用c语言写一个贪吃蛇游戏
时间: 2023-06-13 21:03:20 浏览: 55
好的,以下是一个简单的贪吃蛇游戏的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#define UP 72
#define DOWN 80
#define LEFT 75
#define RIGHT 77
int length = 1;
int bend_no;
int len;
char key;
void record();
void load();
int life;
void Delay(long double);
void Move();
int Score();
void Print();
void gotoxy(int x, int y);
void Bend();
void Boarder();
struct coordinate {
int x;
int y;
int direction;
};
typedef struct coordinate coordinate;
coordinate head, bend[500], food, body[30];
int main() {
char key;
Print();
system("cls");
load();
length = 1;
head.x = 25;
head.y = 20;
head.direction = RIGHT;
Boarder();
food.x = 30;
food.y = 20;
life = 3;
bend[0] = head;
Move();
return 0;
}
void Move() {
int a, i;
do {
foodrand:
food.x = rand() % 70;
if (food.x <= 10) {
food.x += 11;
}
food.y = rand() % 30;
if (food.y <= 10) {
food.y += 11;
}
} while (food.x == head.x && food.y == head.y);
system("cls");
Boarder();
if (head.direction == RIGHT) {
gotoxy(head.x, head.y);
printf(">");
} else if (head.direction == LEFT) {
gotoxy(head.x, head.y);
printf("<");
} else if (head.direction == UP) {
gotoxy(head.x, head.y);
printf("^");
} else if (head.direction == DOWN) {
gotoxy(head.x, head.y);
printf("v");
}
for (i = 0; i < length; i++) {
body[i].x = bend[i].x;
body[i].y = bend[i].y;
}
Bend();
gotoxy(food.x, food.y);
printf("*");
if (head.x == food.x && head.y == food.y) {
length++;
life++;
record();
goto foodrand;
}
for (i = 0; i < bend_no; i++) {
gotoxy(bend[i].x, bend[i].y);
printf("O");
}
for (i = 0; i < length; i++) {
gotoxy(body[i].x, body[i].y);
printf("*");
}
Delay(length);
coordinate prev = head;
gotoxy(head.x, head.y);
printf(" ");
if (head.direction == RIGHT) {
head.x++;
} else if (head.direction == LEFT) {
head.x--;
} else if (head.direction == UP) {
head.y--;
} else if (head.direction == DOWN) {
head.y++;
}
if (head.x >= 70 || head.x <= 10 || head.y >= 30 || head.y <= 10) {
life--;
if (life >= 0) {
head = prev;
Bend();
} else {
system("cls");
printf("\n\n\n\n\n\n\n\n\n\t\t\t\tGame Over!\n\n\n\n\n\n\n\n\n");
printf("\t\t\t\tPress any key to quit the game\n\n\n\n\n\n\n\n\n\n");
exit(0);
}
}
}
void Delay(long double k) {
long double i;
for (i = 0; i <= 10000000; i++) {
}
}
void Bend() {
int i, j, diff;
for (i = bend_no; i >= 0 && length < 5; i--) {
if (bend[i].x == bend[i - 1].x) {
diff = bend[i].y - bend[i - 1].y;
if (diff < 0) {
for (j = bend[i].y; j <= bend[i - 1].y; j++) {
bend[len].x = bend[i].x;
bend[len].y = j;
len++;
if (len == length) {
break;
}
}
} else {
for (j = bend[i].y; j >= bend[i - 1].y; j--) {
bend[len].x = bend[i].x;
bend[len].y = j;
len++;
if (len == length) {
break;
}
}
}
} else if (bend[i].y == bend[i - 1].y) {
diff = bend[i].x - bend[i - 1].x;
if (diff < 0) {
for (j = bend[i].x; j <= bend[i - 1].x; j++) {
bend[len].x = j;
bend[len].y = bend[i].y;
len++;
if (len == length) {
break;
}
}
} else {
for (j = bend[i].x; j >= bend[i - 1].x; j--) {
bend[len].x = j;
bend[len].y = bend[i].y;
len++;
if (len == length) {
break;
}
}
}
}
}
}
void Print() {
gotoxy(10, 12);
printf("\t\t\t\t--------------------------");
gotoxy(10, 30);
printf("\t\t\t\t--------------------------");
gotoxy(20, 14);
printf("WELCOME TO THE GAME 'SNAKE'");
gotoxy(20, 16);
printf("Use arrow keys to control the snake");
gotoxy(20, 18);
printf("Eat the food to increase the length");
gotoxy(20, 20);
printf("Press any key to start the game");
gotoxy(10, 40);
printf("\t\t\t\t--------------------------");
gotoxy(10, 50);
printf("\t\t\t\t--------------------------");
getch();
}
void load() {
int row, col, r, c;
gotoxy(10, 12);
printf("Loading...");
gotoxy(10, 14);
for (r = 1; r <= 20; r++) {
for (c = 1; c <= 10000000; c++) {
}
printf("%c", 177);
}
printf("\n");
}
int Score() {
int score;
score = length - 1;
return score;
}
void record() {
int sc = Score();
char plname[20], nplname[20], cha, c;
FILE* f;
f = fopen("score.txt", "a+");
if (f == 0) {
f = fopen("score.txt", "w+");
system("cls");
printf("Please wait while we configure your system files");
printf("\nPress any key to continue");
getch();
}
system("cls");
gotoxy(10, 12);
printf("Enter your name");
gotoxy(10, 14);
scanf("%s", plname);
fprintf(f, "Name: %s\nScore: %d\n", plname, sc);
gotoxy(10, 15);
printf("Do you want to see past records? (y/n)");
cha = getch();
if (cha == 'y') {
system("cls");
rewind(f);
printf("\t\tSCORES\n");
while (fscanf(f, "\n%s\n%d\n", nplname, &score) != EOF) {
printf("\n%s\n%d", nplname, score);
}
getch();
}
fclose(f);
}
void Boarder() {
system("cls");
int i;
gotoxy(food.x, food.y);
printf("*");
gotoxy(10, 10);
printf("SCORE: %d", Score());
gotoxy(70, 10);
printf("Life: %d", life);
for (i = 10; i < 71; i++) {
gotoxy(i, 10);
printf("-");
gotoxy(i, 30);
printf("-");
}
for (i = 10; i < 31; i++) {
gotoxy(10, i);
printf("|");
gotoxy(70, i);
printf("|");
}
}
void gotoxy(int x, int y) {
COORD coord;
coord.X = x;
coord.Y = y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), coord);
}
```
这是一个基于控制台的简单贪吃蛇游戏,游戏窗口大小为80x40,可以通过修改代码自定义窗口大小和其他游戏参数。
相关推荐
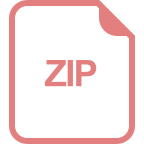
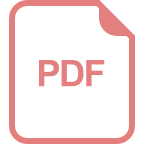


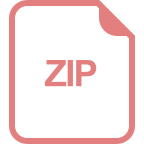
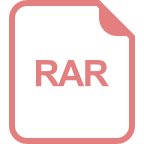
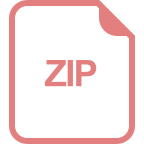
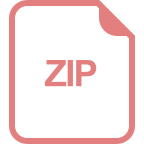
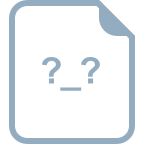