个通道以读取文件,类似于上面的代码中使用ZipSplitReadOnlySeekableByteChannel打开通道以写入文件。
### 回答2:
ZipSplitReadOnlySeekableByteChannel是Java的一个类,用于创建分卷压缩的代码示例可以如下:
import java.io.IOException;
import java.io.RandomAccessFile;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.channels.SeekableByteChannel;
import java.nio.file.Path;
import java.nio.file.StandardOpenOption;
import java.util.zip.ZipException;
public class ZipSplitExample {
public static void main(String[] args) {
try {
Path zipPath = Path.of("example.zip"); // 压缩文件路径
String splitZipName = "split.zip"; // 分卷压缩文件名
long maxSplitSize = 10 * 1024 * 1024; // 每个分卷的最大大小(字节)
// 打开原始的压缩文件
SeekableByteChannel zipChannel = FileChannel.open(zipPath, StandardOpenOption.READ);
// 创建分卷压缩文件
SeekableByteChannel splitChannel = new ZipSplitReadOnlySeekableByteChannel(zipChannel, splitZipName, maxSplitSize);
// 使用分卷压缩文件进行读取或操作
ByteBuffer buffer = ByteBuffer.allocate(1024);
while (splitChannel.read(buffer) != -1) {
buffer.flip();
// 对分卷压缩文件进行操作
// ...
buffer.clear();
}
// 关闭通道
splitChannel.close();
zipChannel.close();
} catch (ZipException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
上述代码首先定义了压缩文件的路径(`zipPath`)、分卷压缩文件的名称(`splitZipName`)和每个分卷的最大大小(`maxSplitSize`)。然后,通过`FileChannel.open`方法打开原始的压缩文件,得到一个`SeekableByteChannel`对象`zipChannel`。
之后,创建一个`ZipSplitReadOnlySeekableByteChannel`对象`splitChannel`,通过传入`zipChannel`、`splitZipName`和`maxSplitSize`参数来创建分卷压缩文件。然后,使用`splitChannel`对象进行读取或操作分卷压缩文件。
最后,关闭通道,释放资源。
以上代码示例了如何使用`ZipSplitReadOnlySeekableByteChannel`类创建分卷压缩的代码。
### 回答3:
ZipSplitReadOnlySeekableByteChannel是一个类,用于创建和管理分卷压缩文件的读取通道。它可以将一个大型压缩文件拆分成多个较小的分卷压缩文件,并提供按需读取这些文件的功能。
以下是一个示例代码,演示了使用ZipSplitReadOnlySeekableByteChannel创建分卷压缩文件的过程:
```java
import java.io.IOException;
import java.nio.channels.*;
public class ZipSplitExample {
public static void main(String[] args) {
String sourceFile = "path/to/large.zip";
String destFolder = "path/to/dest/folder";
int volumeSize = 100 * (1024 * 1024); // 100MB
try (SeekableByteChannel sourceChannel = Files.newByteChannel(Paths.get(sourceFile));
ZipSplitReadOnlySeekableByteChannel zipSplitChannel = new ZipSplitReadOnlySeekableByteChannel(sourceChannel, volumeSize);) {
// 逐个分卷读取文件并处理
int volumeIndex = 0;
while (zipSplitChannel.hasNextVolume()) {
SeekableByteChannel volumeChannel = zipSplitChannel.nextVolume();
String volumeFileName = String.format("volume-%03d.zip", volumeIndex);
Path volumeFilePath = Paths.get(destFolder, volumeFileName);
// 保存分卷文件到目标文件夹
try (FileChannel destChannel = FileChannel.open(volumeFilePath, StandardOpenOption.CREATE_NEW, StandardOpenOption.WRITE)) {
destChannel.transferFrom(volumeChannel, 0, volumeChannel.size());
}
// 处理分卷文件
// TODO: Add your code here
volumeIndex++;
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们首先创建了一个ZipSplitReadOnlySeekableByteChannel实例,传入源文件的通道和分卷大小。接下来,我们通过调用nextVolume()方法来逐个获取分卷文件的通道,并保存到目标文件夹中。然后,我们可以根据需要对每个分卷文件进行任何处理。
请注意,示例中的"path/to/large.zip"是源文件的路径,"path/to/dest/folder"是保存分卷文件的目标文件夹的路径,volumeSize是每个分卷文件的大小,通过字节数表示。
阅读全文
相关推荐
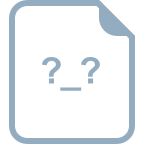
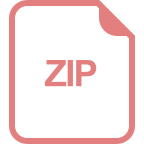
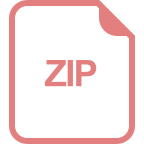
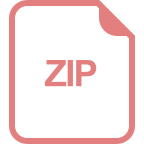
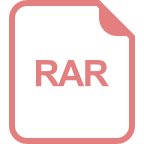
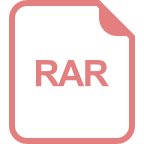
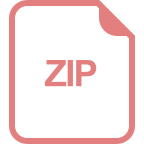
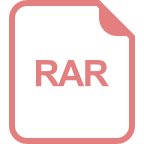
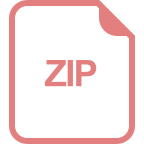
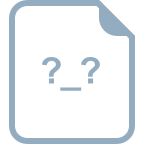
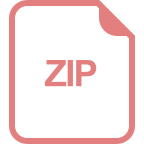
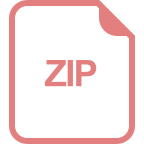
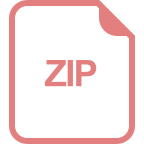
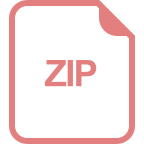
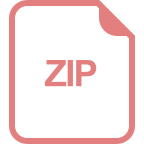
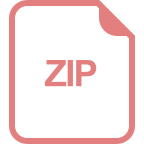
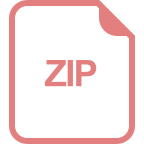
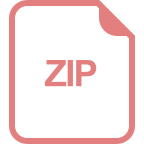