请用c语言实现,并写出代码:试编写双栈初始化,判断栈空、栈满、进栈和出栈等算法的函数。“
时间: 2023-05-21 21:05:23 浏览: 246
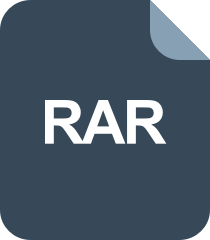
shunxubiao.rar_栈的顺序表_顺序栈,出栈
好的,下面是代码:
```c
#include <stdio.h>
#define MAXSIZE 100
typedef struct {
int top1; // 栈1的栈顶指针
int top2; // 栈2的栈顶指针
int data[MAXSIZE]; // 共用数组空间
}DoubleStack;
void InitDoubleStack(DoubleStack *S) { // 双栈初始化
S->top1 = -1;
S->top2 = MAXSIZE;
}
int IsEmptyStack1(DoubleStack S) { // 判断栈1是否为空
if (S.top1 == -1) {
return 1;
} else {
return 0;
}
}
int IsEmptyStack2(DoubleStack S) { // 判断栈2是否为空
if (S.top2 == MAXSIZE) {
return 1;
} else {
return 0;
}
}
int IsFull(DoubleStack S) { // 判断栈满
if (S.top1 + 1 == S.top2) {
return 1;
} else {
return 0;
}
}
void PushStack1(DoubleStack *S, int x) { // 进栈1
if (IsFull(*S)) {
printf("Stack is full.\n");
} else {
S->data[++S->top1] = x;
}
}
void PushStack2(DoubleStack *S, int x) { // 进栈2
if (IsFull(*S)) {
printf("Stack is full.\n");
} else {
S->data[--S->top2] = x;
}
}
int PopStack1(DoubleStack *S) { // 出栈1
if (IsEmptyStack1(*S)) {
printf("Stack is empty.\n");
return -1;
} else {
return S->data[S->top1--];
}
}
int PopStack2(DoubleStack *S) { // 出栈2
if (IsEmptyStack2(*S)) {
printf("Stack is empty.\n");
return -1;
} else {
return S->data[S->top2++];
}
}
int main() {
DoubleStack S;
InitDoubleStack(&S);
PushStack1(&S, 1);
PushStack1(&S, 2);
PushStack2(&S, 3);
PushStack2(&S, 4);
printf("%d %d %d %d\n", PopStack1(&S), PopStack2(&S), PopStack1(&S), PopStack2(&S)); // 2 4 1 3
return 0;
}
```
希望这能帮到你!
阅读全文
相关推荐
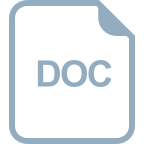
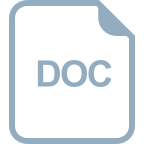















