Java怎么连接到数据库
时间: 2023-12-05 19:04:36 浏览: 24
Java连接到数据库的步骤如下:
1. 加载数据库驱动程序
使用`Class.forName()`方法加载相应数据库的驱动程序,例如MySQL的驱动程序类名为`com.mysql.jdbc.Driver`。
2. 创建数据库连接
使用`DriverManager.getConnection()`方法创建数据库连接,需要指定数据库的URL、用户名和密码。
3. 创建Statement对象
使用`Connection.createStatement()`方法创建Statement对象,用于执行SQL语句。
4. 执行SQL语句
使用Statement对象的`executeQuery()`方法执行查询语句,使用`executeUpdate()`方法执行更新、插入或删除操作。
5. 处理查询结果
如果执行的是查询语句,使用ResultSet对象处理查询结果,可以通过`ResultSet.next()`方法遍历查询结果集合。
6. 关闭连接
使用`Connection.close()`方法关闭数据库连接。
以下是一个连接MySQL数据库并执行查询语句的示例代码:
```java
import java.sql.*;
public class TestJDBC {
public static void main(String[] args) {
try {
// 加载MySQL驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 创建数据库连接
String url = "jdbc:mysql://localhost:3306/test";
String username = "root";
String password = "123456";
Connection conn = DriverManager.getConnection(url, username, password);
// 创建Statement对象
Statement stmt = conn.createStatement();
// 执行查询语句
String sql = "SELECT * FROM user";
ResultSet rs = stmt.executeQuery(sql);
// 处理查询结果
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
System.out.println("id: " + id + ", name: " + name);
}
// 关闭连接
rs.close();
stmt.close();
conn.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
相关推荐
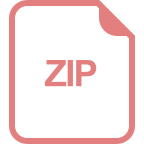
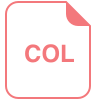
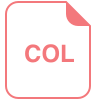
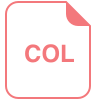
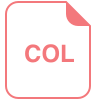
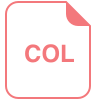









