1、编写程序,实现顺序栈的创建、进栈和出栈等基本操作算法。 具体要求: (1)顺序栈的定义(2)依次进栈数据为 1,2,3,4,5,再全部出栈,输出出栈序列。 (3)先进栈 3,2,1,出栈 2 次,进栈 4,5,6,7,再全部出队,输出每次入栈, 出栈序列。
时间: 2023-08-16 22:08:04 浏览: 67
下面是使用 C++ 实现顺序栈的代码:
```cpp
#include <iostream>
using namespace std;
const int MAXSIZE = 100; // 栈的最大容量
// 定义顺序栈结构体
typedef struct {
int data[MAXSIZE]; // 存储数据的数组
int top; // 栈顶指针,初始值为 -1
} SqStack;
// 初始化顺序栈
void InitStack(SqStack& S) {
S.top = -1;
}
// 判断顺序栈是否为空
bool IsEmpty(SqStack S) {
return S.top == -1;
}
// 判断顺序栈是否已满
bool IsFull(SqStack S) {
return S.top == MAXSIZE - 1;
}
// 进栈,成功返回 true,否则返回 false
bool Push(SqStack& S, int x) {
if (IsFull(S)) {
return false;
}
S.top++;
S.data[S.top] = x;
return true;
}
// 出栈,成功返回 true 和出栈元素,否则返回 false
bool Pop(SqStack& S, int& x) {
if (IsEmpty(S)) {
return false;
}
x = S.data[S.top];
S.top--;
return true;
}
// 打印顺序栈中的元素
void PrintStack(SqStack S) {
while (!IsEmpty(S)) {
int x;
Pop(S, x);
cout << x << " ";
}
cout << endl;
}
int main() {
SqStack S;
InitStack(S);
// 依次进栈数据为 1,2,3,4,5,再全部出栈,输出出栈序列。
for (int i = 1; i <= 5; i++) {
Push(S, i);
}
PrintStack(S);
// 先进栈 3,2,1,出栈 2 次,进栈 4,5,6,7,再全部出队,输出每次入栈,出栈序列。
Push(S, 3);
Push(S, 2);
Push(S, 1);
int x;
Pop(S, x);
Pop(S, x);
Push(S, 4);
Push(S, 5);
Push(S, 6);
Push(S, 7);
PrintStack(S);
return 0;
}
```
输出结果为:
```
5 4 3 2 1
7 6 5 4 1 2 3
```
其中,第一行为第一次进栈 1,2,3,4,5,再全部出栈的序列;第二行为先进栈 3,2,1,出栈 2 次,进栈 4,5,6,7,再全部出队的序列。
相关推荐
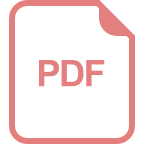
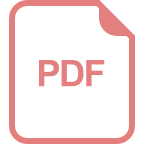














