web练习使用jjwt和Spring Security构建安全的API,完成校园网项目的认证和鉴权容易遇到的问题
时间: 2024-03-03 22:52:27 浏览: 23
在使用jjwt和Spring Security构建安全的API时,校园网项目的认证和鉴权可能会遇到以下问题:
1. 一些敏感信息泄露的问题:在使用jjwt和Spring Security时,要注意保护用户的敏感信息。例如,用户的密码、邮箱地址等信息应该进行加密存储,以免出现泄露的情况。
2. token过期问题:在使用jjwt时,token的过期时间应该设置得合理,以保证token不会过期太快,也不会过期太慢。如果token过期了,用户就需要重新登录,这会影响用户的体验。
3. 恶意攻击问题:在使用jjwt和Spring Security时,要注意防范恶意攻击。例如,使用CSRF令牌来防止跨站点请求伪造攻击(CSRF攻击);禁止使用明文传输密码等敏感信息。
4. 权限控制问题:在使用Spring Security时,要注意权限控制。例如,对于一些需要特定权限才能访问的API接口,要对用户的权限进行验证,以保证用户只能访问他们有权限访问的API接口。
5. 错误处理问题:在使用jjwt和Spring Security时,要注意错误处理。例如,如果用户提供的token无效,需要给出相应的错误提示。
总之,在使用jjwt和Spring Security构建安全的API时,要注意安全问题,并采取相应措施来避免出现安全漏洞。
相关问题
Spring Cloud Gateway实现统一认证和鉴权
Spring Cloud Gateway是一个基于Spring Boot 2.x的API网关,可以作为微服务架构中的统一入口,提供路由、转发、负载均衡、限流、降级、统一认证和鉴权等功能。在实现统一认证和鉴权时,可以结合Spring Security和JWT来实现。
具体实现步骤如下:
1. 引入Spring Security和JWT的依赖
在Spring Cloud Gateway的pom.xml文件中,引入Spring Security和JWT的依赖:
```
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-web</artifactId>
<version>${spring-security.version}</version>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-config</artifactId>
<version>${spring-security.version}</version>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>${jjwt.version}</version>
</dependency>
```
2. 配置Spring Security
在Spring Cloud Gateway的配置类中,配置Spring Security:
```
@Configuration
@EnableWebFluxSecurity
public class SecurityConfig {
@Autowired
private JwtAuthenticationManager jwtAuthenticationManager;
@Bean
public SecurityWebFilterChain securityWebFilterChain(ServerHttpSecurity http) {
return http.csrf().disable()
.authorizeExchange()
.pathMatchers("/login").permitAll()
.anyExchange().authenticated()
.and()
.addFilterAt(new JwtAuthenticationFilter(jwtAuthenticationManager), SecurityWebFiltersOrder.AUTHENTICATION)
.build();
}
}
```
在上面的配置中,首先禁用了CSRF防护,然后配置了登录接口不需要认证,其它接口都需要认证。最后添加了一个JWT认证过滤器。
3. 配置JWT
在Spring Cloud Gateway的配置类中,配置JWT:
```
@Configuration
public class JwtConfig {
@Value("${jwt.secret}")
private String secret;
@Value("${jwt.expiration}")
private Long expiration;
@Bean
public JwtAuthenticationManager jwtAuthenticationManager() {
return new JwtAuthenticationManager(secret);
}
@Bean
public JwtTokenGenerator jwtTokenGenerator() {
return new JwtTokenGenerator(secret, expiration);
}
}
```
在上面的配置中,配置了JWT的密钥和过期时间,并创建了JWT的管理器和生成器。
4. 实现登录接口
实现登录接口,生成JWT并返回给客户端:
```
@RestController
public class LoginController {
@Autowired
private JwtTokenGenerator jwtTokenGenerator;
@PostMapping("/login")
public Mono<ResponseEntity<Map<String, String>>> login(@RequestBody LoginRequest loginRequest) {
// 验证用户名和密码
if (validateUsernameAndPassword(loginRequest)) {
// 生成JWT
String token = jwtTokenGenerator.generateToken(loginRequest.getUsername());
// 返回JWT
Map<String, String> responseBody = new HashMap<>();
responseBody.put("token", token);
return Mono.just(ResponseEntity.ok(responseBody));
} else {
return Mono.just(ResponseEntity.status(HttpStatus.UNAUTHORIZED).build());
}
}
private boolean validateUsernameAndPassword(LoginRequest loginRequest) {
// 验证用户名和密码逻辑
}
}
```
在上面的代码中,先验证用户名和密码是否正确,如果正确则生成JWT并返回给客户端,否则返回401未授权状态码。
