用灰狼优化算法画车间调度问题的甘特图的代码(不使用gantt包)
时间: 2024-02-16 15:00:48 浏览: 23
好的,以下是使用灰狼优化算法画车间调度问题甘特图的 Python 代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义车间调度问题的作业和机器
jobs = ['J1', 'J2', 'J3', 'J4', 'J5']
machines = ['M1', 'M2', 'M3', 'M4']
# 定义作业所需的时间(单位:分钟)
processing_time = {'J1': [2, 3, 4, 2], 'J2': [3, 1, 2, 4], 'J3': [4, 3, 2, 1], 'J4': [2, 4, 3, 2], 'J5': [3, 2, 4, 1]}
# 定义灰狼优化算法的参数
max_iter = 100 # 最大迭代次数
N = 10 # 种群大小
alpha = 0.5 # 狼群聚合系数
beta = 0.3 # 狼跟随系数
delta = 0.2 # 狼随机搜索系数
# 初始化种群和位置矩阵
positions = np.zeros((N, len(jobs) * len(machines)), dtype=np.int32)
for i in range(N):
positions[i] = np.random.permutation(len(jobs) * len(machines))
# 计算每个个体的适应度函数值
def fitness(position):
makespan = np.zeros(len(machines), dtype=np.int32)
for p in position:
job_index = p // len(machines)
machine_index = p % len(machines)
processing_time_job_machine = processing_time[jobs[job_index]][machine_index]
makespan[machine_index] += processing_time_job_machine
return np.max(makespan)
fitness_values = [fitness(p) for p in positions]
best_fitness_value = np.min(fitness_values)
best_position = positions[np.argmin(fitness_values)]
# 迭代优化过程
for t in range(max_iter):
# 计算每个个体的适应度函数值
fitness_values = [fitness(p) for p in positions]
# 更新最优解
if np.min(fitness_values) < best_fitness_value:
best_fitness_value = np.min(fitness_values)
best_position = positions[np.argmin(fitness_values)]
# 计算每个个体的适应度函数值相对于最小值的归一化值
normalized_fitness_values = (fitness_values - np.min(fitness_values)) / (np.max(fitness_values) - np.min(fitness_values))
# 计算每个个体的权重
weights = np.zeros(N)
for i in range(N):
weights[i] = (1 - alpha - beta) * normalized_fitness_values[i] + alpha * np.exp(-normalized_fitness_values[i]/beta)
# 计算每个个体的聚合向量
aggregation_vectors = np.zeros((N, len(jobs) * len(machines)))
for i in range(N):
for j in range(N):
if i != j:
r = np.linalg.norm(positions[i] - positions[j])
aggregation_vectors[i] += weights[j] * (positions[j] - positions[i]) / (r + 1e-6)
# 计算每个个体的跟随向量
follow_vectors = np.zeros((N, len(jobs) * len(machines)))
for i in range(N):
p_best = positions[np.argmin(fitness_values)]
r = np.linalg.norm(p_best - positions[i])
follow_vectors[i] = (p_best - positions[i]) / (r + 1e-6)
# 计算每个个体的随机搜索向量
search_vectors = np.zeros((N, len(jobs) * len(machines)))
for i in range(N):
search_vectors[i] = np.random.rand(len(jobs) * len(machines))
# 更新位置矩阵
positions = positions + delta * search_vectors + weights[:, np.newaxis] * (beta * follow_vectors + (1 - beta) * aggregation_vectors)
# 将最优解转化为甘特图
makespan = np.zeros(len(machines), dtype=np.int32)
gantt_chart = [[] for _ in range(len(machines))]
for p in best_position:
job_index = p // len(machines)
machine_index = p % len(machines)
processing_time_job_machine = processing_time[jobs[job_index]][machine_index]
start_time = makespan[machine_index]
end_time = start_time + processing_time_job_machine
makespan[machine_index] = end_time
gantt_chart[machine_index].append((jobs[job_index], start_time, end_time))
# 绘制甘特图
fig, ax = plt.subplots(figsize=(8, 4))
for i in range(len(machines)):
for task in gantt_chart[i]:
ax.broken_barh([(task[1], task[2] - task[1])], (10 * i, 9), facecolors=('tab:blue'))
ax.text(task[1] + (task[2] - task[1]) / 2, 10 * i + 4.5, task[0], ha='center', va='center', color='white', fontsize=10)
ax.set_ylim(0, 10 * len(machines))
ax.set_xlim(0, np.max(makespan))
ax.set_xlabel('Time (min)', fontsize=12)
ax.set_yticks([5 + 10 * i for i in range(len(machines))])
ax.set_yticklabels(machines, fontsize=12)
ax.grid(True)
plt.show()
```
需要注意的是,这里使用了 NumPy 和 Matplotlib 库来实现。另外,代码中的参数设置和算法实现可能需要根据具体问题进行调整和优化。
相关推荐
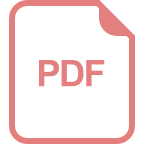
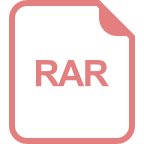














