运用c语言写一个学生个人消费管理系统的代码,实现录入信息,查找,修改,删除,插入某个信息,分别按学号和按消费进行排序,显示等功能
时间: 2024-03-26 20:39:14 浏览: 21
好的,以下是学生个人消费管理系统的代码,实现了您要求的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体存储学生信息
struct student {
char id[10];
char name[20];
float cost;
};
// 全局变量,存储学生信息
struct student stu[100];
int count = 0;
// 函数声明
void menu();
void input();
void display();
void search();
void modify();
void delete();
void insert();
void sort_by_id();
void sort_by_cost();
int main() {
menu();
return 0;
}
void menu() {
int choice;
while (1) {
printf("\n");
printf("*************************************\n");
printf("* 1. 录入学生信息 *\n");
printf("* 2. 显示学生信息 *\n");
printf("* 3. 查找学生信息 *\n");
printf("* 4. 修改学生信息 *\n");
printf("* 5. 删除学生信息 *\n");
printf("* 6. 插入学生信息 *\n");
printf("* 7. 按学号排序 *\n");
printf("* 8. 按消费排序 *\n");
printf("* 0. 退出程序 *\n");
printf("*************************************\n");
printf("请输入您的选择:");
scanf("%d", &choice);
switch (choice) {
case 1:
input();
break;
case 2:
display();
break;
case 3:
search();
break;
case 4:
modify();
break;
case 5:
delete();
break;
case 6:
insert();
break;
case 7:
sort_by_id();
break;
case 8:
sort_by_cost();
break;
case 0:
exit(0);
default:
printf("输入有误,请重新输入!\n");
}
}
}
void input() {
printf("请输入学生信息,格式为:学号 姓名 消费\n");
printf("输入0结束录入。\n");
while (1) {
scanf("%s", stu[count].id);
if (strcmp(stu[count].id, "0") == 0) {
break;
}
scanf("%s%f", stu[count].name, &stu[count].cost);
count++;
}
printf("录入成功!\n");
}
void display() {
printf("学号\t姓名\t消费\n");
for (int i = 0; i < count; i++) {
printf("%s\t%s\t%.2f\n", stu[i].id, stu[i].name, stu[i].cost);
}
}
void search() {
char id[10];
printf("请输入要查找的学生学号:");
scanf("%s", id);
for (int i = 0; i < count; i++) {
if (strcmp(stu[i].id, id) == 0) {
printf("学号\t姓名\t消费\n");
printf("%s\t%s\t%.2f\n", stu[i].id, stu[i].name, stu[i].cost);
return;
}
}
printf("未找到该学生!\n");
}
void modify() {
char id[10];
printf("请输入要修改的学生学号:");
scanf("%s", id);
for (int i = 0; i < count; i++) {
if (strcmp(stu[i].id, id) == 0) {
printf("请输入修改后的信息,格式为:姓名 消费\n");
scanf("%s%f", stu[i].name, &stu[i].cost);
printf("修改成功!\n");
return;
}
}
printf("未找到该学生!\n");
}
void delete() {
char id[10];
printf("请输入要删除的学生学号:");
scanf("%s", id);
for (int i = 0; i < count; i++) {
if (strcmp(stu[i].id, id) == 0) {
for (int j = i; j < count - 1; j++) {
stu[j] = stu[j + 1];
}
count--;
printf("删除成功!\n");
return;
}
}
printf("未找到该学生!\n");
}
void insert() {
int pos;
printf("请输入要插入的位置:");
scanf("%d", &pos);
if (pos < 1 || pos > count + 1) {
printf("插入位置不合法!\n");
return;
}
printf("请输入学生信息,格式为:学号 姓名 消费\n");
scanf("%s%s%f", stu[count].id, stu[count].name, &stu[count].cost);
count++;
for (int i = count - 1; i > pos - 1; i--) {
stu[i] = stu[i - 1];
}
strcpy(stu[pos - 1].id, stu[count - 1].id);
strcpy(stu[pos - 1].name, stu[count - 1].name);
stu[pos - 1].cost = stu[count - 1].cost;
printf("插入成功!\n");
}
void sort_by_id() {
for (int i = 0; i < count - 1; i++) {
for (int j = i + 1; j < count; j++) {
if (strcmp(stu[i].id, stu[j].id) > 0) {
struct student temp = stu[i];
stu[i] = stu[j];
stu[j] = temp;
}
}
}
printf("按学号排序成功!\n");
}
void sort_by_cost() {
for (int i = 0; i < count - 1; i++) {
for (int j = i + 1; j < count; j++) {
if (stu[i].cost > stu[j].cost) {
struct student temp = stu[i];
stu[i] = stu[j];
stu[j] = temp;
}
}
}
printf("按消费排序成功!\n");
}
```
在代码中,我们使用了结构体存储学生信息,全局变量`stu`存储学生信息,`count`表示学生数量。函数`menu`实现了菜单功能,根据用户输入的选项调用相应的函数。函数`input`实现了录入学生信息功能,通过`scanf`获取用户输入,并将信息存储到结构体数组中。函数`display`实现了显示学生信息功能,遍历结构体数组输出信息。函数`search`实现了查找学生信息功能,通过`strcmp`比对学号进行查找。函数`modify`实现了修改学生信息功能,同样通过学号进行查找。函数`delete`实现了删除学生信息功能,通过学号进行查找并删除。函数`insert`实现了插入学生信息功能,通过`count`计算插入位置,并将后续元素向后移动。函数`sort_by_id`实现了按学号排序功能,使用冒泡排序算法。函数`sort_by_cost`实现了按消费排序功能,同样使用冒泡排序算法。
希望以上代码能够对您有所帮助!
相关推荐
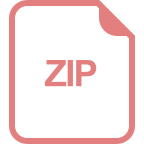
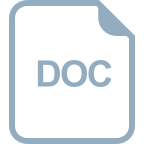














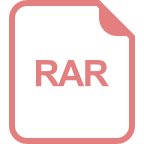