ThreadPoolExecutor 的使用
时间: 2024-12-27 18:13:47 浏览: 6
### 使用 `ThreadPoolExecutor` 的方法及示例
#### 创建线程池并提交任务
为了使用 `ThreadPoolExecutor` 实现并发执行任务,可以通过指定最大工作线程数来创建一个实例。通常情况下,在上下文管理器 (`with` 语句) 中使用该对象能够确保资源被正确释放。
```python
from concurrent.futures import ThreadPoolExecutor
def task_function(param):
# 执行具体业务逻辑的任务函数
result = param * 2
return f"Processed {param} -> Result is {result}"
# 定义要处理的数据列表
data_points = [1, 2, 3, 4]
# 使用 with 语句初始化具有固定数量工作者的最大线程池
with ThreadPoolExecutor(max_workers=4) as executor:
futures = []
for item in data_points:
future = executor.submit(task_function, item)
futures.append(future)
for fut in futures:
try:
print(fut.result()) # 获取已完成任务的结果
except Exception as exc:
print('Task generated an exception:', exc)[^1]
```
上述代码展示了如何定义一个简单的任务函数,并通过 `submit()` 方法向线程池提交多个独立的任务。之后遍历所有的 Future 对象以收集它们完成后的返回值[^2]。
#### 利用 map 函数批量分发任务
除了单独调用 `submit()`, 还可以直接采用更简洁的方式 —— 调用 `map()` 来一次性分配一系列参数给目标函数:
```python
from concurrent.futures import ThreadPoolExecutor
def square_number(x):
"""计算平方"""
return x*x
numbers_to_process = list(range(5))
with ThreadPoolExecutor(max_workers=8) as executors_pool:
squared_numbers = list(executors_pool.map(square_number, numbers_to_process))
print(squared_numbers)
```
这段例子说明了当有大量相似性质的小型任务时,可以借助于 `map()` 方便快捷地将其映射到不同的线程上去执行,最终得到有序的结果集合[^3]。
阅读全文
相关推荐
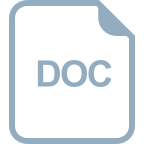
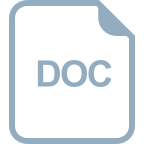
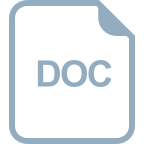













