java程序能实现矩阵的加减乘运算
时间: 2023-05-21 20:00:45 浏览: 60
是的,Java程序可以实现矩阵的加减乘运算。您可以使用Java中的多维数组来表示矩阵,并使用循环来实现矩阵的运算。此外,您还可以使用Java中的矩阵库,如Apache Commons Math库,来实现矩阵运算。
相关问题
编写java程序实现矩阵加减乘的运算
好的,以下是一个简单的 Java 程序实现矩阵加减乘的运算:
```java
public class Matrix {
private int[][] data;
private int rows;
private int cols;
public Matrix(int[][] data) {
this.data = data;
this.rows = data.length;
this.cols = data[0].length;
}
public Matrix add(Matrix other) {
if (this.rows != other.rows || this.cols != other.cols) {
throw new IllegalArgumentException("Matrices must have the same dimensions");
}
int[][] result = new int[rows][cols];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result[i][j] = this.data[i][j] + other.data[i][j];
}
}
return new Matrix(result);
}
public Matrix subtract(Matrix other) {
if (this.rows != other.rows || this.cols != other.cols) {
throw new IllegalArgumentException("Matrices must have the same dimensions");
}
int[][] result = new int[rows][cols];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result[i][j] = this.data[i][j] - other.data[i][j];
}
}
return new Matrix(result);
}
public Matrix multiply(Matrix other) {
if (this.cols != other.rows) {
throw new IllegalArgumentException("Number of columns in first matrix must match number of rows in second matrix");
}
int[][] result = new int[this.rows][other.cols];
for (int i = 0; i < this.rows; i++) {
for (int j = 0; j < other.cols; j++) {
int sum = 0;
for (int k = 0; k < this.cols; k++) {
sum += this.data[i][k] * other.data[k][j];
}
result[i][j] = sum;
}
}
return new Matrix(result);
}
}
```
这个类中包含了三个方法,分别是 `add`、`subtract` 和 `multiply`,分别用于实现矩阵的加法、减法和乘法运算。这些方法都接受一个 `Matrix` 类型的参数,表示另一个矩阵,然后返回一个新的 `Matrix` 对象,表示运算的结果。
java 用数组计算矩阵的加减乘
Java中可以使用二维数组来表示矩阵,然后通过循环遍历数组进行加减乘运算。以下是一个示例代码:
// 矩阵加法
public static int[][] matrixAdd(int[][] a, int[][] b) {
int row = a.length;
int col = a[0].length;
int[][] result = new int[row][col];
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
result[i][j] = a[i][j] + b[i][j];
}
}
return result;
}
// 矩阵减法
public static int[][] matrixSubtract(int[][] a, int[][] b) {
int row = a.length;
int col = a[0].length;
int[][] result = new int[row][col];
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
result[i][j] = a[i][j] - b[i][j];
}
}
return result;
}
// 矩阵乘法
public static int[][] matrixMultiply(int[][] a, int[][] b) {
int rowA = a.length;
int colA = a[0].length;
int colB = b[0].length;
int[][] result = new int[rowA][colB];
for (int i = 0; i < rowA; i++) {
for (int j = 0; j < colB; j++) {
for (int k = 0; k < colA; k++) {
result[i][j] += a[i][k] * b[k][j];
}
}
}
return result;
}
注意:以上代码仅为示例,实际使用时需要根据具体情况进行修改和优化。
相关推荐
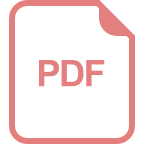
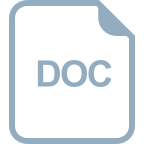
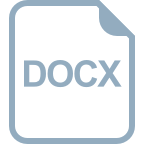