5. 实现JWT认证过滤器
实现JWT认证过滤器,从请求头中获取JWT并验证:
```
public class JwtAuthenticationFilter extends AuthenticationWebFilter {
public JwtAuthenticationFilter(JwtAuthenticationManager jwtAuthenticationManager) {
super(jwtAuthenticationManager);
}
@Override
protected Mono<Void> onAuthSuccess(Authentication authentication, ServerWebExchange exchange) {
return super.onAuthSuccess(authentication, exchange);
}
@Override
protected Mono<Void> onAuthFailure(AuthenticationException e, ServerWebExchange exchange) {
return super.onAuthFailure(e, exchange);
}
@Override
public Mono<Void> filter(ServerWebExchange exchange, AuthenticationFilterChain chain) {
String token = extractToken(exchange.getRequest().getHeaders().getFirst("Authorization"));
if (StringUtils.isEmpty(token)) {
return chain.filter(exchange);
} else {
JwtAuthenticationToken jwtAuthenticationToken = new JwtAuthenticationToken(token);
return super.filter(exchange, chain)
.subscriberContext(ReactiveSecurityContextHolder.withAuthentication(jwtAuthenticationToken));
}
}
private String extractToken(String header) {
// 从Authorization头中提取JWT
}
}
```
在上面的代码中,先从请求头中提取JWT,如果JWT为空则直接调用下一个过滤器,否则创建JwtAuthenticationToken并将其设置到SecurityContext中。
6. 实现JWT认证管理器
实现JWT认证管理器,验证JWT是否正确:
```
public class JwtAuthenticationManager implements ReactiveAuthenticationManager {
private final String secret;
public JwtAuthenticationManager(String secret) {
this.secret = secret;
}
@Override
public Mono<Authentication> authenticate(Authentication authentication) {
String token = authentication.getCredentials().toString();
try {
Jws<Claims> claimsJws = Jwts.parser().setSigningKey(secret).parseClaimsJws(token);
String username = claimsJws.getBody().getSubject();
return Mono.just(new JwtAuthenticationToken(username, token));
} catch (JwtException e) {
return Mono.error(e);
}
}
}
```
在上面的代码中,使用JWT解析器解析JWT,并验证签名和过期时间,如果验证通过则创建JwtAuthenticationToken。
7. 实现JWT认证令牌
实现JWT认证令牌:
```
public class JwtAuthenticationToken extends AbstractAuthenticationToken {
private final String token;
private final String username;
public JwtAuthenticationToken(String token) {
super(Collections.emptyList());
this.token = token;
this.username = null;
}
public JwtAuthenticationToken(String username, String token) {
super(Collections.emptyList());
this.token = token;
this.username = username;
setAuthenticated(true);
}
@Override
public Object getCredentials() {
return token;
}
@Override
public Object getPrincipal() {
return username;
}
}
```
在上面的代码中,实现了AbstractAuthenticationToken的两个抽象方法,并添加了一个token和username属性。
8. 配置路由规则
最后,配置路由规则,启用Spring Cloud Gateway:
```
@Configuration
public class GatewayConfig {
@Autowired
private JwtTokenGenerator jwtTokenGenerator;
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("login", r -> r.path("/login")
.uri("http://localhost:8080/login"))
.route("hello", r -> r.path("/hello")
.filters(f -> f.requestHeader("Authorization", "Bearer " + jwtTokenGenerator.generateToken("user")))
.uri("http://localhost:8081/hello"))
.build();
}
}
```
在上面的配置中,配置了两个路由规则,一个是登录接口,另一个是hello接口,hello接口需要通过JWT认证才能访问。
Spring Security和WT登录认证实现
