利用2位七段LED显示计秒数值,利用内部定时器实现秒表功能,计秒数值在2位LED上显示,显示时间范围为0-99s,初始值为00,用1个按键控制秒表启停,停止后数码管显示当前值,再次启动时数码管先清零然后重新开始计数,溢出后循环显示。
时间: 2024-03-27 17:41:12 浏览: 104
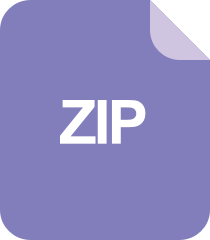
本设计可直接用作时钟计数器,同时有调时,定时功能。 Led[3_0]显示秒钟的变化情况。 func用作计时,调时,定时功能转换
好的,这是一个关于秒表的问题。以下是一个基于51单片机的简单实现步骤:
1. 首先需要选择合适的2位七段LED数码管模块,并连接至单片机的IO口。
2. 设置定时器,定时器溢出时间为1秒,每次溢出后计数器加1。
3. 设置外部中断,用于控制秒表的启停。
4. 初始化计数器为0,显示器显示00。
5. 每次定时器溢出后,更新计数器的值,并将其转换为两位数的十进制数。可以使用以下代码进行转换:
```c
void decimalToBCD(unsigned char decimal, unsigned char *BCD_high, unsigned char *BCD_low) {
*BCD_high = decimal / 10;
*BCD_low = decimal % 10;
}
```
其中,decimal为十进制数值,BCD_high和BCD_low为BCD码高低位的指针。
6. 将转换后的数值显示在LED上,可以使用以下代码进行显示:
```c
void display(unsigned char high, unsigned char low) {
unsigned char code table[]={
0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90
};
P2 = 0xff;
P0 = table[high];
P2 = 0xfe;
delay(5);
P2 = 0xff;
P0 = table[low];
P2 = 0xfd;
delay(5);
}
```
其中,table[]数组为BCD码到数码管段码的转换表,high和low为BCD码高低位的值。
7. 当按下外部中断时,判断秒表的状态,如果是启动状态则停止,如果是停止状态则重新开始计数,并将显示器清零。
8. 当计数器溢出时,将计数器清零并重新开始计数。
9. 实现以上功能的代码如下:
```c
#include <reg52.h>
typedef unsigned char uchar;
typedef unsigned int uint;
sbit KEY = P1^0;
void delay(uint xms) {
uint i, j;
for(i = xms; i > 0; i--)
for(j = 110; j > 0; j--);
}
void decimalToBCD(uchar decimal, uchar *BCD_high, uchar *BCD_low) {
*BCD_high = decimal / 10;
*BCD_low = decimal % 10;
}
void display(uchar high, uchar low) {
uchar code table[]={
0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90
};
P2 = 0xff;
P0 = table[high];
P2 = 0xfe;
delay(5);
P2 = 0xff;
P0 = table[low];
P2 = 0xfd;
delay(5);
}
void main() {
TMOD = 0x01;
TH0 = 0x4c;
TL0 = 0x00;
TR0 = 1;
ET0 = 1;
EA = 1;
uchar second = 0;
uchar BCD_high = 0;
uchar BCD_low = 0;
uchar flag = 0;
display(BCD_high, BCD_low);
while(1) {
if(KEY == 0) {
delay(10);
if(KEY == 0) {
flag = !flag;
while(!KEY);
}
}
if(flag) {
if(second == 100) {
second = 0;
BCD_high = 0;
BCD_low = 0;
}
decimalToBCD(second, &BCD_high, &BCD_low);
display(BCD_high, BCD_low);
} else {
display(BCD_high, BCD_low);
}
}
}
void timer0() interrupt 1 {
TH0 = 0x4c;
TL0 = 0x00;
second++;
}
```
以上是一个简单的秒表实现步骤,具体实现还需要考虑硬件选型和具体编程实现。
阅读全文
相关推荐
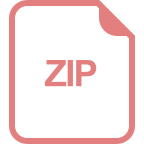
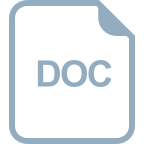

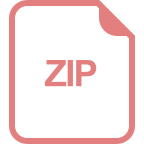
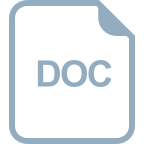


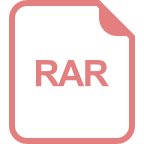
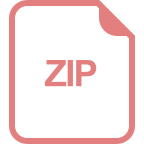
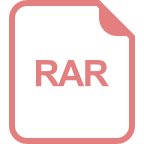
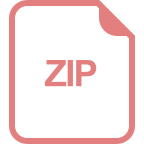
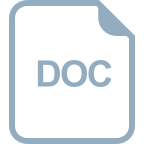
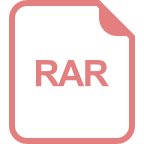
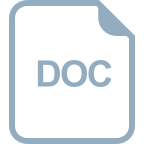
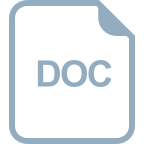
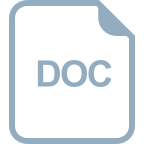
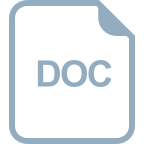