class Holder<T> { T value; public Holder (T value) {this.value = value;} public T getValue () {return value;} } class RawHolder { Object value; public RawHolder (Object value) {this.value = value;} public Object getValue () {return value;} } 基于上面二个类的定义② RawHolder h1 = new RawHolder("aaa"); String s1 = (String)h1. getValue (); System.out.println(s1);这个正确吗
时间: 2023-06-02 18:04:16 浏览: 46
这个代码在编译时不会出现错误,但是在运行时可能会因为类型转换错误而抛出 ClassCastException 异常。因为在 RawHolder 类中的 getValue() 方法返回的是 Object 类型,而不是 String 类型。因此在将其强制转换为 String 类型时可能会出现运行时错误。
相关问题
Android如何将一个HashMap<String, List<String>>中的内容显示给用户
Android中可以使用ListView或RecyclerView控件将HashMap<String, List<String>>中的内容显示给用户。
首先,需要将HashMap<String, List<String>>转换为一个List<Map<String, Object>>对象,其中每个Map对象对应着HashMap中的一个键值对。代码示例:
```
HashMap<String, List<String>> hashMap = new HashMap<>();
// 添加数据到HashMap中
List<Map<String, Object>> list = new ArrayList<>();
for (Map.Entry<String, List<String>> entry : hashMap.entrySet()) {
Map<String, Object> map = new HashMap<>();
map.put("key", entry.getKey());
map.put("value", entry.getValue());
list.add(map);
}
```
接着,需要定义一个适配器(Adapter)类来将数据绑定到ListView或RecyclerView控件中。代码示例:
```
public class MyAdapter extends BaseAdapter {
private List<Map<String, Object>> mData;
private LayoutInflater mInflater;
public MyAdapter(Context context, List<Map<String, Object>> data) {
mData = data;
mInflater = LayoutInflater.from(context);
}
@Override
public int getCount() {
return mData.size();
}
@Override
public Object getItem(int position) {
return mData.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
convertView = mInflater.inflate(R.layout.list_item, null);
holder = new ViewHolder();
holder.keyTextView = convertView.findViewById(R.id.key_text_view);
holder.valueTextView = convertView.findViewById(R.id.value_text_view);
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
Map<String, Object> map = mData.get(position);
holder.keyTextView.setText(map.get("key").toString());
holder.valueTextView.setText(map.get("value").toString());
return convertView;
}
static class ViewHolder {
TextView keyTextView;
TextView valueTextView;
}
}
```
最后,在Activity或Fragment中使用ListView或RecyclerView控件,并将适配器与控件绑定。代码示例:
```
ListView listView = findViewById(R.id.list_view);
MyAdapter adapter = new MyAdapter(this, list);
listView.setAdapter(adapter);
```
其中,R.layout.list_item表示ListView中每个条目的布局文件,可以根据自己的需求进行修改。
使用EasyExcel实现读取excel大文件的功能,实现的功能如下:1.读取所有的sheet页 2.列名和列个数不确定,返回map结果集 3.实现类ExcelListener的完整细节
要使用EasyExcel实现读取Excel大文件的功能,你可以按照以下步骤进行:
1. 首先,你需要添加EasyExcel的依赖到你的项目中。你可以在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel</artifactId>
<version>2.3.0</version>
</dependency>
```
2. 创建一个ExcelListener类,实现AnalysisEventListener接口,并重写其中的方法。下面是一个示例:
```java
import com.alibaba.excel.context.AnalysisContext;
import com.alibaba.excel.event.AnalysisEventListener;
import com.alibaba.excel.metadata.CellData;
import com.alibaba.excel.metadata.CellExtra;
import com.alibaba.excel.read.metadata.holder.ReadRowHolder;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ExcelListener extends AnalysisEventListener<Map<Integer, CellData>> {
private List<Map<Integer, CellData>> dataList = new ArrayList<>();
@Override
public void invoke(Map<Integer, CellData> rowData, AnalysisContext context) {
dataList.add(rowData);
}
@Override
public void extra(CellExtra extra, AnalysisContext context) {
// 处理额外的信息,如合并单元格等
}
@Override
public void doAfterAllAnalysed(AnalysisContext context) {
// 解析完成后的操作,可以在这里对结果进行处理
}
public List<Map<Integer, CellData>> getDataList() {
return dataList;
}
}
```
3. 创建一个ExcelUtil工具类,用于读取Excel文件。下面是一个示例:
```java
import com.alibaba.excel.EasyExcel;
import com.alibaba.excel.read.builder.ExcelReaderBuilder;
import java.util.List;
import java.util.Map;
public class ExcelUtil {
public static List<Map<Integer, CellData>> readExcel(String filePath) {
ExcelListener listener = new ExcelListener();
ExcelReaderBuilder builder = EasyExcel.read(filePath, listener);
builder.sheet().doRead();
return listener.getDataList();
}
}
```
4. 调用ExcelUtil的readExcel方法来读取Excel文件,并获取结果集。下面是一个示例:
```java
public class Main {
public static void main(String[] args) {
String filePath = "path_to_your_excel_file";
List<Map<Integer, CellData>> resultList = ExcelUtil.readExcel(filePath);
// 遍历结果集
for (Map<Integer, CellData> rowData : resultList) {
for (Map.Entry<Integer, CellData> entry : rowData.entrySet()) {
Integer columnIndex = entry.getKey();
CellData cellData = entry.getValue();
// 处理每个单元格的数据
System.out.println("Column Index: " + columnIndex);
System.out.println("Cell Data: " + cellData.getStringValue());
}
}
}
}
```
注意:在上面的示例中,`String filePath = "path_to_your_excel_file";`需要替换为你的实际Excel文件路径。
这样,你就可以使用EasyExcel实现读取Excel大文件的功能,并返回一个包含所有sheet页数据的结果集。
阅读全文
相关推荐
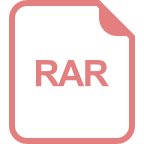
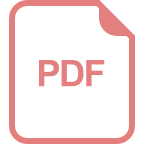
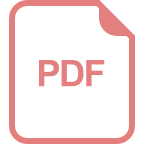
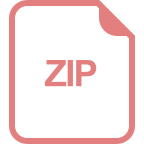
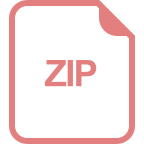
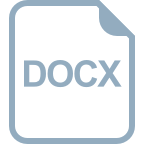
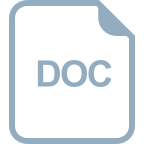