下面是Spring Security和WT登录认证的实现步骤:
1. 配置Spring Security
在Spring Security的配置文件中,需要定义用户的身份认证方式,可以使用基于数据库、LDAP等多种方式。具体的配置可以参考Spring Security的官方文档。
2. 添加WT支持
可以使用第三方库,如jjwt,来实现WT的支持。在生成WT Token时,需要指定过期时间、用户信息等内容。
3. 实现登录接口
在登录接口中,需要使用Spring Security进行身份认证,验证用户名和密码是否正确。如果验证通过,可以生成WT Token,并将Token返回给前端。
4. 实现Token验证拦截器
在拦截器中,需要解析前端传递的Token,并验证Token的有效性。如果Token有效,则允许用户访问对应的资源。
具体实现的代码可以参考以下示例:
1. 配置Spring Security
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/login").permitAll()
.anyRequest().authenticated()
.and()
.addFilter(new JWTAuthenticationFilter(authenticationManager()))
.addFilter(new JWTAuthorizationFilter(authenticationManager()))
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.csrf().disable();
}
@Override
public void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(new BCryptPasswordEncoder());
}
}
```
2. 添加WT支持
```java
public class JWTUtils {
private static final String SECRET = "mySecret";
private static final long EXPIRATION_TIME = 864_000_000; // 10 days
private static final String TOKEN_PREFIX = "Bearer ";
private static final String HEADER_STRING = "Authorization";
public static String generateToken(Authentication auth) {
UserDetails user = (UserDetails) auth.getPrincipal();
Date expirationDate = new Date(System.currentTimeMillis() + EXPIRATION_TIME);
String token = Jwts.builder()
.setSubject(user.getUsername())
.setExpiration(expirationDate)
.signWith(SignatureAlgorithm.HS512, SECRET)
.compact();
return TOKEN_PREFIX + token;
}
public static Authentication verifyToken(HttpServletRequest request) {
String token = request.getHeader(HEADER_STRING);
if (token != null) {
String user = Jwts.parser()
.setSigningKey(SECRET)
.parseClaimsJws(token.replace(TOKEN_PREFIX, ""))
.getBody()
.getSubject();
if (user != null) {
return new UsernamePasswordAuthenticationToken(user, null, new ArrayList<>());
}
return null;
}
return null;
}
}
```
3. 实现登录接口
```java
@RestController
public class LoginController {
@Autowired
private AuthenticationManager authenticationManager;
@PostMapping("/login")
public ResponseEntity<?> login(@RequestBody User user) {
try {
Authentication auth = authenticationManager.authenticate(
new UsernamePasswordAuthenticationToken(user.getUsername(), user.getPassword()));
String token = JWTUtils.generateToken(auth);
return ResponseEntity.ok(new AuthResponse(token));
} catch (BadCredentialsException e) {
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).build();
}
}
}
```
4. 实现Token验证拦截器
```java
public class JWTAuthorizationFilter extends BasicAuthenticationFilter {
public JWTAuthorizationFilter(AuthenticationManager authenticationManager) {
super(authenticationManager);
}
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws IOException, ServletException {
Authentication auth = JWTUtils.verifyToken(request);
if (auth != null) {
SecurityContextHolder.getContext().setAuthentication(auth);
chain.doFilter(request, response);
} else {
response.setStatus(HttpServletResponse.SC_UNAUTHORIZED);
}
}
}
```
以上代码仅供参考,具体的实现方式可能因为业务需求和框架版本等因素而有所不同。
相关推荐
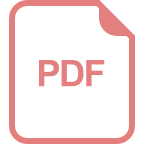












