学生信息管理系统c++
时间: 2023-11-03 18:53:26 浏览: 63
学生信息管理系统是一个常见的C++项目,主要功能是存储和管理学生的个人信息和成绩信息。以下是一个简单的学生信息管理系统C++代码示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 定义学生类
class Student {
public:
string name; // 姓名
int age; // 年龄
string gender; // 性别
int mathScore; // 数学成绩
int englishScore; // 英语成绩
int computerScore; // 计算机成绩
// 构造函数
Student(string n, int a, string g, int m, int e, int c) {
name = n;
age = a;
gender = g;
mathScore = m;
englishScore = e;
computerScore = c;
}
// 显示学生信息
void display() {
cout << "姓名:" << name << endl;
cout << "年龄:" << age << endl;
cout << "性别:" << gender << endl;
cout << "数学成绩:" << mathScore << endl;
cout << "英语成绩:" << englishScore << endl;
cout << "计算机成绩:" << computerScore << endl;
}
// 计算平均成绩
float averageScore() {
return (mathScore + englishScore + computerScore) / 3.0;
}
};
// 主函数
int main() {
// 创建学生对象
Student stu("张三", 18, "男", 90, 80, 85);
// 显示学生信息
stu.display();
// 显示平均成绩
cout << "平均成绩:" << stu.averageScore() << endl;
return 0;
}
```
该程序定义了一个名为 `Student` 的类,包含了学生的基本信息和成绩信息,并且定义了构造函数、显示学生信息和计算平均成绩的方法。在主函数中创建了一个学生对象,并且调用了上述方法。
相关推荐
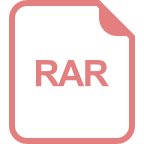
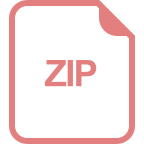

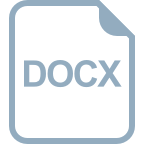
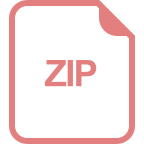
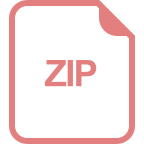
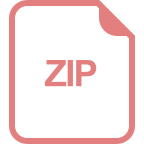
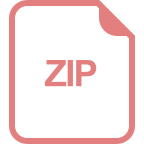
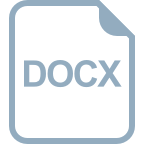
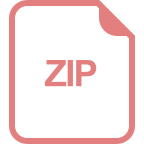
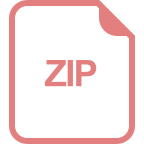
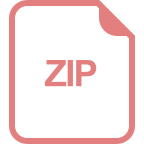