springboot 单元测试 指定启动类
时间: 2023-08-21 13:14:41 浏览: 167
在 Spring Boot 单元测试中指定启动类可以使用 `@SpringBootTest` 注解的 `classes` 属性,例如:
```java
@SpringBootTest(classes = Application.class)
public class MyTest {
// ...
}
```
其中,`Application.class` 是指你的 Spring Boot 应用程序的启动类。这样指定后,在测试中就可以使用 Spring Boot 的自动配置和依赖注入等特性。
相关问题
springBoot单元测试类启动时指定不加载某个配置文件
Spring Boot 的单元测试通常使用 `@SpringBootTest` 或 `@ContextConfiguration` 注解来初始化 Spring 应用上下文,以便测试。如果你想要在单元测试中跳过特定的配置文件,你可以通过设置 `spring.config.location` 系统属性或在 `@ContextConfiguration` 中排除特定的资源配置。
1. 使用系统属性:
在测试类上添加 `@RunWith(SpringRunner.class)` 并在 `@Test` 方法前使用静态导入:
```java
@RunWith(SpringRunner.class)
@SpringBootTest
@ActiveProfiles("test") // 如果有多个 profile,可以选择其中一个
public class YourTestClass {
static {
System.setProperty("spring.config.location", "classpath:/your-test-config.properties");
}
//...
```
这里假设你有一个名为 `your-test-config.properties` 的文件,它不会被默认加载。
2. 使用 `@ContextConfiguration` 和 `excludeLocations`:
```java
@SpringBootTest(excludeLocations = {"classpath:/your-production-config.yml"}) // 避免加载 production 配置
@ContextConfiguration(locations = {"/your-test-config.yml"})
public class YourTestClass {
//...
```
这样只会加载 `your-test-config.yml` 文件。
注意:如果配置文件是基于 profiles 的,记得在测试时选择正确的 profile,如上面示例中的 `@ActiveProfiles("test")`。
springboot单元测试Apollo
### 如何在Spring Boot中使用Apollo进行单元测试
#### 使用`@SpringBootTest`注解启动应用上下文并加载Apollo配置
为了确保在执行单元测试时能够正确加载来自Apollo的配置,在编写测试类时可以利用`@SpringBootTest`注解来指定要使用的主应用程序类。这会触发整个Spring Boot应用上下文的初始化过程,从而使得可以从Apollo获取最新的配置数据。
```java
@SpringBootTest(classes = MySpringbootApplication.class, webEnvironment = WebEnvironment.RANDOM_PORT)
public class ApplicationTests {
// 测试逻辑...
}
```
此设置允许测试环境下的应用实例连接到Apollo服务器,并读取相应的配置文件[^3]。
#### 配置Apollo客户端以适应测试需求
为了让Apollo能够在测试期间正常工作,通常需要调整一些默认行为以便更好地模拟生产环境中的情况。可以通过自定义属性或覆盖现有属性的方式实现这一点:
- 设置Meta Server地址指向本地运行的服务端;
- 修改AppId为特定于测试用途的名字;
- 如果适用的话,还可以设定不同的Cluster名称用于区分不同类型的测试场景;
这些更改可以在项目的`application-test.yml`(或其他格式)中完成,该文件仅当激活了对应的profile(`test`)才会被加载。
```yaml
app:
id: "your-app-id-for-tests"
apollo:
meta: http://localhost:8080 # 假设这是本地部署的meta server URL
spring:
profiles:
active: test
```
上述YAML片段展示了如何针对测试目的定制Apollo的行为方式[^4]。
#### 编写基于JUnit/Jupiter框架的具体测试案例
一旦完成了前面提到的基础准备工作之后,就可以像平常一样创建具体的测试方法了。下面给出了一段简单的例子用来验证某个依赖注入组件是否按照预期接收到由Apollo提供的参数值。
```java
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
class SomeServiceTest {
@Autowired
private SomeService someService;
@Test
void shouldReturnExpectedValueFromApolloConfig() throws Exception {
String expectedValue = "value-from-apollo";
assertEquals(expectedValue, someService.getConfiguredProperty());
}
}
```
这段代码假设`SomeService`内部有一个名为`getConfiguredProperty()`的方法返回了一个字符串形式的应用程序配置项,而这个配置项是由Apollo动态下发给各个节点的[^1]。
阅读全文
相关推荐
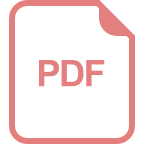
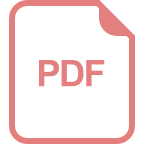
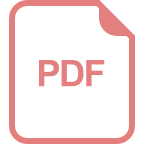












