写一个代码,输入出生年月输出年龄
时间: 2023-04-03 19:02:10 浏览: 56
您好,根据您输入的出生年月,我可以为您计算出您的年龄。以下是代码示例:
```c
#include <stdio.h>
#include <time.h>
int main()
{
int year, month, day;
printf("请输入您的出生年月(格式为YYYY-MM-DD):");
scanf("%d-%d-%d", &year, &month, &day);
time_t now = time(NULL);
struct tm *tm_now = localtime(&now);
int age = tm_now->tm_year + 190 - year;
if (tm_now->tm_mon + 1 < month || (tm_now->tm_mon + 1 == month && tm_now->tm_mday < day)) {
age--;
}
printf("您的年龄为:%d岁\n", age);
return ;
}
```
请注意,以上代码仅供参考,实际应用中可能需要考虑更多的边界情况。
相关问题
用phyton写一个求出生年月的代码
可以使用以下代码来求出生年月:
```python
import datetime
def calculate_age(birth_date):
today = datetime.date.today()
age = today.year - birth_date.year - ((today.month, today.day) < (birth_date.month, birth_date.day))
return age
birth_year = int(input("请输入出生年份:"))
birth_month = int(input("请输入出生月份:"))
birth_day = int(input("请输入出生日期:"))
birth_date = datetime.date(birth_year, birth_month, birth_day)
age = calculate_age(birth_date)
print("您的出生年月为:{}年{}月{}日".format(birth_year, birth_month, birth_day))
print("您现在的年龄为:{}岁".format(age))
```
这个代码可以让用户输入出生年月日,然后计算出用户的年龄,并输出出生年月和年龄。
用python帮我写一段代码,要求是输入身份证号码可以判断出性别年龄和出生年月日
当然可以!以下是一个示例代码,可以根据输入的身份证号码判断出性别、年龄和出生年月日:
```python
def analyze_id_card(id_card):
# 获取出生年月日
birth_year = id_card[6:10]
birth_month = id_card[10:12]
birth_day = id_card[12:14]
# 获取性别
gender = int(id_card[16:17])
if gender % 2 == 0:
gender = '女'
else:
gender = '男'
# 计算年龄
from datetime import datetime
current_year = datetime.now().year
age = current_year - int(birth_year)
return gender, age, birth_year, birth_month, birth_day
# 输入身份证号码
id_card_number = input("请输入身份证号码:")
# 分析身份证信息
gender, age, birth_year, birth_month, birth_day = analyze_id_card(id_card_number)
# 打印结果
print("性别:", gender)
print("年龄:", age)
print("出生年月日:", birth_year, "/", birth_month, "/", birth_day)
```
你可以在运行时输入身份证号码,然后代码会分析并输出性别、年龄和出生年月日信息。请记得输入正确的身份证号码格式。
相关推荐
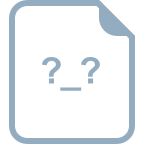












