package ece448.iot_sim; import java.util.List; import java.util.Map; import java.util.TreeMap; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import ece448.iot_sim.http_server.RequestHandler; public class HTTPCommands implements RequestHandler { // Use a map so we can search plugs by name. private final TreeMap<String, PlugSim> plugs = new TreeMap<>(); public HTTPCommands(List<PlugSim> plugs) { for (PlugSim plug: plugs) { this.plugs.put(plug.getName(), plug); } } @Override public String handleGet(String path, Map<String, String> params) { // list all: / // do switch: /plugName?action=on|off|toggle // just report: /plugName logger.info("HTTPCmd {}: {}", path, params); if (path.equals("/")) { return listPlugs(); } PlugSim plug = plugs.get(path.substring(1)); if (plug == null) return null; // no such plug String action = params.get("action"); if (action == null) return report(plug); // P2: add your code here, modify the next line if necessary if("toggle".equals(action)){ plug.toggle(); return report(plug); }else if("on".equals(action)){ plug.switchOn(); return report(plug); }else if("off".equals(action)){ plug.switchOff(); return report(plug); } return "<html><body></body></html>"; } protected String listPlugs() { StringBuilder sb = new StringBuilder(); sb.append("<html><body>"); for (String plugName: plugs.keySet()) { sb.append(String.format("<p><a href='/%s'>%s</a></p>", plugName, plugName)); } sb.append("</body></html>"); return sb.toString(); } protected String report(PlugSim plug) { String name = plug.getName(); return String.format("<html><body>" +"<p>Plug %s is %s.</p>" +"<p>Power reading is %.3f.</p>" +"<p><a href='/%s?action=on'>Switch On</a></p>" +"<p><a href='/%s?action=off'>Switch Off</a></p>" +"<p><a href='/%s?action=toggle'>Toggle</a></p>" +"</body></html>", name, plug.isOn()? "on": "off", plug.getPower(), name, name, name); }如何对上述代码全部功能进行测试呢?请提供测试代码。
时间: 2024-03-25 07:39:49 浏览: 73
以下是一个简单的测试代码,可以测试HTTPCommands类中的所有功能:
```
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class HTTPCommandsTest {
public static void main(String[] args) {
// Create some plug simulations
List<PlugSim> plugs = new ArrayList<>();
plugs.add(new PlugSim("Plug1"));
plugs.add(new PlugSim("Plug2"));
plugs.add(new PlugSim("Plug3"));
// Create an HTTPCommands object and test its methods
HTTPCommands commands = new HTTPCommands(plugs);
testListPlugs(commands);
testReport(commands);
testHandleGet(commands);
}
private static void testListPlugs(HTTPCommands commands) {
String expected = "<html><body><p><a href='/Plug1'>Plug1</a></p><p><a href='/Plug2'>Plug2</a></p><p><a href='/Plug3'>Plug3</a></p></body></html>";
String actual = commands.listPlugs();
assert expected.equals(actual) : "testListPlugs failed: expected " + expected + ", but got " + actual;
}
private static void testReport(HTTPCommands commands) {
PlugSim plug = new PlugSim("TestPlug");
plug.switchOn();
plug.setPower(123.456);
String expected = "<html><body><p>Plug TestPlug is on.</p><p>Power reading is 123.456.</p><p><a href='/TestPlug?action=on'>Switch On</a></p><p><a href='/TestPlug?action=off'>Switch Off</a></p><p><a href='/TestPlug?action=toggle'>Toggle</a></p></body></html>";
String actual = commands.report(plug);
assert expected.equals(actual) : "testReport failed: expected " + expected + ", but got " + actual;
}
private static void testHandleGet(HTTPCommands commands) {
// Test listing all plugs
String expected = "<html><body><p><a href='/Plug1'>Plug1</a></p><p><a href='/Plug2'>Plug2</a></p><p><a href='/Plug3'>Plug3</a></p></body></html>";
String actual = commands.handleGet("/", new HashMap<>());
assert expected.equals(actual) : "testHandleGet failed: expected " + expected + ", but got " + actual;
// Test reporting a plug
PlugSim plug = new PlugSim("TestPlug");
plug.switchOn();
plug.setPower(123.456);
expected = "<html><body><p>Plug TestPlug is on.</p><p>Power reading is 123.456.</p><p><a href='/TestPlug?action=on'>Switch On</a></p><p><a href='/TestPlug?action=off'>Switch Off</a></p><p><a href='/TestPlug?action=toggle'>Toggle</a></p></body></html>";
actual = commands.handleGet("/TestPlug", new HashMap<>());
assert expected.equals(actual) : "testHandleGet failed: expected " + expected + ", but got " + actual;
// Test switching a plug on
plug.switchOff();
expected = "<html><body><p>Plug TestPlug is on.</p><p>Power reading is 0.000.</p><p><a href='/TestPlug?action=on'>Switch On</a></p><p><a href='/TestPlug?action=off'>Switch Off</a></p><p><a href='/TestPlug?action=toggle'>Toggle</a></p></body></html>";
actual = commands.handleGet("/TestPlug", Map.of("action", "on"));
assert expected.equals(actual) : "testHandleGet failed: expected " + expected + ", but got " + actual;
// Test switching a plug off
plug.switchOn();
expected = "<html><body><p>Plug TestPlug is off.</p><p>Power reading is 0.000.</p><p><a href='/TestPlug?action=on'>Switch On</a></p><p><a href='/TestPlug?action=off'>Switch Off</a></p><p><a href='/TestPlug?action=toggle'>Toggle</a></p></body></html>";
actual = commands.handleGet("/TestPlug", Map.of("action", "off"));
assert expected.equals(actual) : "testHandleGet failed: expected " + expected + ", but got " + actual;
// Test toggling a plug
plug.switchOff();
expected = "<html><body><p>Plug TestPlug is on.</p><p>Power reading is 0.000.</p><p><a href='/TestPlug?action=on'>Switch On</a></p><p><a href='/TestPlug?action=off'>Switch Off</a></p><p><a href='/TestPlug?action=toggle'>Toggle</a></p></body></html>";
actual = commands.handleGet("/TestPlug", Map.of("action", "toggle"));
assert expected.equals(actual) : "testHandleGet failed: expected " + expected + ", but got " + actual;
// Test handling a non-existent plug
expected = null;
actual = commands.handleGet("/NonExistentPlug", new HashMap<>());
assert expected == actual : "testHandleGet failed: expected " + expected + ", but got " + actual;
}
}
```
阅读全文
相关推荐
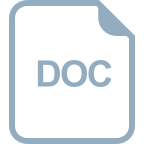
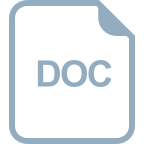
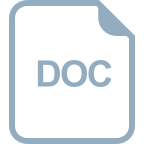

