使用c语言,利用线性表的基本操作实现一个班级学生信息(包括:学号,姓名,学院,专业,班级,性别,年龄等)管理系统,主要功能包括:数据录入,插入,删除,输出,查找等
时间: 2024-05-12 15:19:02 浏览: 143
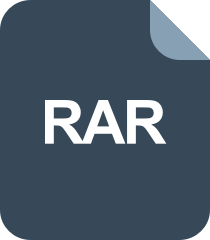
学生信息管理系统,实现学生信息包括:学号、姓名、性别、年龄、班级等信息。

首先,我们需要定义学生信息的结构体:
```c
#define MAX_NAME_LENGTH 20
#define MAX_COLLEGE_LENGTH 30
#define MAX_MAJOR_LENGTH 30
#define MAX_CLASS_LENGTH 10
typedef struct {
int id;
char name[MAX_NAME_LENGTH];
char college[MAX_COLLEGE_LENGTH];
char major[MAX_MAJOR_LENGTH];
char class[MAX_CLASS_LENGTH];
char gender;
int age;
} Student;
```
然后,我们需要定义线性表的结构体和基本操作函数:
```c
#define MAX_STUDENT_NUM 100
typedef struct {
Student data[MAX_STUDENT_NUM];
int length;
} SeqList;
// 初始化线性表
void InitList(SeqList *L);
// 插入元素
void InsertList(SeqList *L, int i, Student e);
// 删除元素
void DeleteList(SeqList *L, int i);
// 查找元素
int SearchList(SeqList L, int id);
// 输出所有元素
void PrintList(SeqList L);
```
接下来,我们可以实现具体的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "student.h"
void Menu();
void AddStudent(SeqList *L);
void InsertStudent(SeqList *L);
void DeleteStudent(SeqList *L);
void SearchStudent(SeqList L);
void PrintAllStudent(SeqList L);
int main() {
SeqList L;
InitList(&L);
int choice;
do {
Menu();
scanf("%d", &choice);
switch (choice) {
case 1:
AddStudent(&L);
break;
case 2:
InsertStudent(&L);
break;
case 3:
DeleteStudent(&L);
break;
case 4:
SearchStudent(L);
break;
case 5:
PrintAllStudent(L);
break;
case 0:
printf("Exit.\n");
break;
default:
printf("Invalid choice.\n");
break;
}
} while (choice != 0);
return 0;
}
void Menu() {
printf("\n**************************\n");
printf("1. Add Student\n");
printf("2. Insert Student\n");
printf("3. Delete Student\n");
printf("4. Search Student\n");
printf("5. Print All Student\n");
printf("0. Exit\n");
printf("**************************\n");
printf("Please enter your choice: ");
}
void AddStudent(SeqList *L) {
if (L->length >= MAX_STUDENT_NUM) {
printf("The list is full.\n");
return;
}
Student s;
printf("Please enter the student's information:\n");
printf("ID: ");
scanf("%d", &s.id);
printf("Name: ");
scanf("%s", s.name);
printf("College: ");
scanf("%s", s.college);
printf("Major: ");
scanf("%s", s.major);
printf("Class: ");
scanf("%s", s.class);
printf("Gender (M/F): ");
scanf(" %c", &s.gender);
printf("Age: ");
scanf("%d", &s.age);
L->data[L->length++] = s;
printf("Add student successfully.\n");
}
void InsertStudent(SeqList *L) {
if (L->length >= MAX_STUDENT_NUM) {
printf("The list is full.\n");
return;
}
int id, index;
printf("Please enter the student's ID and the index to insert:\n");
printf("ID: ");
scanf("%d", &id);
index = SearchList(*L, id);
if (index == -1) {
printf("Student not found.\n");
return;
}
Student s;
printf("Please enter the student's information:\n");
printf("ID: ");
scanf("%d", &s.id);
printf("Name: ");
scanf("%s", s.name);
printf("College: ");
scanf("%s", s.college);
printf("Major: ");
scanf("%s", s.major);
printf("Class: ");
scanf("%s", s.class);
printf("Gender (M/F): ");
scanf(" %c", &s.gender);
printf("Age: ");
scanf("%d", &s.age);
InsertList(L, index + 1, s);
printf("Insert student successfully.\n");
}
void DeleteStudent(SeqList *L) {
int id, index;
printf("Please enter the student's ID to delete:\n");
printf("ID: ");
scanf("%d", &id);
index = SearchList(*L, id);
if (index == -1) {
printf("Student not found.\n");
return;
}
DeleteList(L, index + 1);
printf("Delete student successfully.\n");
}
void SearchStudent(SeqList L) {
int id, index;
printf("Please enter the student's ID to search:\n");
printf("ID: ");
scanf("%d", &id);
index = SearchList(L, id);
if (index == -1) {
printf("Student not found.\n");
return;
}
printf("ID\tName\tCollege\tMajor\tClass\tGender\tAge\n");
printf("%d\t%s\t%s\t%s\t%s\t%c\t%d\n", L.data[index].id, L.data[index].name, L.data[index].college, L.data[index].major, L.data[index].class, L.data[index].gender, L.data[index].age);
}
void PrintAllStudent(SeqList L) {
printf("ID\tName\tCollege\tMajor\tClass\tGender\tAge\n");
for (int i = 0; i < L.length; i++) {
printf("%d\t%s\t%s\t%s\t%s\t%c\t%d\n", L.data[i].id, L.data[i].name, L.data[i].college, L.data[i].major, L.data[i].class, L.data[i].gender, L.data[i].age);
}
}
```
最后,我们可以编译并运行程序,测试它的功能。
阅读全文
相关推荐
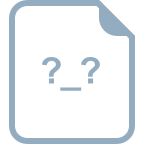





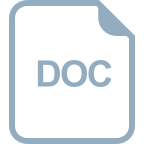
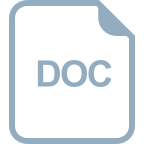







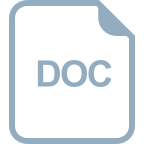
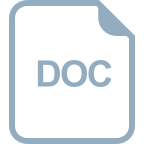