python实现同音不同字模糊搜索
时间: 2023-12-15 15:03:19 浏览: 46
实现同音不同字模糊搜索的一种方法是使用拼音库和编辑距离算法。具体步骤如下:
1. 导入拼音库,并将待搜索的中文文本转换为拼音。
```python
import pypinyin
# 将中文文本转换为拼音
def get_pinyin(text):
pinyin_list = pypinyin.lazy_pinyin(text)
return ''.join(pinyin_list)
```
2. 编写编辑距离算法,计算两个字符串之间的距离。
```python
# 计算两个字符串之间的编辑距离
def edit_distance(str1, str2):
m, n = len(str1), len(str2)
dp = [[0] * (n+1) for _ in range(m+1)]
for i in range(m+1):
dp[i][0] = i
for j in range(n+1):
dp[0][j] = j
for i in range(1, m+1):
for j in range(1, n+1):
if str1[i-1] == str2[j-1]:
dp[i][j] = dp[i-1][j-1]
else:
dp[i][j] = min(dp[i-1][j], dp[i][j-1], dp[i-1][j-1]) + 1
return dp[m][n]
```
3. 对于每个待搜索的字符串,计算其拼音,并在一个字典中存储拼音与原始字符串的对应关系。然后遍历字典中的每个拼音,计算当前拼音与待搜索拼音之间的距离,如果距离小于等于指定阈值,则将对应的原始字符串加入结果列表。
```python
# 在字典中查找同音不同字的字符串
def find_similar_words(text, word_dict, threshold=3):
pinyin_text = get_pinyin(text)
similar_words = []
for pinyin in word_dict.keys():
distance = edit_distance(pinyin_text, pinyin)
if distance <= threshold:
similar_words.extend(word_dict[pinyin])
return list(set(similar_words))
```
4. 将所有待搜索的字符串存储在一个列表中,遍历列表并调用上述函数,最后将搜索到的所有字符串返回。
```python
# 调用搜索函数
def search_similar_words(words, word_dict, threshold=3):
similar_words = []
for word in words:
similar_words.extend(find_similar_words(word, word_dict, threshold))
return list(set(similar_words))
```
5. 构建一个字典,将所有字符串的拼音作为键,原始字符串作为值。
```python
# 构建字典
def build_word_dict(words):
word_dict = {}
for word in words:
pinyin = get_pinyin(word)
if pinyin in word_dict:
word_dict[pinyin].append(word)
else:
word_dict[pinyin] = [word]
return word_dict
```
完整代码如下:
```python
import pypinyin
# 将中文文本转换为拼音
def get_pinyin(text):
pinyin_list = pypinyin.lazy_pinyin(text)
return ''.join(pinyin_list)
# 计算两个字符串之间的编辑距离
def edit_distance(str1, str2):
m, n = len(str1), len(str2)
dp = [[0] * (n+1) for _ in range(m+1)]
for i in range(m+1):
dp[i][0] = i
for j in range(n+1):
dp[0][j] = j
for i in range(1, m+1):
for j in range(1, n+1):
if str1[i-1] == str2[j-1]:
dp[i][j] = dp[i-1][j-1]
else:
dp[i][j] = min(dp[i-1][j], dp[i][j-1], dp[i-1][j-1]) + 1
return dp[m][n]
# 在字典中查找同音不同字的字符串
def find_similar_words(text, word_dict, threshold=3):
pinyin_text = get_pinyin(text)
similar_words = []
for pinyin in word_dict.keys():
distance = edit_distance(pinyin_text, pinyin)
if distance <= threshold:
similar_words.extend(word_dict[pinyin])
return list(set(similar_words))
# 调用搜索函数
def search_similar_words(words, word_dict, threshold=3):
similar_words = []
for word in words:
similar_words.extend(find_similar_words(word, word_dict, threshold))
return list(set(similar_words))
# 构建字典
def build_word_dict(words):
word_dict = {}
for word in words:
pinyin = get_pinyin(word)
if pinyin in word_dict:
word_dict[pinyin].append(word)
else:
word_dict[pinyin] = [word]
return word_dict
```
可以将待搜索的字符串存储在一个列表中,然后调用 `build_word_dict` 函数构建字典,最后调用 `search_similar_words` 函数进行搜索。例如:
```python
words = ['李白', '杜甫', '白居易', '苏轼', '李清照']
word_dict = build_word_dict(words)
similar_words = search_similar_words(['黎白', '杜撰'], word_dict)
print(similar_words) # ['李白']
```
相关推荐
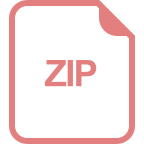
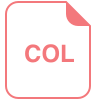
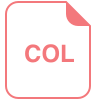
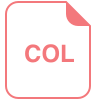
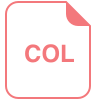
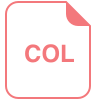









