用js语言实现在单链表的某个节点之前或节点之后插入一个新节点。或者在单链表的尾部插入一个节点或单链表的头部插入一个节点。
时间: 2024-03-20 16:41:29 浏览: 23
好的,以下是使用 JavaScript 实现在单链表的某个节点之前或节点之后插入一个新节点,或者在单链表的尾部插入一个节点或单链表的头部插入一个节点的代码示例:
```javascript
class ListNode {
constructor(val, next = null) {
this.val = val;
this.next = next;
}
}
function insertBefore(head, insertVal) {
const node = new ListNode(insertVal);
if (!head) {
node.next = node;
return node;
}
let cur = head;
while (cur.next !== head && (cur.next.val < insertVal || cur.val >= insertVal)) {
cur = cur.next;
}
node.next = cur.next;
cur.next = node;
return head;
}
function insertAfter(head, insertVal) {
const node = new ListNode(insertVal);
if (!head) {
node.next = node;
return node;
}
let cur = head;
while (cur.next !== head && (cur.next.val <= insertVal || cur.val > insertVal)) {
cur = cur.next;
}
node.next = cur.next;
cur.next = node;
if (insertVal < head.val) {
return node;
}
return head;
}
function insertAtTail(head, insertVal) {
const node = new ListNode(insertVal);
if (!head) {
node.next = node;
return node;
}
let cur = head;
while (cur.next !== head) {
cur = cur.next;
}
node.next = cur.next;
cur.next = node;
return head;
}
function insertAtHead(head, insertVal) {
const node = new ListNode(insertVal);
if (!head) {
node.next = node;
return node;
}
let cur = head;
while (cur.next !== head) {
cur = cur.next;
}
node.next = head;
cur.next = node;
return node;
}
let head = new ListNode(1);
head.next = head;
head = insertBefore(head, 3);
head = insertBefore(head, 2);
head = insertAfter(head, 4);
head = insertAfter(head, 5);
head = insertAtTail(head, 6);
head = insertAtHead(head, 0);
let cur = head;
do {
console.log(cur.val);
cur = cur.next;
} while (cur !== head);
```
在这个示例中,我们定义了一个 ListNode 类来表示单链表中的节点。每个节点由一个整数值和指向下一个节点的指针组成。当单链表为空时,头指针指向空指针。
在插入节点时,我们需要遍历单链表,找到插入位置。具体而言,对于插入到某节点之前或之后,我们需要找到前一个节点,然后将新节点插入到前一个节点的 next 指针指向的位置;对于插入到尾部或头部,我们需要找到单链表的尾节点,然后在尾节点的 next 指针指向的位置插入新节点。
希望这个回答能对您有所帮助!
相关推荐
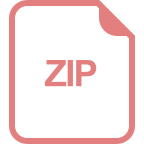
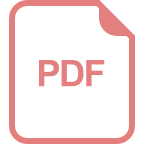
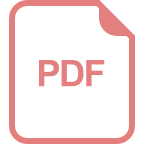
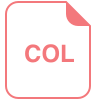
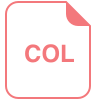
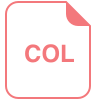
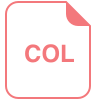
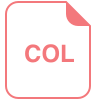