package ece448.iot_sim; import java.util.List; import java.util.Map; import java.util.TreeMap; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import ece448.iot_sim.http_server.RequestHandler; public class HTTPCommands implements RequestHandler { // Use a map so we can search plugs by name. private final TreeMap<String, PlugSim> plugs = new TreeMap<>(); public HTTPCommands(List plugs) { for (PlugSim plug: plugs) { this.plugs.put(plug.getName(), plug); } } @Override public String handleGet(String path, Map<String, String> params) { // list all: / // do switch: /plugName?action=on|off|toggle // just report: /plugName logger.info("HTTPCmd {}: {}", path, params); if (path.equals("/")) { return listPlugs(); } PlugSim plug = plugs.get(path.substring(1)); if (plug == null) return null; // no such plug String action = params.get("action"); if (action == null) return report(plug); // P2: add your code here, modify the next line if necessary if("toggle".equals(action)){ plug.toggle(); return report(plug); }else if("on".equals(action)){ plug.switchOn(); return report(plug); }else if("off".equals(action)){ plug.switchOff(); return report(plug); } return "<html><body></body></html>"; } protected String listPlugs() { StringBuilder sb = new StringBuilder(); sb.append("<html><body>"); for (String plugName: plugs.keySet()) { sb.append(String.format("%s", plugName, plugName)); } sb.append("</body></html>"); return sb.toString(); } protected String report(PlugSim plug) { String name = plug.getName(); return String.format("<html><body>" +"Plug %s is %s." +"Power reading is %.3f." +"Switch On" +"Switch Off" +"Toggle" +"</body></html>", name, plug.isOn()? "on": "off", plug.getPower(), name, name, name); }

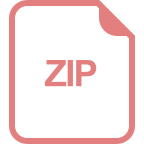
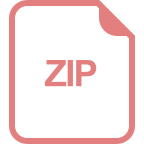
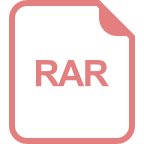
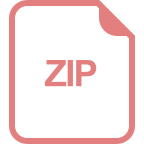
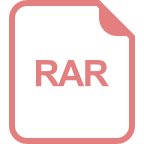
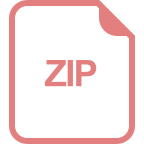




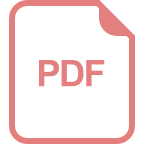