#include<stdio.h> #include<string.h> #include<stdlib.h> struct student{ char number[10]; char name[15]; double cscore; double mathscore; double englishscore; double sum; struct student *next; }; struct student *getfiledata() { FILE *fp; struct student *head,*tail,*p; head=tail=p; if((fp=fopen("data.txt","r"))==NULL) { printf("File open error!\n"); exit(0); } while(!feof(fp)) { p=(struct student*)malloc(sizeof(struct student)); fscanf(fp,"%s%s%f%f%f%f",p->number,p->name,&p->cscore,&p->mathscore,&p->englishscore,&p->sum); if(head==NULL) head=p; else tail->next=p; tail=p; p->next=NULL; } if(fclose(fp)){ printf("Can not close the file!\n"); exit(0); } return head; } void numberchaxun(struct student *h) { struct student *p1=h; char number1[10]; int flag=0; printf("请输入要查询的学生的学号:");scanf("%s",number1); for(;p1!=NULL;p1=p1->next) if(strcmp(p1->number,number1)==0) { flag=1; printf("%s %s %f %f %f %f",p1->number,p1->name,p1->cscore,p1->mathscore,p1->englishscore,p1->sum); break; } if(!flag)printf("没有该学号\n"); } void main() { numberchaxun(getfiledata()); }出现了什么错误,不能打印
时间: 2024-03-04 08:48:14 浏览: 23
根据代码分析,可能是因为输出语句中缺少换行符导致输出不正常。你可以在输出语句的最后加上换行符`\n`来解决这个问题,例如:
```
printf("%s %s %f %f %f %f\n",p1->number,p1->name,p1->cscore,p1->mathscore,p1->englishscore,p1->sum);
```
另外,建议在程序结束时释放动态申请的内存,避免内存泄漏。可以在`getfiledata()`函数中添加如下语句:
```
if(p != NULL) {
free(p);
}
```
这样可以释放最后一次循环中申请的内存。
相关问题
#include<stdio.h> #include<stdlib.h> #include<string.h> #include<time.h> struct information { int number;//学生序号 char gender[10];//学生性别 char name[10];//学生姓名 char id[20];//学生学号 char clas[40];//学生班级 }student[100]; void student_information_print(str
uct information stu[], int n) { //打印学生信息 printf("学生信息如下:\n"); printf("序号\t性别\t姓名\t学号\t\t班级\n"); for(int i=0;i<n;i++) { printf("%d\t%s\t%s\t%s\t%s\n",stu[i].number,stu[i].gender,stu[i].name,stu[i].id,stu[i].clas); } } int main() { int n;//学生人数 printf("请输入学生人数:"); scanf("%d",&n); for(int i=0;i<n;i++) { printf("请输入第%d个学生的信息:\n",i+1); printf("序号:"); scanf("%d",&student[i].number); printf("性别:"); scanf("%s",student[i].gender); printf("姓名:"); scanf("%s",student[i].name); printf("学号:"); scanf("%s",student[i].id); printf("班级:"); scanf("%s",student[i].clas); } student_information_print(student,n); return 0; }
这是一个简单的学生信息管理程序,通过结构体存储学生信息,并可以打印出所有学生信息。你可以把这段代码保存为一个 C 文件,然后使用 GCC 编译器编译运行即可。
c语言的学生管理系统在此代码基础上写一个删除学生信息#include<stdio.h> #include<conio.h> #include<stdlib.h> #include<string.h> typedef struct _student{ int ID; char name[20]; char sex[5]; char Class[20]; char date[20]; char Tel[20]; char home[20]; }student; typedef struc
t _node{ student data; struct _node *next; }node; node *createList() { node *head,*p; head = p = (node*)malloc(sizeof(node)); p->next = NULL; return head; } void addStudent(node *head, student *newStu) { node *p, *q; p = head; while(p->next != NULL) { p = p->next; } q = (node*)malloc(sizeof(node)); q->data = *newStu; q->next = p->next; p->next = q; } void displayList(node *head) { node *p; p = head->next; while(p != NULL) { printf("%d\t%s\t%s\t%s\t%s\t%s\t%s\n", p->data.ID, p->data.name, p->data.sex, p->data.Class, p->data.date, p->data.Tel, p->data.home); p = p->next; } } void deleteStudent(node *head, int ID) { node *p, *q; p = head->next; while(p != NULL && p->data.ID != ID) { q = p; p = p->next; } if(p == NULL) { printf("No student with ID %d exists.\n", ID); } else { q->next = p->next; free(p); printf("Student with ID %d has been deleted.\n", ID); } } int main() { node *head; student newStu; int choice, ID; char name[20], sex[5], Class[20], date[20], Tel[20], home[20]; head = createList(); do { printf("Menu:\n"); printf("1. Add student\n"); printf("2. Display all students\n"); printf("3. Delete student\n"); printf("4. Exit\n"); printf("Enter your choice: "); scanf("%d", &choice); switch(choice) { case 1: printf("Enter student ID: "); scanf("%d", &newStu.ID); printf("Enter student name: "); scanf("%s", &newStu.name); printf("Enter student sex: "); scanf("%s", &newStu.sex); printf("Enter student class: "); scanf("%s", &newStu.Class); printf("Enter student date of birth: "); scanf("%s", &newStu.date); printf("Enter student telephone number: "); scanf("%s", &newStu.Tel); printf("Enter student home address: "); scanf("%s", &newStu.home); addStudent(head, &newStu); break; case 2: displayList(head); break; case 3: printf("Enter student ID to delete: "); scanf("%d", &ID); deleteStudent(head, ID); break; case 4: printf("Exiting program...\n"); break; default: printf("Invalid choice. Please try again.\n"); break; } } while(choice != 4); return 0; }
相关推荐
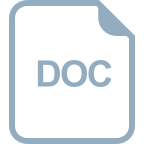
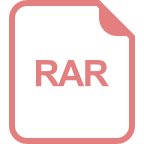
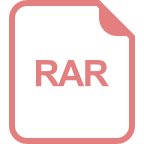
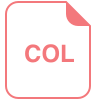
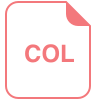












