设将整数1、2、3、4依次进栈,但只要出栈时栈非空,则可将出栈操作按任何次序夹入其中,请写出检验括号匹配的算法,把完整c语言代码给我。
时间: 2024-10-24 12:12:52 浏览: 34
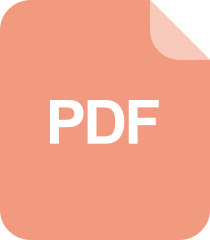
Java定义栈结构,并实现入栈、出栈操作完整示例
这是一个经典的动态规划问题,通常用于检查括号是否匹配,例如 "()"、"[]" 和 "{}" 等。我们可以用一个栈来模拟这个过程,并记录打开和关闭括号的顺序。当遍历到字符串末尾且栈为空时,说明括号配对。以下是使用 C 语言实现的一种简单算法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <string.h>
// 检查括号是否匹配的函数
bool isValid(char* s) {
stack<char> st;
int open_brackets[6] = { '{', '(', '[', '<', '{', '(' };
int close_brackets[6] = { '}', ')', ']', '>', '} ', ')' };
for (int i = 0; s[i]; ++i) {
if (s[i] == open_brackets[0] || s[i] == open_brackets[1] || s[i] == open_brackets[2]) {
st.push(s[i]);
} else if (s[i] == close_brackets[0] || s[i] == close_brackets[1] || s[i] == close_brackets[2]) {
if (st.empty() || st.top() != open_brackets[(int)s[i] - 1]) {
return false;
}
st.pop();
}
}
// 如果还有剩余的开放括号未关闭,也是不匹配的
return st.empty();
}
int main() {
char* test_cases[] = {"()", "({})", "[{)]", "([)]", "{[()]}"};
const int num_test_cases = sizeof(test_cases) / sizeof(test_cases[0]);
for (int i = 0; i < num_test_cases; ++i) {
bool result = isValid(test_cases[i]);
printf("Test case %d: '%s' is %spair\n", i + 1, test_cases[i], result ? "" : "un");
}
return 0;
}
```
在这个代码中,我们首先初始化一个栈 `st` 和两个数组,分别存储开括号和对应的闭括号。然后遍历输入字符串,如果遇到开括号就压入栈,遇到闭括号则判断栈顶元素是否与其对应。如果不匹配或者栈已空,则返回 `false`。最后,如果栈为空,说明所有的括号都正确匹配,返回 `true`。
阅读全文
相关推荐
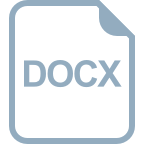
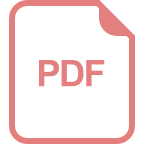















