你需要将一系列的学生期末考试信息进行存储(学生人数不会超过100)。每个学生的信息包括: 姓名(由 first name 和last name 两部分组成,例如Jingyu LI,first_name = "Jingyu" last_name = "LI") ; 学号(12 位数字组成,开头4位为2022、2021、2020); C语言成绩(一个大于等于零的整数); 重修信息(学号 2022.........为否,其余为是); GPA等级(A+, A, B+, B, C+, C, D, F )。 A+: 100-93; A: 92-85; B+: 84-80; B: 79-75; C+:74-70; C: 69-65; D: 64-60; F: <60. 其中,姓名,学号,成绩为输入数据,其余数据需要你计算。 另外,学号不符合规定的数据需要删除掉。 输入 一系列 名、姓、学号、成绩 输出 名、姓、学号、成绩、重修信息、GPA等级 用c语言
时间: 2024-03-08 21:47:23 浏览: 83
好的,我可以帮你设计一个结构体来存储每个学生的信息,然后再对输入的数据进行处理计算,最后输出每个学生的完整信息。以下是代码实现:
```c
#include <stdio.h>
#include <string.h>
// 定义结构体来存储学生信息
struct Student {
char first_name[20];
char last_name[20];
long long student_id;
int score;
int is_repeat;
char grade[3];
};
// 计算GPA等级
void calculate_grade(struct Student *s) {
int score = s->score;
if (score >= 93) {
strcpy(s->grade, "A+");
} else if (score >= 85) {
strcpy(s->grade, "A");
} else if (score >= 80) {
strcpy(s->grade, "B+");
} else if (score >= 75) {
strcpy(s->grade, "B");
} else if (score >= 70) {
strcpy(s->grade, "C+");
} else if (score >= 65) {
strcpy(s->grade, "C");
} else if (score >= 60) {
strcpy(s->grade, "D");
} else {
strcpy(s->grade, "F");
}
}
int main() {
struct Student students[100];
int count = 0;
// 输入学生信息
while (1) {
char first_name[20], last_name[20];
long long student_id;
int score;
scanf("%s %s %lld %d", first_name, last_name, &student_id, &score);
// 判断学号是否符合规定,如果不符合则删除该数据
if (student_id < 202000000000 || student_id >= 202300000000) {
continue;
}
// 将输入的数据存储到结构体中
struct Student s;
strcpy(s.first_name, first_name);
strcpy(s.last_name, last_name);
s.student_id = student_id;
s.score = score;
s.is_repeat = (student_id >= 202200000000) ? 0 : 1;
calculate_grade(&s);
// 将结构体存储到数组中
students[count++] = s;
// 如果输入的数据已经达到100个,则退出输入
if (count == 100) {
break;
}
}
// 输出学生信息
for (int i = 0; i < count; i++) {
struct Student s = students[i];
printf("%s %s %lld %d %s %s\n", s.first_name, s.last_name, s.student_id, s.score, s.is_repeat ? "Yes" : "No", s.grade);
}
return 0;
}
```
这个程序可以循环输入一系列学生信息,直到输入的数据达到100个或者输入结束。对于每个输入的学生信息,程序会判断其学号是否符合规定,如果不符合则删除该数据,否则将其存储到结构体中,计算出重修信息和GPA等级,并将结构体存储到数组中。最后程序会输出每个学生的完整信息,包括姓名、学号、成绩、重修信息和GPA等级。
相关推荐
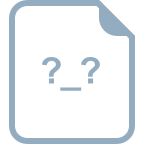
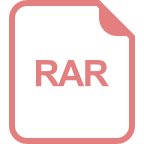
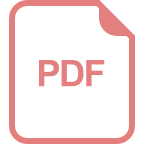







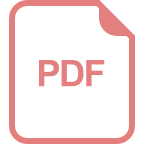
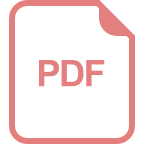
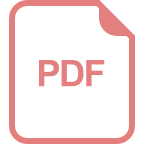
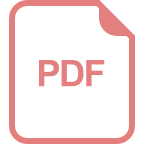
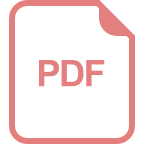
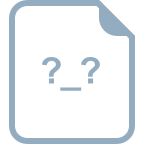
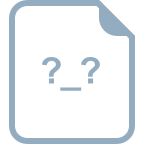