灰狼算法matlab代码
时间: 2023-08-01 18:14:29 浏览: 33
以下是一个基于MATLAB的灰狼算法实现示例:
```matlab
function [best_solution, best_fitness] = grey_wolf_optimizer(func, dim, lb, ub, max_iter, pop_size)
% Grey Wolf Optimizer
% Inputs:
% func: function to be optimized
% dim: dimension of the problem
% lb: lower bound of the search space
% ub: upper bound of the search space
% max_iter: maximum number of iterations
% pop_size: population size
% Outputs:
% best_solution: best solution found
% best_fitness: best fitness value found
% Initialize the positions of the grey wolves
positions = repmat(lb, pop_size, 1) + rand(pop_size, dim) .* (repmat(ub, pop_size, 1) - repmat(lb, pop_size, 1));
% Initialize the fitness values of the grey wolves
fitness_values = feval(func, positions);
% Initialize the alpha, beta, and delta positions and fitness values
[~, alpha_index] = min(fitness_values);
alpha_position = positions(alpha_index, :);
alpha_fitness = fitness_values(alpha_index);
[~, beta_index] = min(fitness_values([1:alpha_index-1, alpha_index+1:end]));
beta_position = positions(beta_index, :);
beta_fitness = fitness_values(beta_index);
[~, delta_index] = max(fitness_values);
delta_position = positions(delta_index, :);
delta_fitness = fitness_values(delta_index);
% Initialize the convergence curve
convergence_curve = inf(max_iter, 1);
% Main loop
for iter = 1:max_iter
% Update the positions of the grey wolves
a = 2 - iter * (2 / max_iter); % parameter a decreases linearly from 2 to 0
r1 = rand(pop_size, dim);
r2 = rand(pop_size, dim);
A1 = 2 * a .* r1 - a;
C1 = 2 .* r2;
D_alpha = abs(C1 .* alpha_position - positions);
X1 = alpha_position - A1 .* D_alpha;
r1 = rand(pop_size, dim);
r2 = rand(pop_size, dim);
A2 = 2 * a .* r1 - a;
C2 = 2 .* r2;
D_beta = abs(C2 .* beta_position - positions);
X2 = beta_position - A2 .* D_beta;
r1 = rand(pop_size, dim);
r2 = rand(pop_size, dim);
A3 = 2 * a .* r1 - a;
C3 = 2 .* r2;
D_delta = abs(C3 .* delta_position - positions);
X3 = delta_position - A3 .* D_delta;
new_positions = (X1 + X2 + X3) / 3;
% Apply boundary conditions
new_positions = max(new_positions, repmat(lb, pop_size, 1));
new_positions = min(new_positions, repmat(ub, pop_size, 1));
% Evaluate the new positions
new_fitness_values = feval(func, new_positions);
% Update the alpha, beta, and delta positions and fitness values
if min(new_fitness_values) < alpha_fitness
[~, alpha_index] = min(new_fitness_values);
alpha_position = new_positions(alpha_index, :);
alpha_fitness = new_fitness_values(alpha_index);
end
if min(new_fitness_values([1:alpha_index-1, alpha_index+1:end])) < beta_fitness
[~, beta_index] = min(new_fitness_values([1:alpha_index-1, alpha_index+1:end]));
beta_position = new_positions(beta_index, :);
beta_fitness = new_fitness_values(beta_index);
end
if max(new_fitness_values) > delta_fitness
[~, delta_index] = max(new_fitness_values);
delta_position = new_positions(delta_index, :);
delta_fitness = new_fitness_values(delta_index);
end
% Update the convergence curve
convergence_curve(iter) = alpha_fitness;
end
% Output the results
best_solution = alpha_position;
best_fitness = alpha_fitness;
% Plot the convergence curve
semilogy(convergence_curve);
xlabel('Iterations');
ylabel('Best Cost');
grid on;
end
```
这个函数接受以下参数:
- `func`: 要进行优化的函数的句柄
- `dim`: 问题的维度
- `lb`: 搜索空间的下限
- `ub`: 搜索空间的上限
- `max_iter`: 最大迭代次数
- `pop_size`: 群体大小
它返回找到的最佳解和相应的最佳适应度值,并绘制收敛曲线。
相关推荐
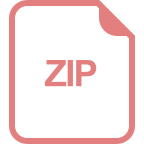














